❔ Enumerating Running Processes
I am missing something, besides brain cells but what should I be returning if I want a ComboBox to have the list of Windows processes?
83 Replies
there is so much wrong here
?
I thought List<T> would be good here, maybe not.
Was making a task manager kind of thing for a tech app
I would pass Processes to List
instead of string
then use comboBox.DisplayMember to show ProcessName
What do you mean?
1 sec let me show you
I do have a List
ok
you're using a
GetX()
method instead of a property;
you're just constantly adding more things to the RunningProcessList
without ever clearing it;
you're not disposing the processes!!;
your return
syntax is completely gibberish;
the above leads me to believe you're not even using a proper IDE, or have never done any kind of c# tutorialI was following a tutorial on YT
your return type is also
string
, when RunningProcessList
is a List<string>
as usual, should of used ChatGPT instead lol
jesus christ no
also it's "should have"
it did help me solve a issue 2 days ago 🙂
w/o googling for 40 hrs
sec
i will check ms's site for process examples
It just works
Oh, that's ALL I needed? 😂
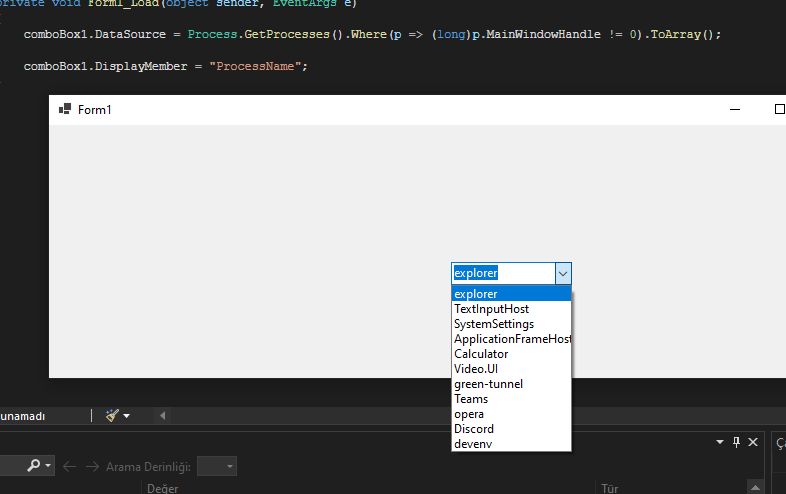
ah the getter/setter stuff 🙂
you need to dispose of the processes
Wait so which one do I need now?
otherwise you're building up more and more memory
Hmm makes sense, garbage collection
Ero's is easier, 2 lines 🙂
I will try both ways
and see what one is effective , since i probably will grab the id too
Using Property is okay but
only returning ProcessName doesn't make sense
Using DisplayMember and ValueMember is better
Yes, I need ID too
ok
so use yours?
i dont wanna have another stackoverflow day heh
the large example doesnt work
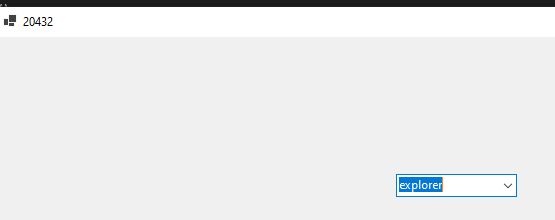
Operator '!=' cannot be applied to operands of type 'IntPtr' and 'int'
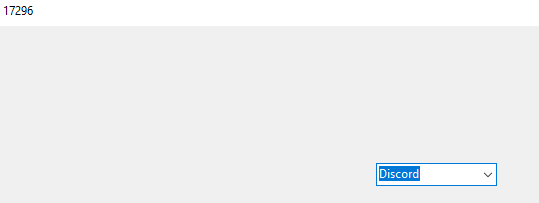
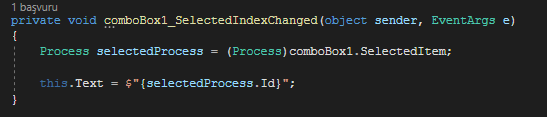
See
I can get id
Because i pass Process itself
Wait so do I use the example they gave but use DataSource and MemberName?
no, you would have to do it a bit differently
What would I need to change?
class side
something like this
it's not that pretty, but
you have to dispose of the process objects
yes the example has that now
var processes = Process.GetProcesses().Where(p => p.MainWindowHandle != 0 && !p.HasExited);
this isnt going to work with IntPtr/int
CS0019 Operator '!=' cannot be applied to operands of type 'IntPtr' and 'int'
yes it is, on .net 7, which you should be using
Why 7?
I'm on 6
because it's the most up to date...?
Use IntPtr.Zero
Ero#1111
REPL Result: Success
Result: bool
Compile: 406.433ms | Execution: 28.379ms | React with ❌ to remove this embed.
Yes but I need yo support Windows 7 still for some legacy people
no you don't
ty
also????
.net 7 definitely works on win7
oh ok
.net 7 it is
how do I add it? I'm on VS 2022 Community
should just be in the installer
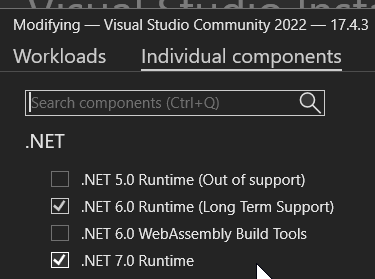
ty
Wonder why VS 2022 is still Preview , or is that still a thing?
the preview is always gonna be ahead of stable
preview and stable are different builds basically
I don't need to keep 2019 still right? I got both
nah you can uninstall that
ok
as long as 2022 is stable enough then, np
I have to relearn .NET now, 7 is new
there is basically no difference
Ah, just a new # then
What is a good video tutorial to watch these days without selling my kidney?
Tim Corey is cool but I don't have a job atm.
no clue. i've never used any tutorials
I appreciate the help regardless @Ero and @Samarichitane, thanks. 🙂
Updating VS 2022 and will remove 2019 too
You can use too
that would make sense, its similar to != 0 so i can understand it
welp, time to learn .net 7 and WPF
im happy to see WPF has the new tech where you can have source generators now
makes stuff easier
props like this are generally considered to be quite smelly
it should be a method
why would that be?
new list every time?
too much work for 1 unit?
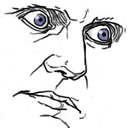
what side effects would this possibly have?
prop gets should be cheap, side-effect free, and the same value as last time unless something caused it to change
diposing the processes returned by the last get of the prop
i don't get how that's bad
you're also returning a private list, allowing it to be fucked with
i mean the whole class is internal
i expect it to only be used by people who know what they're doing
return it
.AsReadOnly()
for all i caredoesn't matter
code should be resilient
can't argue with that
still just don't deem it a big deal is all
it's bad code
this would not pass even the thinnest of code reviews
when I do a get on a property, I do not expect it to have surprising side effects
get should have zero side effects
plus; there is an expectation of immediacy on a get
What if with expression bodied property ?
that's not any different
is the same as
i guess u r right
It would be less bad if you only disposed the processes which needed it, there's not much point of a cache if the entire thing is invalidated every use.
Note, you don't really need to Dispose them. The finalizer will do that on GC. It's good to do so, but you won't leak if not
well, the idea i got from this was that yes, the whole point is that it will get invalidated every use; every time it gets called, it needs to re-get the processes, in case there are new ones, or ones that closed.
If you wanted to be really safe a solution would be to wrap it in your own disposable container.
Notepad code following
Imo that's just overkill though
oh :x
no idea what half the stuff is, like DisposibleList, must be new for 7
but will check it out
Its my own class, I define it in that block
Can I borrow it? Will add credit.
Sure, but please dont credit it's not great 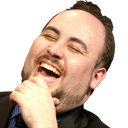
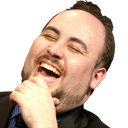
What is great anymore?
many changes in programming and now there's AI taking jobs lol
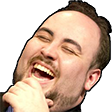
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.