how can i add a function/method to a class that can be changed upon construction?
i'm trying to make a command class but how can i make it so i can provide a response function to the command when i construct a new one? (
this.Respond
)23 Replies
im new to C# by the way
oh hey moles i remember u mentioned delegates
but im not sure how id use it..
elaborate
i tried this but it says

oh maybe i should provide a return type too lol
now how can i change it in the construction?
i cant do
this.Respond = data.Respond;

i see
so how can i do what im looking for?
i need to be able to
create a method/change it upon construction
example if you can?
i hat eto be a bother
im just not familiar with these concepts lol
lambda goes 

i understand how to create a delegate now but
is there a way to process it this way
?
i want to assign it in the constructor if possible
oh ok
how about using
Action<T>
yewhat is
Action
?
ohthere are differen delegate types predefined
Func, Predicate, Action
read online for reference
action is void ye
looks good?
and they all exist with overloads up to like 18 parameters or smth
like Func<T1, T2, T3........., TResult>
oh
is this a macro?
or something of the sort
its used in D#+
to create new commands
we call those
attribute
o
ah
okay so now if i want to make a new command
how do i write the response function?
like to fit the action type
figured it out
i need to move elfinclient
but its going well :D
just
Respond = (message) => //code;
should be enough
omg im getting so good 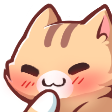
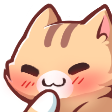
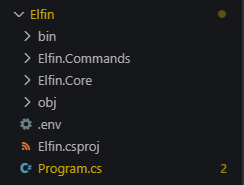
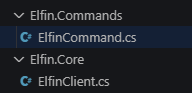

C# is so fun
yep
thanks guys ❤️