โ how to make an API call
I have tried looking up tutorials but they ended up making me more confused. I want to take a string, generate an API call from that, then download the response.
The API takes a URL-encoded string, and returns a link to a wav file, which I'd like to download. Is that possible?
here's the link to the API I'd like to try using:
https://tts.cyzon.us/
https://github.com/calzoneman/aeiou/blob/master/docs/usage-guidelines.md
GitHub
aeiou/usage-guidelines.md at master ยท calzoneman/aeiou
node.js web interface to silly text to speech things - aeiou/usage-guidelines.md at master ยท calzoneman/aeiou
120 Replies
someone sent me this, which is what they personally use to interact with a different API. Could this potentially be adapted for my use?
I've tried understanding it but I can't...
Unknown Userโข2y ago
Message Not Public
Sign In & Join Server To View
if you know it downloads a wav file, why are you saving it as an mp3 lol
using on the first 2
afaik you shouldn't be
using
an HttpClient
you should
that's why it implements idisposable
so you can dispose it
anyway, that's how you should do it
the idea is to not create a new client every time if you do a lot of requests
so you create one static long running client for the whole app
Very bad idea to phrase it like this.
MS docs repeatedly show that you should not be
using HttpClient client = new()
, as that pattern is never the correct one for httpclients. You should have a static one, or use the factory.Unknown Userโข2y ago
Message Not Public
Sign In & Join Server To View
that's what someone sent me as their own version
not made for me
but for me to use as a base
Unknown Userโข2y ago
Message Not Public
Sign In & Join Server To View
huh? just declaring it like this works?
I thought you had to call a method or something
there are method calls in that snippet
Well you still call a method
I think the reason why they advice against is is because it is bad performance wise do constantly make a new HttpClient, and you should reuse the same client.
Because it does stuff in the background when you create one
But don't quote me on that
no, it has to do with connection pool exhaustion
its not performance related
the usage guideline they sent says it's just a get?
Unknown Userโข2y ago
Message Not Public
Sign In & Join Server To View
I have no idea what your words mean...
so... I set up the variables with the info I need
then call a method?
Unknown Userโข2y ago
Message Not Public
Sign In & Join Server To View
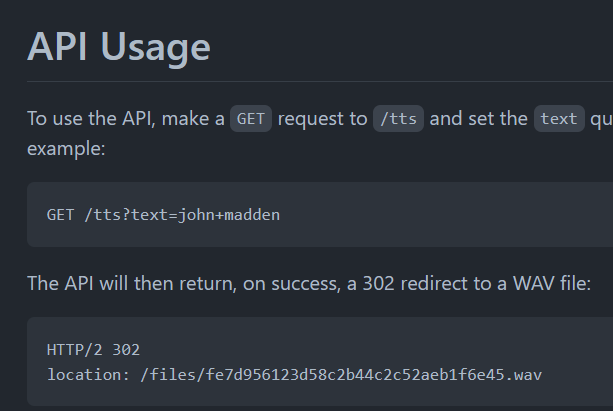
Unknown Userโข2y ago
Message Not Public
Sign In & Join Server To View
which is why I'm asking for help lol... this is my first time doing anything related to API
https://tts.cyzon.us/tts?text=hello+world
like this
well start out small, with a console app that just makes a request and shows the response
the link generates a wav file
then go from there
I'm integrating this into another app - the C# code is just a script
so idk how to even open a console
start out small
dont start with going for the end goal
learn the components first
uh huh?
so I make a standalone C# script first?
or how does that work
Unknown Userโข2y ago
Message Not Public
Sign In & Join Server To View
sure, a standalone console app.
I've only ever used C# as a scripting language so...
for unity and now for an app called StreamerBot
perhaps a good time to learn how to use it properly then?
Also for one, you cannot make a "standalone C# script"
uh... I wonder if I have the patience to learn it properly at this point tbh
I've been using C# for years now in unity
I mean, its not hard to make a blank console app
its... 3-4 button clicks in VS
I use notepad
stop using notepad
or like two console commands
how tf do you get anything done...?
it works fine
darkmode, autocomplete, that is sufficient for me
you'e been missing out if you've been using that for years
oh nah nah I use notepad for my Streamerbot scripting
since the in-app editor stinks
it doesn't even properly handle [tab] inputs
How fun is it to fix errors in Notepad?
I don't get any
I mean - the errors I get are very minor ones like typos
I use notepad to write the code then I copy paste it into the streamerbot editor
ah, write everything correctly the first time 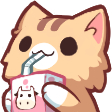
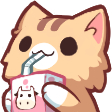
and that has a built-in compiler
hell naw
I make worse code than anyone I know
well you're only doing yourself a disservice by not using any kind of IDE, but you do you I guess
basically, C# is used as a scripting language with its own methods
but if you import other stuff you can do stuff normal C# can
like open new windows
I got someone else's script which opens an admin window
that's besides the point here - I'm just curious if I could make an API call, and if anyone would be able to help me learn the fundimentals
because online tutorials ain't making it clear to me
consider me baby at understanding this crap...
well, if you make a new blank hello world console app as step 1
and then make THAT make the request... then you should be able to tweak it so your bot or whatever can do it
591 lines left ๐
Have you checked $helloworld?
Written interactive course https://learn.microsoft.com/en-us/users/dotnet/collections/yz26f8y64n7k07
Videos https://dotnet.microsoft.com/learn/videos
uh
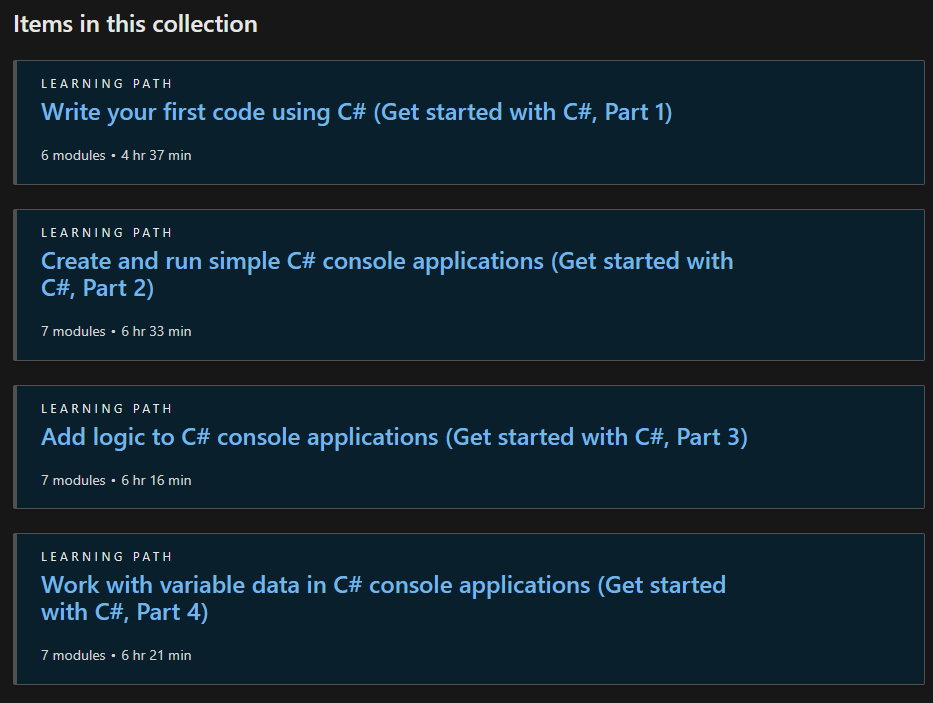
I don't think I have the time for this...
Thrn how do you expect to learn anything...?
That's the absolute basics though, but it's kind of unclear what exactly you're looking for
certainly feels like they want spoonfeeding
I mean...
my way of learning so far was to try and figure out things as I go
I was using arrays before I knew what a class was
still kinda don't know what it is
then go right ahead, you've been given all the information you need already
this?
you need to make a
HttpClient
instance and use the methods on that
thats the basicshttps://learn.microsoft.com/en-us/dotnet/api/system.net.http.httpclient?view=net-8.0
I'll try and base my code off this I guess
HttpClient Class (System.Net.Http)
Provides a class for sending HTTP requests and receiving HTTP responses from a resource identified by a URI.
(and also maybe look into
async
and await
a bit)I'll give it a shot and get back with my attempt
i mean we also have to consider what version of .net this streamerbot thing supports right
I have absolutely no idea
its gonna be .net 4.7 or later, so httpclient will still be the way to go
What are you even running your code through? You said it was some "Streamerbot" program?
yup
Do you have a link to this program/service?
Streamer.bot Wiki
Execute C# Code
usually the scripts I make are to clean up logic, because logic via the GUI sucks
so it's just a simple if/else or switch script of around 40 lines or so
then return true
this is my first time doing something this complex here
https://streamer.bot
this is the main app btw
Streamer.bot
Supercharge your Live Stream | Streamer.bot
Supercharge your live stream with Streamer.bot! The most powerful stream bot with Twitch and YouTube support.
sorry I linked the docs page
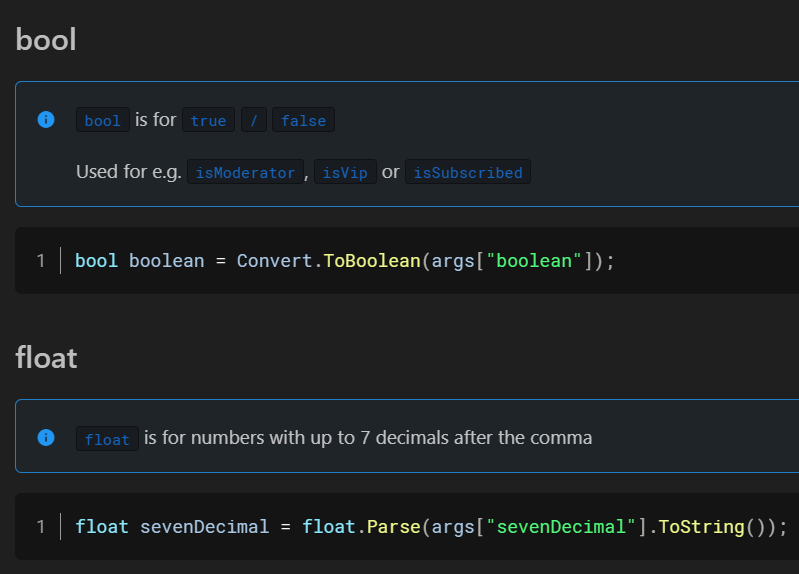
???
.Parse
in one example, Convert
in the otherthat page reads like it itself doesn't know what it's talking about
I am confused myself at how the variables get converted from SB to C# variables
but it's worked so far so...
no clue what it does, but it seems like an ambitious project by a single person?
What's SB?
so it's gonna be rough
Ah just StreamerBot
streamerbot lol
yea
yep
I can understand that the C# implementation is very wonky
but it's the most powerful thing that's available
The project unfortunately doesn't seem to be open-source
the GUI is super restrictive - if/else blocks can only either break out of the current action, or call another action
meaning in code you have if then else, but in the GUI variant you must have 3 different methods that it jumps between
so I always prefer code because it's cleaner
afaik it's closed-source
oof
man... I'll just pray the guy inside the SB help channel is able to come to my rescue
he's the one who made his own TTS via API system, and he's the one who shared the above code with me
that's what he uses and it works
Also since I assume this is for Twitch or something, you can make Twitch bots in C# without this thing
I guess... but then you'd have to re-write it for youtube
yeah ig :/
also I'd have no idea how to listen to the api calls and stuff that twitch fires out
and ofc they keep changing their API so keeping up with that is a pain, SB does that for me with app updates
this is what he told me about the API thing from streamerbot
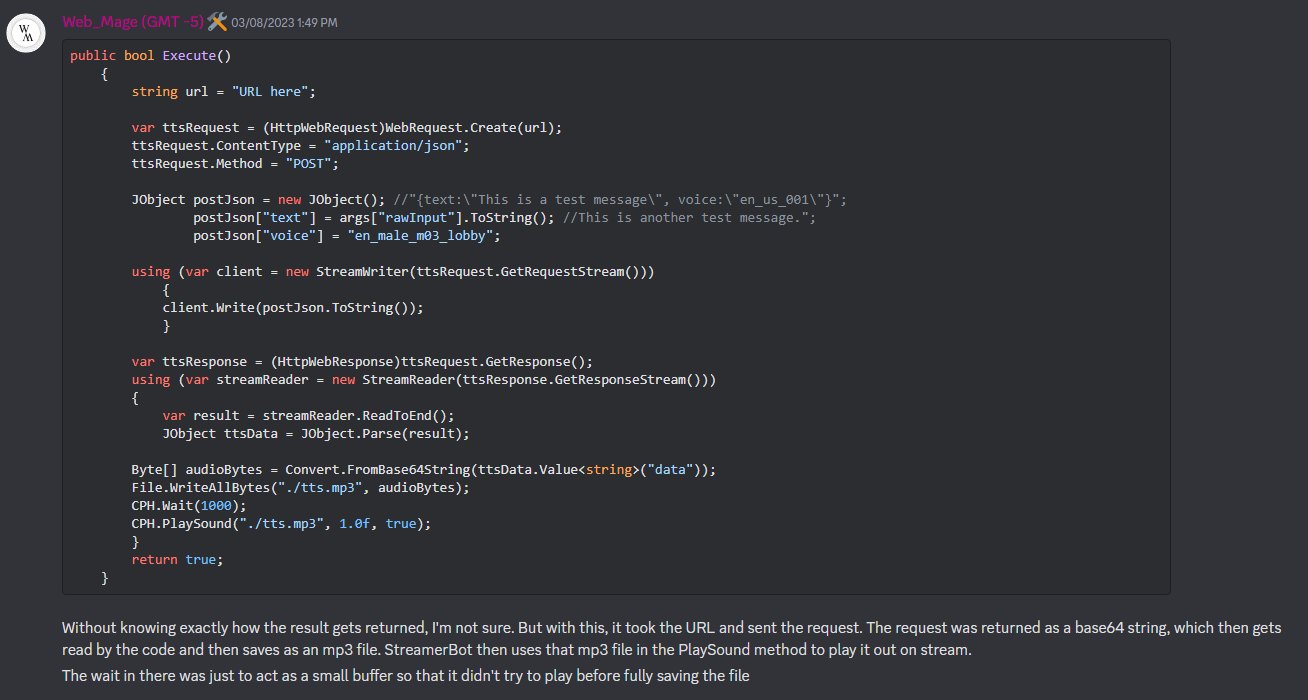
Execute
is a sync method. ouchoof
big oof
ambitious project developed by a single person with mediocre docs, bad practices, and supposedly not very good code
sounds about right
sounds about right
but it's the most powerful but still user-friendly thing I could find
going custom takes a lot of upkeep and knowhow, and is difficult to develop
yeah :/
I feel your pain
this is a part of a script I wrote myself for SB
it's... not a very good script but it works
Unknown Userโข2y ago
Message Not Public
Sign In & Join Server To View
sucks that this is closed source
that alone would make me not even consider using it
this app has a good amount of support
it's the most powerful tool streamers have at their disposal
(besides going custom of course)
but most of em do their stuff like this
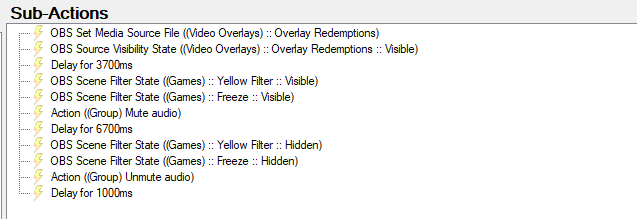
very few actually code in C#
so I guess that's why the implementation might be wonky... not enough people use it to warrant making it proper, but just keep it up-to-date and functional enough
wellp, someone from the SB discord helped me out and I got something that works
still don't know how it works though, I don't understand most of what was written here:
should just be
and the
Execute
method should be async Task<bool>
i don't think that's possible unfortunately
yep, as far as I can tell streamerbots API is entirely synchronous
Unknown Userโข2y ago
Message Not Public
Sign In & Join Server To View
from what I understand that's merging three into a single step, right?
and yes it has to be inside a bool
Unknown Userโข2y ago
Message Not Public
Sign In & Join Server To View
they were asking whether
GetAudioFile().GetAwaiter().GetResult()
was "merging three into a single step",yup
Unknown Userโข2y ago
Message Not Public
Sign In & Join Server To View
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.