✅ ForeignKey Entity Framework and Identity
I've got the following classes: "Account", "Post", "Tag" and "Image".
The "Post" class contains the following:
I can't seem to quite understand how this type of mapping works. Why can't the Lists simply reference the correct AccountId? I see no reason there could not be different fields referencing the same "Id" for different purposes.
What am I doing wrong?
12 Replies
Here's the latest error message among many.
Well, this won't work, unless you have a table of GUIDs
In general, lists of IDs won't work
It's because that's how many-to-one relationships in databases work
Databases, in general, have no concept of a list
So if you want a relationship where one post can have one category, and one category can have many posts, it is the post that needs a foreign key to the category
The category cannot have a list of foreign keys to posts
I was hoping I could declare a List<Guid> and have the DB populate it with every associated AccountId one-by-one as they become relevant.
"it is the POST that needs a FK..." - My attempt at this was to create these List<Guid>.
What is your recommended course of action?
To set it up properly
Also, get rid of those
virtual
s while you're at it
Lazy loading is the devilMaybe I can create a "Participator" class and a "Attending" class. Both with "List<Account>" fields.
Something like this
Those coloured pairs will create many-to-many relationships and necessary pivot tables
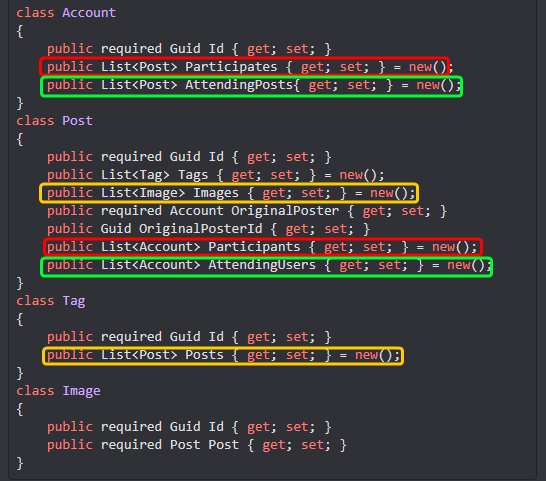
Since there are two many-to-many relationships between accounts and posts, you'll need to configure them a bit
Configuring the post with this should just about do the trick
This utilizes "Fluent API"? Searching in NuGet and wondering whether it's "Henk Mollema" or "Jeremy Skinner" who made it?
Yes, it is fluent config
No clue who made it
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.Thanks ZZ