❔ Object reference not working (unity)
Hello i am somewhat new to coding and am having an issue in my code and i cant identify it so if you could help that would be appreciated!
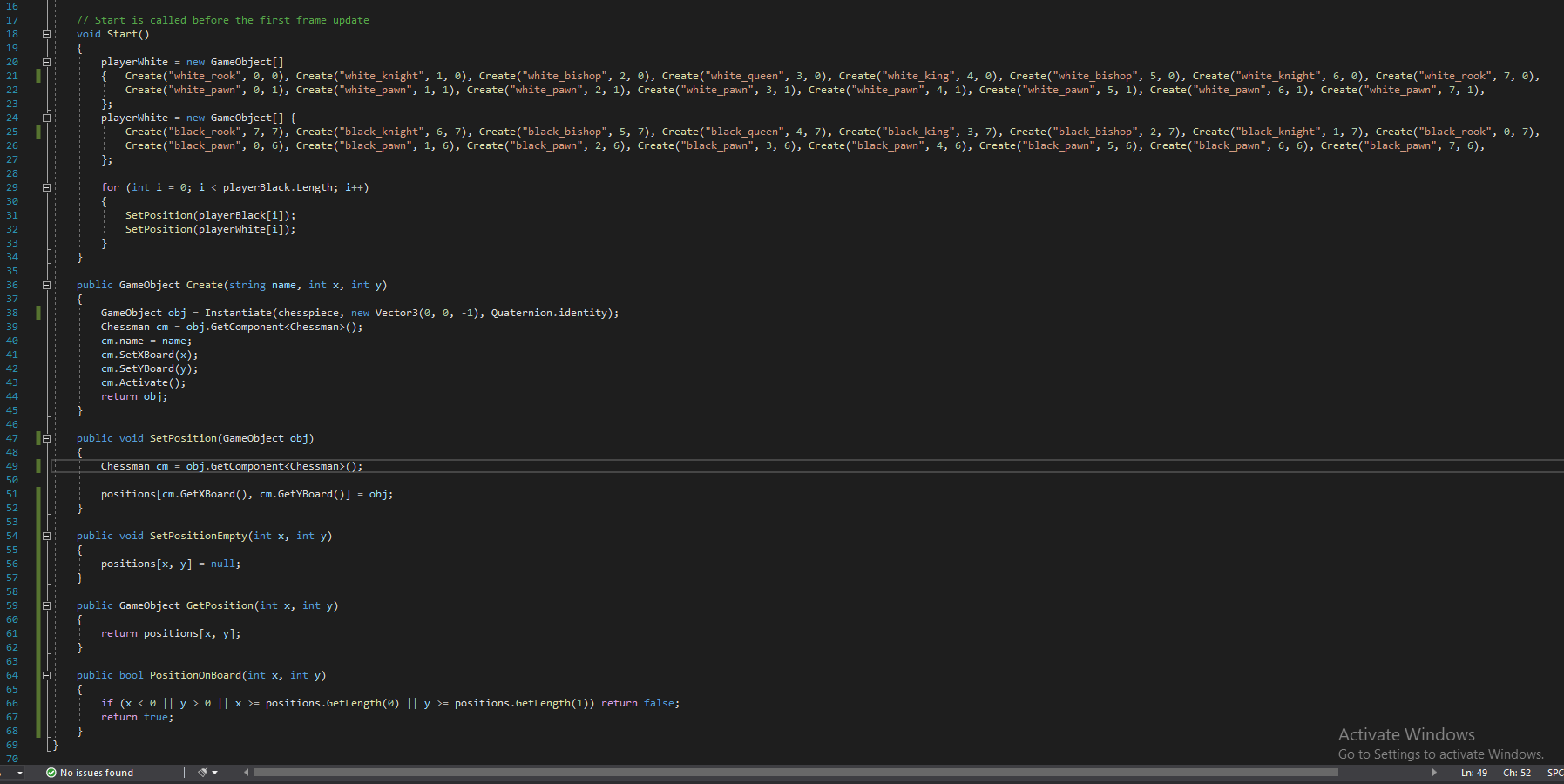

21 Replies
if you have any questions about my script feel free to ask
can you post the code somewhere that isn't a screenshot? I'm old and can't read that 😦
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Game : MonoBehaviour
{
public GameObject chesspiece;
private GameObject[,] positions = new GameObject[8, 8];
private GameObject[] playerBlack = new GameObject[16];
private GameObject[] playerWhite = new GameObject[16];
private string currentPlayer = "White";
private bool gameOver = false;
// Start is called before the first frame update
void Start()
{
playerWhite = new GameObject[]
{ Create("white_rook", 0, 0), Create("white_knight", 1, 0), Create("white_bishop", 2, 0), Create("white_queen", 3, 0), Create("white_king", 4, 0), Create("white_bishop", 5, 0), Create("white_knight", 6, 0), Create("white_rook", 7, 0),
Create("white_pawn", 0, 1), Create("white_pawn", 1, 1), Create("white_pawn", 2, 1), Create("white_pawn", 3, 1), Create("white_pawn", 4, 1), Create("white_pawn", 5, 1), Create("white_pawn", 6, 1), Create("white_pawn", 7, 1),
};
playerWhite = new GameObject[] {
Create("black_rook", 7, 7), Create("black_knight", 6, 7), Create("black_bishop", 5, 7), Create("black_queen", 4, 7), Create("black_king", 3, 7), Create("black_bishop", 2, 7), Create("black_knight", 1, 7), Create("black_rook", 0, 7),
Create("black_pawn", 0, 6), Create("black_pawn", 1, 6), Create("black_pawn", 2, 6), Create("black_pawn", 3, 6), Create("black_pawn", 4, 6), Create("black_pawn", 5, 6), Create("black_pawn", 6, 6), Create("black_pawn", 7, 6),
};
for (int i = 0; i < playerBlack.Length; i++)
{
SetPosition(playerBlack[i]);
SetPosition(playerWhite[i]);
}
}
public GameObject Create(string name, int x, int y)
{
GameObject obj = Instantiate(chesspiece, new Vector3(0, 0, -1), Quaternion.identity);
Chessman cm = obj.GetComponent<Chessman>();
cm.name = name;
cm.SetXBoard(x);
cm.SetYBoard(y);
cm.Activate();
return obj;
}
public void SetPosition(GameObject obj)
{
Chessman cm = obj.GetComponent<Chessman>();
positions[cm.GetXBoard(), cm.GetYBoard()] = obj;
}
public void SetPositionEmpty(int x, int y)
{
positions[x, y] = null;
}
public GameObject GetPosition(int x, int y)
{
return positions[x, y];
}
public bool PositionOnBoard(int x, int y)
{
if (x < 0 y > 0 x >= positions.GetLength(0) || y >= positions.GetLength(1)) return false;
return true;
}
}
You never create any players
Well you do, but in guessing you're using those arrays before there's anything in them
Doesn't the error tell your what line the problem is on?
i have my other script chessman which created the black and white player
its apparently on line 49 or "
public void SetPosition(GameObject obj)
{
Chessman cm = obj.GetComponent<Chessman>();
positions[cm.GetXBoard(), cm.GetYBoard()] = obj;
}"
And what's the error? obj is null?
yeah it saying object reference not set to an instance of an object
Yeah, you don't have any
Attach a debugger
It's ab simple mistake
i tried attaching a debugger and im getting another error saying i need to use a preview version for a line of code used by the debugger
Are you on a dodgy copy of Windows?
No
Why isn't it activated then?
Oh if thats what you ment yeah
Sorry, we can't help you here
Oh alright
What’re you talking about, why are you being rude to him for not having an activated windows license?
Anyone can use windows 10/11 for free without breaking TOS
If you want bonus features, you pay for a license. Illegal would be activating your license without paying
@Forever#5620 when pasting code try to use markdown
I'm unsure which part is the null reference. Is cm null in SetPosition?
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.The
playerBlack
array items have not been initialised. Change playerWhite
to playerBlack
here: The problem is that your editor is not properly configured to use Unity, judging by the obvious lack of syntax
But the guy already left the server 🤷
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.