❔ multiple Async responses
So im trying to use async for the first time, i am making a few api requests one after the other, the issue is that the order gets mixed up since the requests are being made asynchronously. I need a way of ensuring the order is enforced
61 Replies
wdym "order gets mixed up"?
can you show the $code, and say what you're expecting to happen?
(and what's actually happening)
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/its messy, but sure
this gets messages from the database, then displays each one in turn
theres some catches below
shit
wrong bit
Nothing here is async so far
was about to say, but my name could come in handy soon 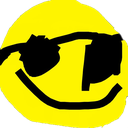
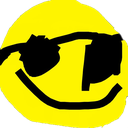
Nothing async yet
Well,
async void
var response = await Task.Run(() => http.GetStringAsync($"myapi").Result);
that's not asyncvar response
And
await Task.Run()
for some reasonjust await GetStringAsync directly
no task?
This is a doozy of a line lmao
why would you wrap an awaitable tasks
.Result
in task.runTask.Run is for shunting CPU-bound work to the thread pool
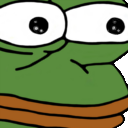
It's an awaited task that runs an asynchronous method in a blocking, non-asynchronous context lol
it is unnecessary here
my friend said the same thing but wasnt sure how to explain
so ive come here
the use of
.Result
is also completely wrongYou're stripping the asynchronicity away with that
.Result
then you add it back with Task.Run()
A zero sumin any case, you mentioned multiple requests, but I only see the one
.GetAwaiter().GetResult()
if you're going to make an asynchronous method call synchronous by the way, for future reference
never .Result
oh I see, multiple calls to DisplayNewMessage
first part of the code i sent
DisplayMessage()
should be async Task
first of allin any case, just because you send multiple requests in a given order, doesn't mean they'll return in that order
thats the issue^
if i cant, ill have to run without async, which i think would be terrible
if you want them in parallel, you'll just have to deal with that
I mean,
will ensure the order
you could queue up the responses somehow, and then run through that queue
Since one call will wait for another
true
would it work, to get all the responses, without adding them to the UI display, and sort them by the "dateSent" property they have
yes
then add them all
that would work?
or this
As long as at no point do you have
async void
, it'll be fine
Because only Task
can be awaitedvar response = await http.GetStringAsync($"myapi");
oh
how do you do your slightly dark background?`code here`
gotcha
ty
this here requires private
async
voidno it doesn't
use
async Task
async Task
is the async version of void
you should only use async void
when you have toT
=> async Task<T>
int
=> async Task<int>
void
=> async Task
ok i see
i think its a little better now, going to try the sorting thing i had
void
is nothing.
Nothing cannot be awaited, cannot be cancelled, cannot be queued... because it doesn't exist.
So you need a something, a Task
is that somethingthat makes a lot of sense, until now void was just "doesnt return anything"
it still is
which is still true, just very vague
^^yup
well thanks guys, had some input from everyone which was nice :))
until next time
trying to use this, once the code hits the await get async part, it stops executing and returns to the UI as it seems
Ah, you're using WPF or some such?
winforms
Uh, yeah, at some point you'd need to use... what was it,
Dispatcher.Invoke()
or something?
I don't have much experience with GUI frameworkshm ok, it was working fine before, with the same api request code
from within one Task, can i make multiple api requests?
Sure
this does not work
this will work, just not in order
the http request is the same
Don't instantiate a new client with each loop iteration
ah i had it above actually, i moved it inside to check
since thats what the second version is effectively doing
Yeah, idk, As I said, I'm not the best at desktop stuff, let alone Winforms
@jcotton42 might know more?
all good, appreciate the time regardless, just threw it in here hoping someone might stumble across it again
anyControl.Invoke
iircim unsure what that means
the Invoke method on any control
like
someTextBox.Invoke
, someCheckBox.Invoke
etc.Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.