❔ Designing an api that can create "fields" with different values
Hi, so I am creating a feature in my API where the user can create kind of a Form, and the user can create some fields with different value types. The field types are bool, string, date, decimal, and a list, where the important things are the name.
The thing i find difficult is how to store the different values in the best way.
I have several different thought, ranging from every field type having it's own class, inheriting from one Field class with common properties, to just store it in one class type, and shoving every possible type in a string, and having an enum tell me what type it is, and just convert it back. This will make it hard to make any search meaningful however. Last thought i had is to have one class type and having it have a BoolValue, StringValue and so on.
I feel like there is some better OOP way to do this that i ain't seeing. This also needs to work with Entity Framework 🙂
16 Replies
I might be missunderstanding but why not create a type that represents the result of this Form and assign each input of the form to a property of that type using events?
the properties will have basic types like string, DateTime, decimal, list each representing an input
I may have been to vague, but the form will be custom. So the user can design it themselves. Therefore i wanna a class that represents the form name etc. and connect a list to that with the available fields to fill
you'd go with something like this:
and a reponse like
It's all generic now
So you would serialize everything to a string value? dates etc?
ye
depending on the
DataType
the front-end would show a date picker/ text box/ checkbox, and the back-end would use it to cast Value to the correct valueYea that was my thinking as well. Only thing i was thinking is the search would be slow on a db?
depends on how you want to search
you could also serialize the form to JSON in your db if your db has good JSON support
If i want to search in a date range?
Using regular Azure Relational DB
try it out, and if it doesn't perform, then pick something else ^^
I've made systems exactly like this
if you need it to perform: use several tables:
* Forms
* Form Field Definitions
* Form Submissions
* Form Submission Field Values
Good idea
So Form Submission Field Values is still just string?
yeah probably
or you make several columns of different data types
and fill in one
Cool
Thanks alot 🙂
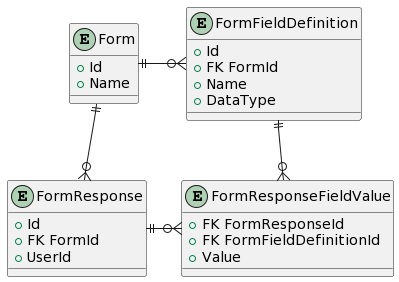
The plantuml :)
have fun!
I've implemented something exactly like this, and it works like a charm
Really nice to know that it works for someone in prod 🙂
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.