How can I create a listener for @discordjs/voice states?
Title kinda explains itself lol, but how can I convert things like into Sapphire Listeners to put in my
listeners
folder?
I've already got some Listeners set up for default discordjs events but I'm just unsure of how to do it for the voice status events.18 Replies
Solution
Nevermind I found my answer somewhere else
https://www.sapphirejs.dev/docs/Guide/listeners/listening-to-events-emitted-by-other-emitters i found it here
Sapphire Framework
Listening to events emitted by other emitters | Sapphire
By default, Sapphire's event emitter is your client. However, for advanced use cases, you can specify a different
Hey dylann! Sorry for the ping and replying to this old topc, but could you please share with me how you did this? I also would like to add voice related listeners.
I don't really remember anymore
apologies
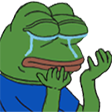
bummer, i was hoping you have a working project that used it, thanks anyway 🙂
Assuming similar code as Dylann's original post
Thanks! Yes, I would like to listen to the state of the bot's voiceconnection and an audioplayer. I have an ogg stream coming from a web api and I don't know why the stream keeps stopping after every song..might be stream related not discord, but I want to check.
I was just not sure it's that easy, I thought as these listeners are not attached to the client, there must be some more difficult magic behind to do it 😄
So it's just the same for the other?
player
as emitter and Audioplayerstate.Playing
as the event? Damn Sapphire sure is convenient 😄yes it is. You can make it "attached to the client" though after which you can use
emitter: 'connection'
(i.e. you dont have to import it). You do this by extending the client class and adding properties to it, similar-ish to https://www.sapphirejs.dev/docs/Guide/CLI/getting-started (though what you're really doing is basic JavaScript class extension, it's not sapphire specific at all)I see. I'm still not so versed in JS, but trying to research stuff, sorry if I asked something lame! 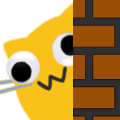
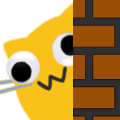
you didnt, no worries
just one more please: how would I access the
oldState
and newState
parameters? input them in the run()
function?yes, the parameter order matches 1:1 with what is emitted.
Bother. Normally I would link docs to show an example of that but the @discordjs/voice docs dont document events on the class. Well you can find what goes where I think.
Oh wait, this is TS actually? bummer I only know JS 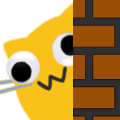
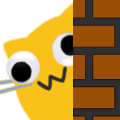
TS is JS, just with additional types.
it's basically the same code
heck when it's ESM (basically modern JS) then it's even closer:
TS is a superset of JS. All valid JS is also valid TS.
Yup, I think that much I knew, but not much more.. okay, I really start to feel dumb, but.. how do I get the
connection
? I need to export it in the command file I create it and import it in the listener?Correct-ish. You're better off doing something like this:

New topic yes