❔ use of args in method
can someone explain the specific context in which we declare args in the methods?
e.g. for this method - no args are declared whereas for another mtd where new variables are introduced, args are declared.
Here's code:
32 Replies
You want arguments when you need to pass something into the method
If the method doesn't take any input, you don't need arguments
ahhh - is that the only context where args are needed? when passing input into method?
Thats literally what they do 🙂
Got it! 🙂
Example: I want to write a method that gets me the chinese zodiac sign for a given year
Would this method need an argument?
Im thinking yes - since we need to input zodiac info?
You need to input the year
ahhh right
I also have another question, I dont really understand the use of the constructors here (it seems like unnecessary double work) - is it 'right' to completely omit it?
constructors
?Constructor is there to ensure the values are set when creating an instance of this class
is your only constructor here
yeap - thats the one im reeferrng to
What is questionable, though, is the use of properties with backing fields instead of autoproperties
what do you mean!
Also, those are fields, not attributes
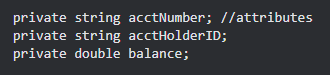
$structure
For C# versions older than 10, see
$StructureOld
$getsetdevolve
can be shortened to
can be shortened to
can be shortened to
can be shortened to
But yeah, a contructor (commonly called a ctor) has one responsibility: Make sure your object has a "valid state" when it is created.
can an account exist without an account number?
nope
okay, then it MUST always have an account number
so a constructor is a good way to guarantee that
i see, and the 'number', 'holder' and 'bal' fields are just used as placeholders in this instance?
they are method arguments 🙂
they are values passed in to the constructor
if your ctor doesnt do anything with them, they do nothing.
why cant i do this
you can, but now you have an account with no account number
or well, a blank strink is the account number. not a great number.
got it! Thanks so much!!!
another question, if i were to use to auto 'get' 'set' property, how will the system know which variable im pointing to without explicitly calling it out?
remove your
attributes
they are actually fields
"auto-properties" dont need explicit fields, they make their own
something like this 🙂Got it - this makes perfect sense!
Alsoo, i wanted to look for practice questions related to object oriented programming - specifically around inheritence and polymorphism - are there any repositiories you recommend? 🙂
Not that specific, no.
no worries! 😄
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.