❔ index out of range or non-negative
im pretty sure that im checking if it ok but i dont know what im doing wrong
83 Replies
Run the debugger, see if there actually is at least 3 elements in every list
i do not know how to do that
?
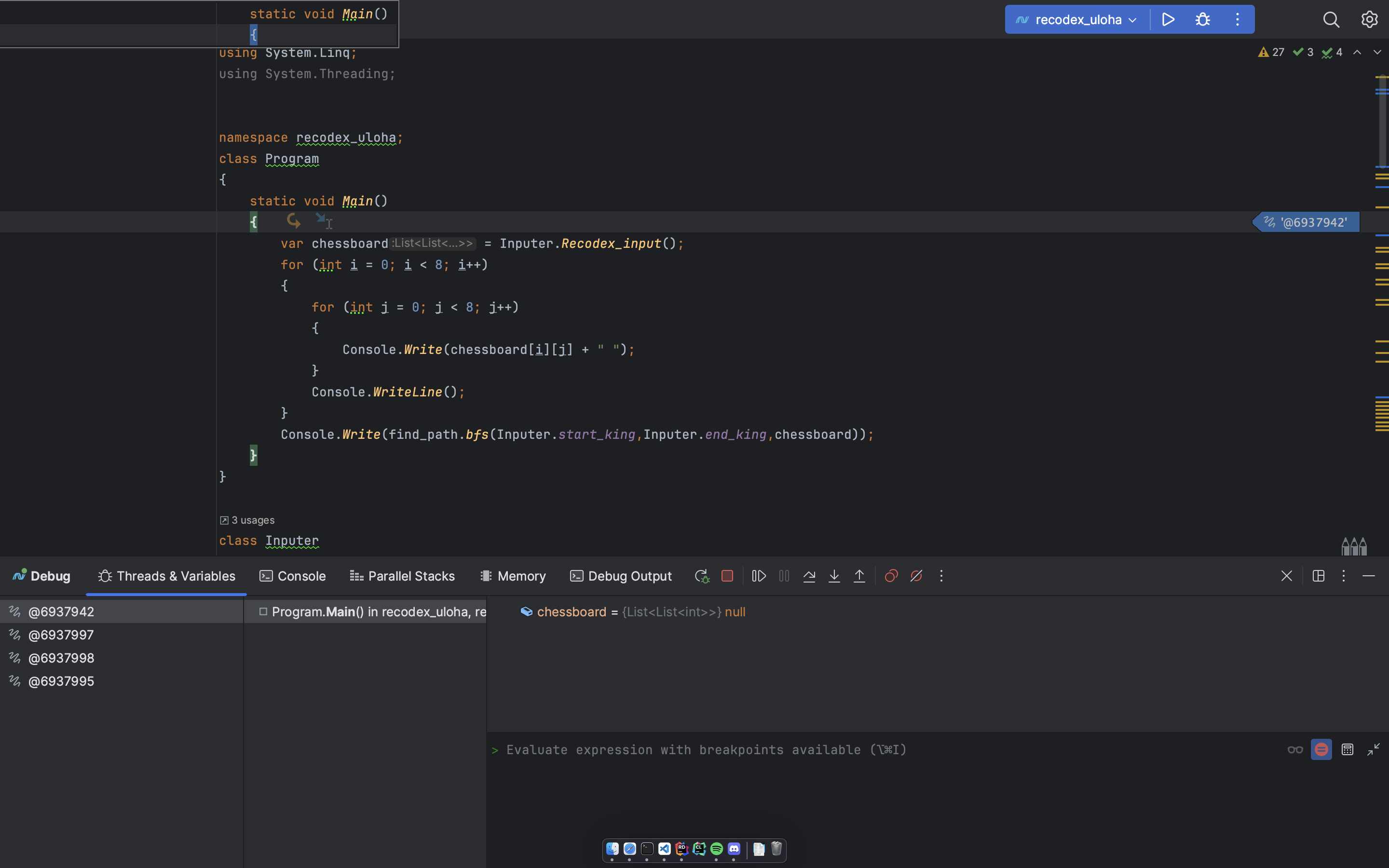
@Angius i get these erroes also error CS8804: Cannot specify /main if there is a compilation unit with top-level statements.
Program.cs(3,1): error CS0103: The name 'Console' does not exist in the current context
it wasnt
i added the x.add(0)
and it helped with the index but its always printing 2
or -1
so i dont think like the generations and incrementing
@Angius im beggin man im losing my fcking mind
@Angius
this was my other attempt
to like add the third value before hand but still nothing
$debug
Tutorial: Debug C# code - Visual Studio (Windows)
Learn features of the Visual Studio debugger and how to start the debugger, step through code, and inspect data in a C# application.
Check what
x
isim not using visual studio
Then google how to use the debugger in whatever you're using
Or
Console.WriteLine()
iti did that and it stops in like 2 Xs dont know why
Well, one of them has fewer than 3 elements
You can try safeguarding against it, for example
i mean if there is a way that i can do it differently
or some such
my x shoud contain like the x coord y coord and some num with number of steps to get there
it was suggesting count
to me
So use count, then
i did that with count and it didnt print anything
Also, did I get it right, that the list contains three different kinds of data?
As in
x[0]
is the x
coordinate, x[1]
is the y
coordinate, and x[2]
is some number of steps?well they are all ints
yes
Use a class, then
Not a list
well i dont know how to implement that i just rewrote it from python to c#
No better time to learn than now
but like what do you want me to do
how should i use class for it
as you can see
im adding it to queue
and then i go trough each one to check if it has the correct x y coords and i want to print the steps
And make it so that this is your
x
so the class will be my list and i will add bunch of classes inside a queue
Yep
will the class return a list?
No, why would it?
It describes one, self-contained object
Not a list of things
well then i will check if whatever.whatever(x) is equal
to x coord?
?
whateverOne.X == whateverTwo.X
That what you mean?well my finish coordinates are the same
so i will not put them in the class beacuse they dont change
what you mean with the One and Two
im just asking what will i write with this class to check the x value
whatever.X
This will give you the value of X
From an instance of Whatever
named whatever
$get
$get:
i dont know what public int X { get; }
public int Y { get; }
public int Steps { get; }
should do
Ah, now I know what you mean
Thought you're trying to invoke some random bot commands
Those are get-only properties
$getsetdevolve
can be shortened to
can be shortened to
can be shortened to
can be shortened to
Lesser languages like Java need whole getter and setter methods
C# has autoproperties
get; is like a readonly field
on so i just set that x coords is readonly field
and they i set it
should i maybe do it like this
well there is something wrong with non static and static but i have no idea also do i put int[] before int_values()
ok well it is somehow working now
but my last problem is
Your problem is the lack of C# knowledge, I'd say
You said you're coming to C# from Python
It'll be hard getting into an OOP language that has strict and static typing from a language where whatever goes as long as the indentation checks out
I'd recommend learning about classes and stuff
Besides that, you didn't follow the code I posted
My code uses a constructor
Yours has a random
int_values
method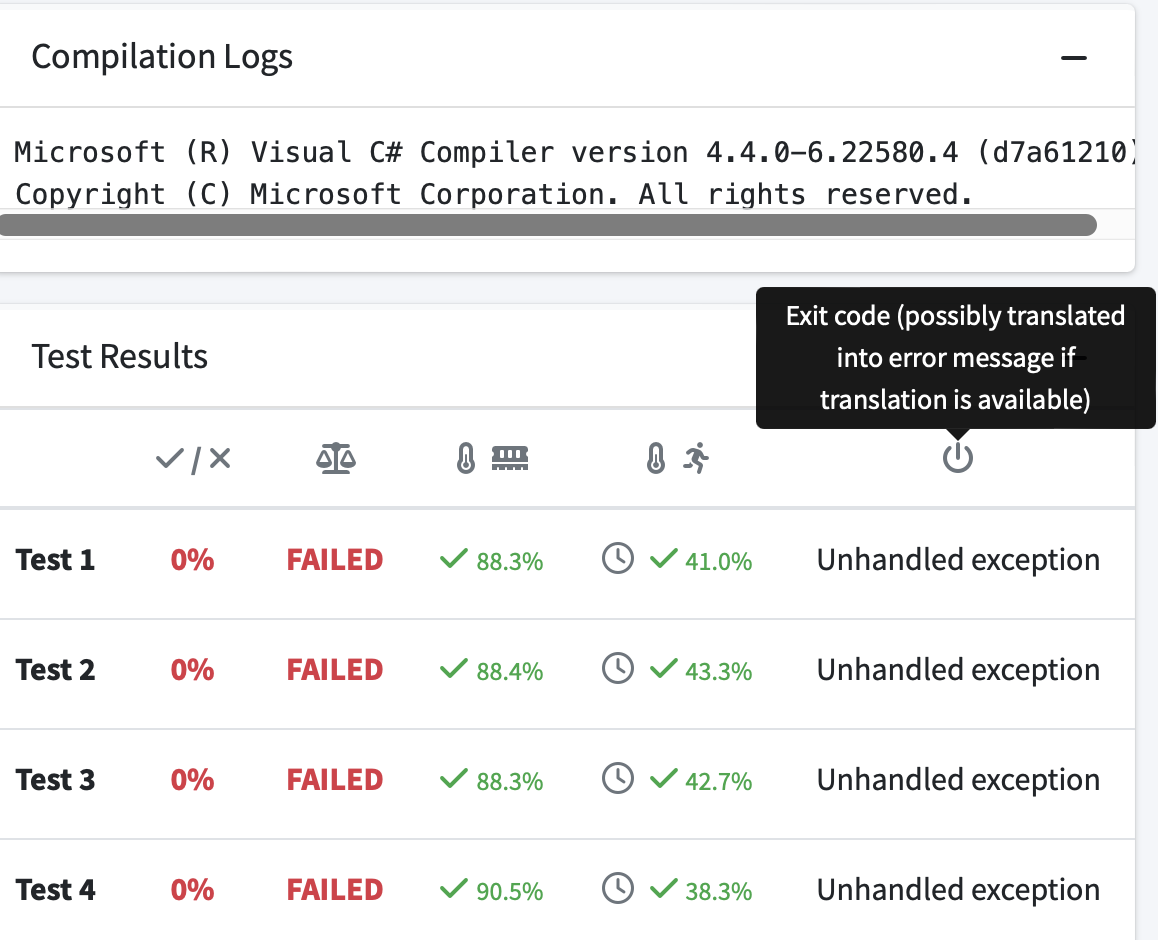
Is that some online IDE?
yep
that checks for test cases
Drop that shit like it's hot and install VS
no im using rider as im on mac
but i write the code and put it on web that it checks if its correct
So why were you having issues with debugging?
Rider has a great debugger
Regardless
Copy my code verbatim
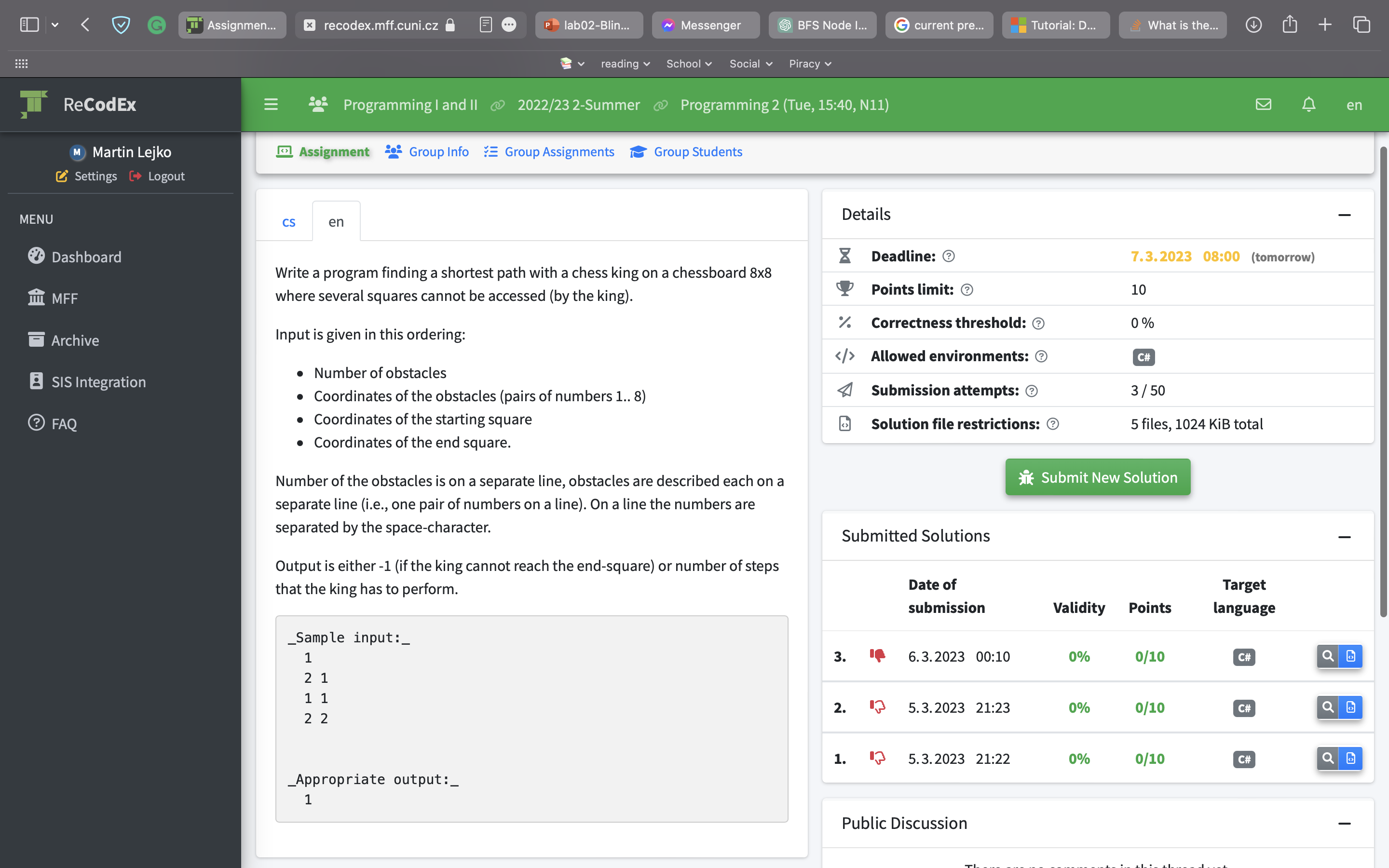
Exactly how it is written
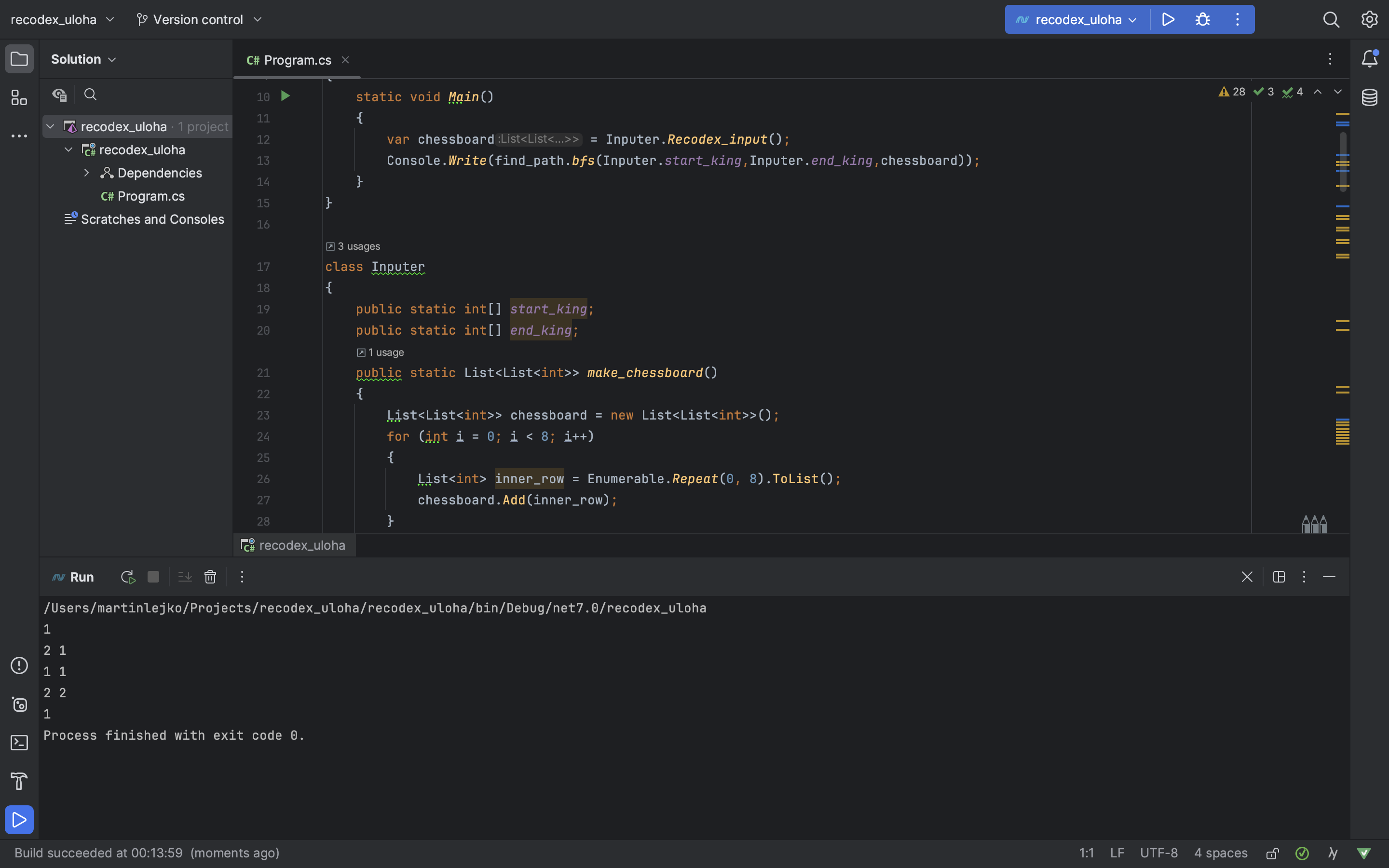
it works fine
Aight, if it works it works, then
Problem solved
but dont know why it still has the
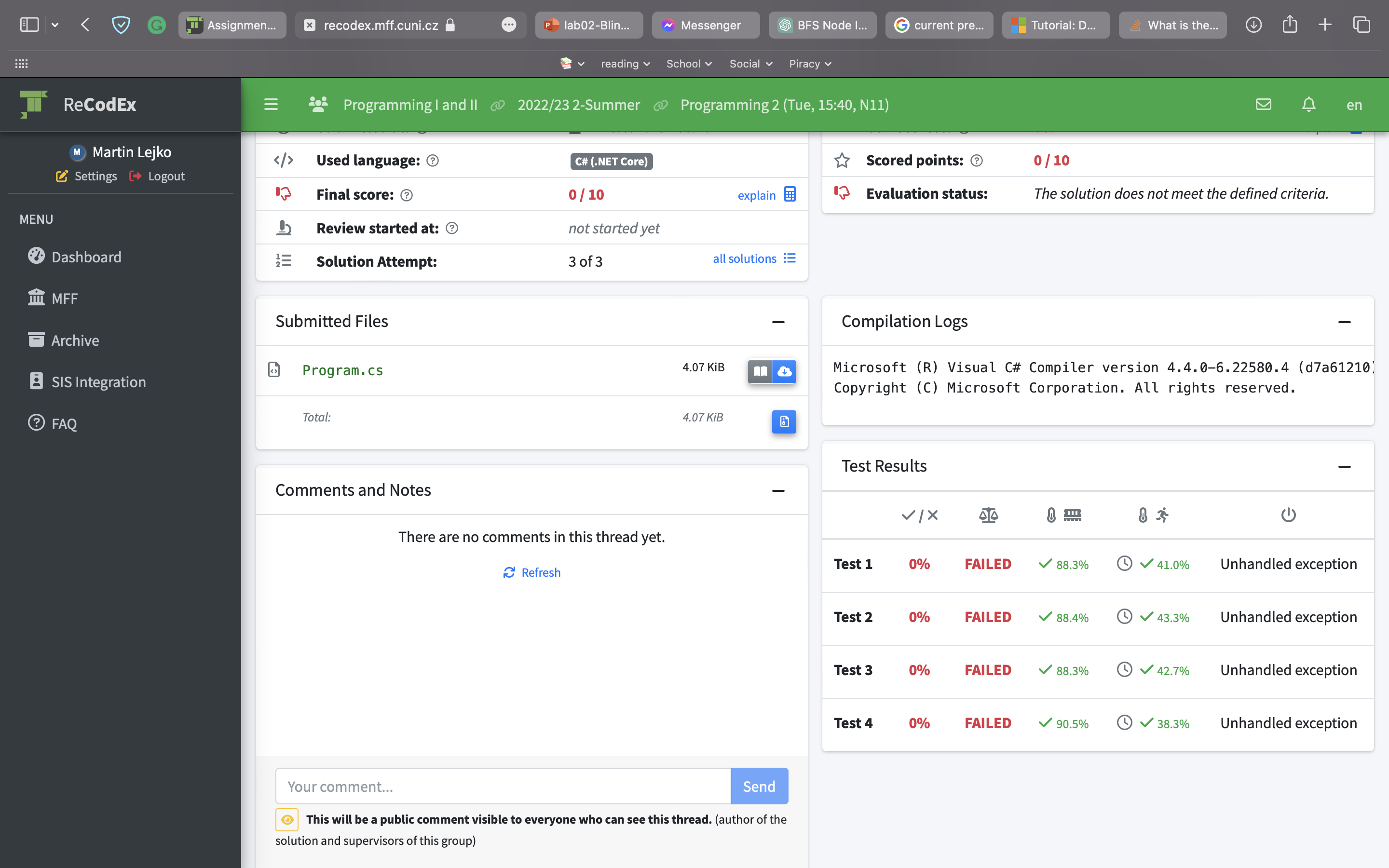
Idk either
"Unhandled exception" is about useless as an error message can get
well
dont know how to solve that will try uni discord
why rider is not showing me this
No clue
Would be easier to tell if this online thingamajig actually showed an error message
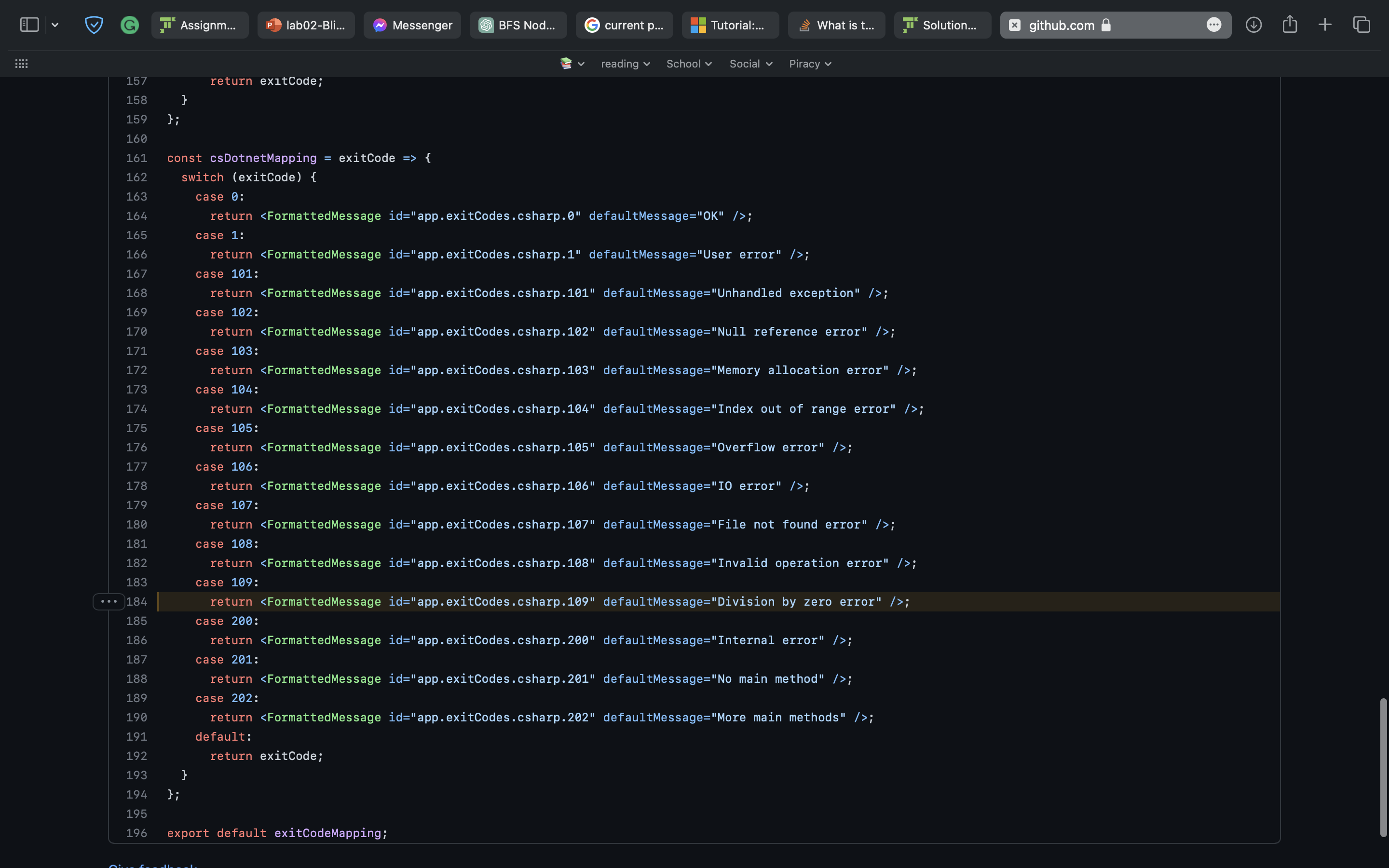
Dunno where you got it from, but if that is the error, it's quite a clear one
its not the devision by zero one
its the one on a line 168
And that's the one I said is useless
So
We're not any closer to any solution
Idk, maybe someone else will have some other ideas
only read this
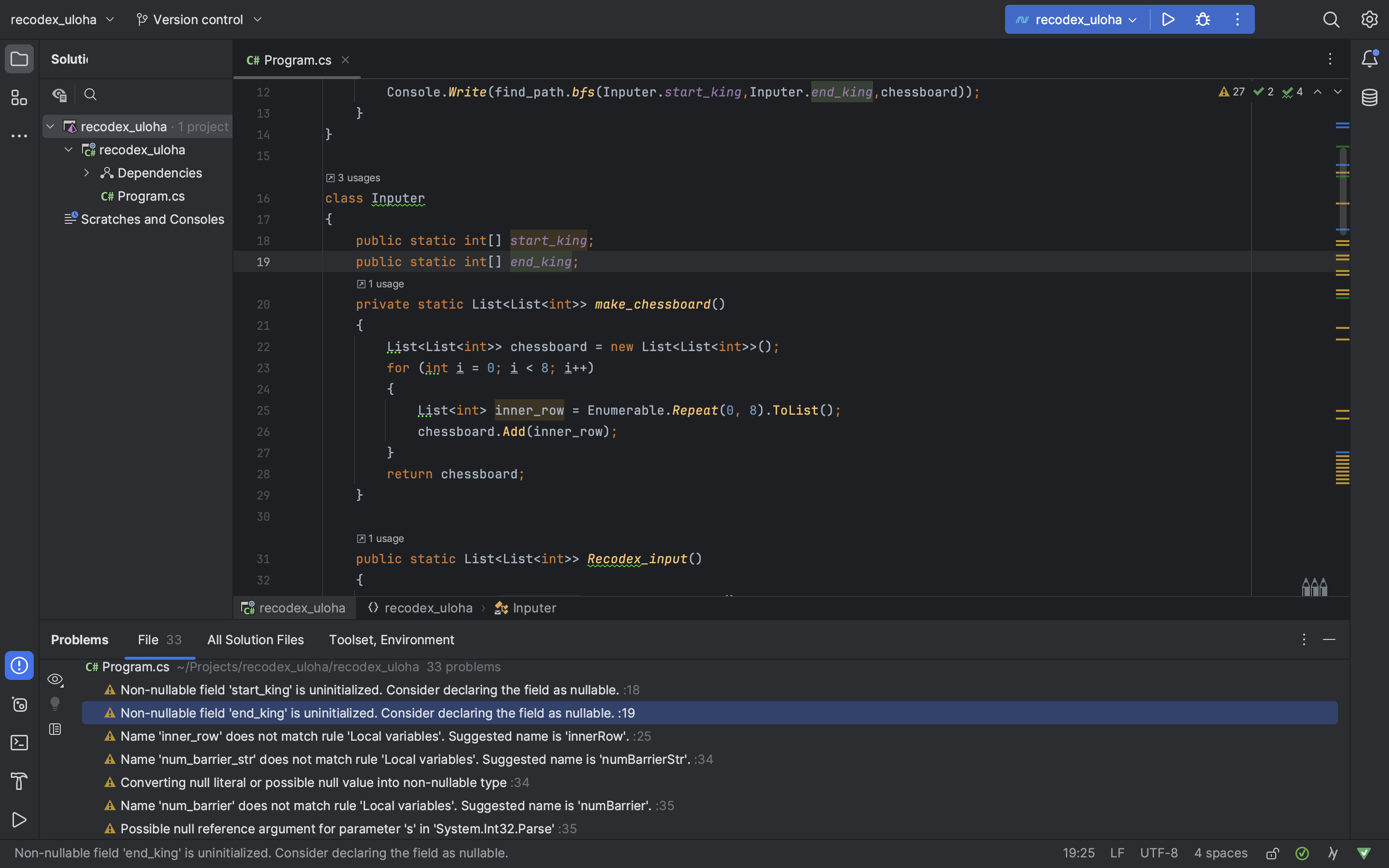
maybe this warning that im getting
Possibly, yes
Just declaring a variable doesn't mean it will have a value
end_king
for example, at this point, does not contain an array
did it like this
Sure, that will give them a value
do i also do the
or just
Nowadays, file-scoped namespaces (the second one) are valid and preferable
But idk what version of .NET that online thingamajig uses
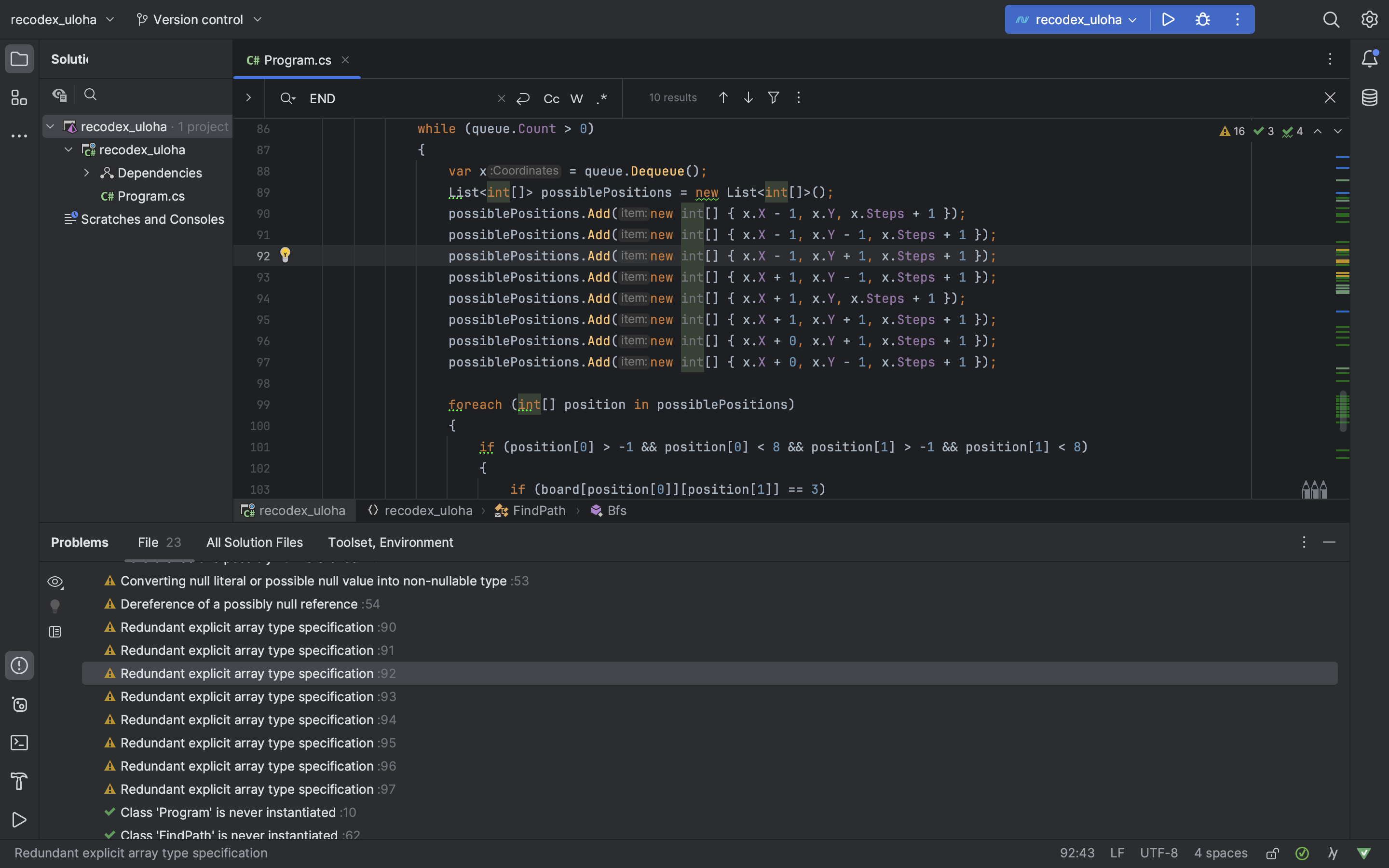
how can i edit this code so it doesnt have the errow
Those aren't errors, just warnings
And it's telling you that you can use
new[] { ... }
instead of new int[] { ... }
Also, ctrl
+space
in Rider will show you quick fixes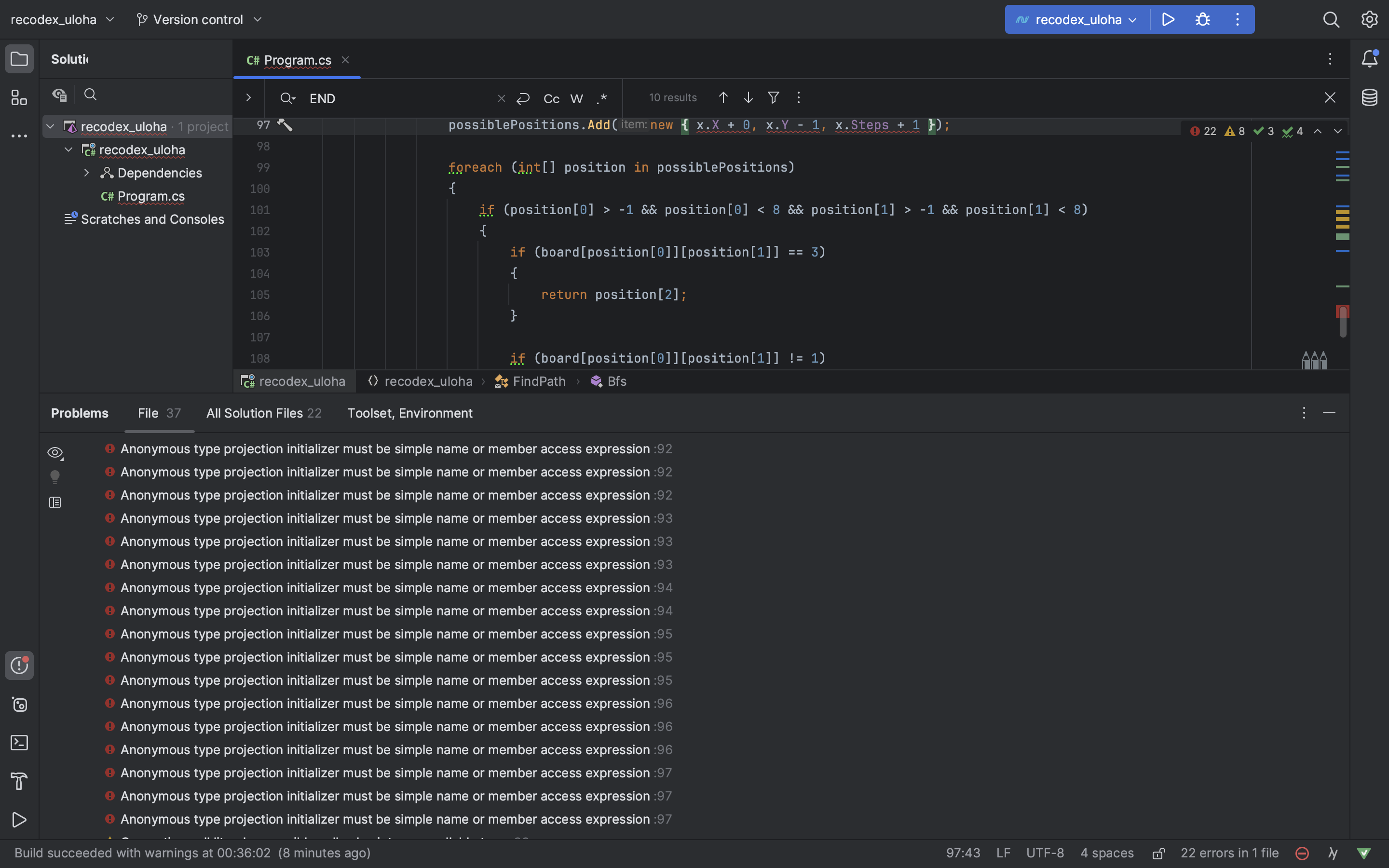
how i have this xd
Because you didn't write the code as I have written it, again
new {}
creates an anonymous object
ye i did it like this
so you want me to just write new {} without anything in it?
The opposite
Read what I said about the warning you were having
Read it in full
Character by character
You will notice that I said
And it's telling you that you can useand notnew[] { ... }
instead ofnew int[] { ... }
And it's telling you that you can usenew { ... }
instead ofnew int[] { ... }
Program.cs(54,21): warning CS8632: The annotation for nullable reference types should only be used in code within a '#nullable' annotations context
got this one this is new
You made something nullable with
?
that can already be nullable by defaultye
i got it
So... don't
but i was one of the quick fixes xd
Maybe Rider made the assumption that you have nullable reference types enabled, or it was just a suggestion ¯\_(ツ)_/¯
@Angius can i solve it somehow without system.Linq
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.