❔ Simple Api Fails to launch, why?
My Code:
My Error:
How do i fix this? whats the prblem?
46 Replies
did you bind "IRecordRepository"?
Unable to resolve service for type 'Core.Interfaces.IRecordRepository' while attempting to activate 'Services.Services.RecordService'
Yeah,
RecordService
has RecordRepository
as a dependency, but the latter is nowhere in the DIhmm, can u elaborate?
Your record service needs a record repository
But has nowhere to take it from
Everything you want injectable you need to register first
how would u go about fixing it?
Register the repository
how do i make it take it from somwehre
builder.Services.AddScoped<IRecordRepository, RecordRepository>();
?
Yep
For example
so this:
gives the same error
why?
wth is going on
Show your RecordService ig
Huh, the code being very enterprise-y aside, it should work
However it doesnt 😄
so,, im dead in the water? 😄
Uh, show your RecordRepository too...?
Remove the ctor from your non-generic repo?
It does nothing anyway
Yeah It does
Not in the code you sent at least
Cs7036
it breaks the build
that piece of code is on all the code examples
i saw
Ah, right, because it creates a default parameterless constructor...
still the problem lingers
The problem is repositories over EF 😛
nah that aint correct
Implementing the Repository and Unit of Work Patterns in an ASP.NET...
The Contoso University sample web application demonstrates how users can create ASP.NET MVC 4 applications by using the Entity Framework 5 Code First and Visual Studio.
You don't seem to have
IUnitOfWork
registeredms offican
l
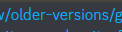

builder.Services.AddScoped<IUnitOfWork, UnitOfWork>();
CORRECT
now
DbContext
is unit of work, DbSet<T>
is a repositorytell me
why?
Angius#1586
DbContext
is unit of work, DbSet<T>
is a repositoryQuoted by
<@!85903769203642368> from #Simple Api Fails to launch, why? (click here)
React with ❌ to remove this embed.
explain to me why this line:
builder.Services.AddScoped<IUnitOfWork, UnitOfWork>();
make this work?
i dont get it
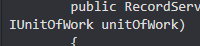
Because you're injecting it
it made swagger paunch
But from where?
launch
As I said, anything you want injected, must be registered first
You were injecting
IUnitOFWork
but it wasn't registered anywhere
You told ASP "gimme the apple from the basket", but the basket had only carrots and motor oilDoes it have to be in this order for it to work?
does the order matter?
Order doesn't matter, not in this case at least
In some edge cases it might, but 99% of the time it doesn't
Gotcha
so every stuff
i inject
anywhere
must be registered first
then it can be injected
got u correct?
Yep
kk
thx
u a life saver 😄
gn thank alot!
Anytime 👌
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.