❔ Is there an easy way to serialize a Dictionary<string, string> to XML nodes?
I'm writing a program that has to produce the following XML:
I'm trying to figure out the best data model that can be serialized to something like this. The most obvious choice seems to be a
Dictionary<string, string>
, but I can also use a custom class with two properties (Name, Value). I've seen some solutions on stack overflow, but all of them involve creating a bunch of helper classes and writing hundreds of lines of additional code, and I refuse to believe that there is no simpler way of doing this, with Xml attributes or something like that.5 Replies
Generally speaking, XML is a bitch
Surprisingly so, seeing how fond of it Microsoft is
Oh yeah. I'm currently trying to write a
IXmlSerializable
to see if I can get it to work..
Yeah, I think I solved it.
Jesus, the more I read about XML serialization the more I hate it
Why does the serializer use
typeof
instead of being generic? Why does it insert data into a stream? Who designed this shit...?
That said... this works
Kinda
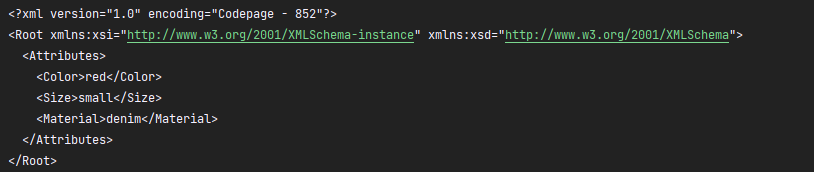
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.