✅ CORS: Cross-Origin Request Blocked
Hello, I'm trying to figure out how to configure CORS on my .NET Web Api Application.
The Api Application is hosted on
localhost:5000
, while the ReactJS Frontend is hosted on localhost:3000
.
Also have defined Application to use Cors in Program.cs
I've followed this from MSDN: https://learn.microsoft.com/en-us/aspnet/core/security/cors?view=aspnetcore-7.0Enable Cross-Origin Requests (CORS) in ASP.NET Core
Learn how CORS as a standard for allowing or rejecting cross-origin requests in an ASP.NET Core app.
47 Replies
"http://localhost:3000/*"
should be "http://localhost:3000"
That's the host, or originLemem try this
Nope, this did not work either
Which makes sense, cause I could've sworn I tried this
Well it's one of the things you should do
You sure you run on port 3000?
Alright, that's one
Yes
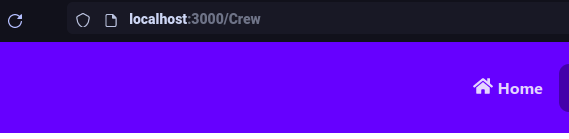
And have you referenced the actual policy? I don't see it specified anywhere
What is the policy? I think that might be the issue
Cause I didn't do anything like that
You add a policy, but you can also just set the default policy
In your case you made the policy but you did not reference it
1 sec
OHHH, I need to use default policy
lemme try that real quick
This is what I did with my last API
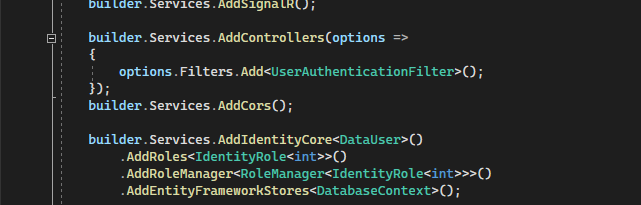
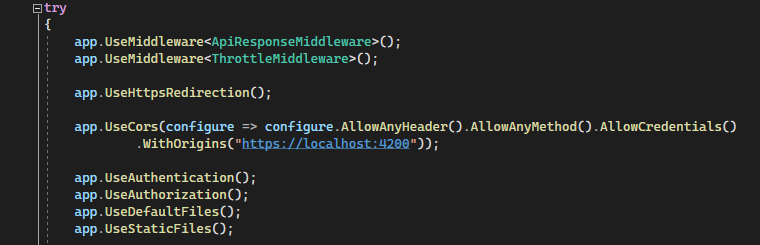
I didn't specify a policy and just used a default one
In your case, you need to specify the policy to use in
UseCors
, like the picture shows, or you do what I did.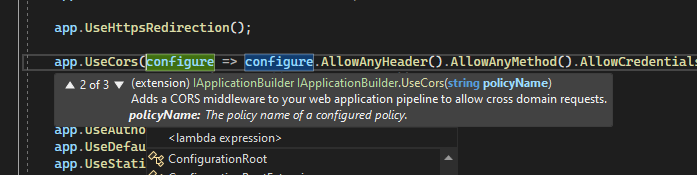
k brb, lemme rewrite some code to try this
Okay, so here is what I've done:
Static Startup Class (Ignore the function names, that's temporary)
Program.cs
This doesn't work either
I'm gonna try default policy too
Nope, neither this, nor the default policy example from MSDN worked
Maybe you need to add
AllowCredentials
to your CORS configuration?
Because it's not a whole lot of code, I assume if you copy paste my example it would workYeah, I've just tried that, it's building
Added
AllowCredentials
in both your way and the MSDN ways
Both did not workHuh
Maybe a weird question, but you call both
InitializeCORS
methods?
And you're sure both invoke, and not one twice for example?
What exactly is the error?Let me recheck, but I'm like 95% sure they should both invoke
both of them do execute
But wait, lemme just recheck something real quick
this is the error btw

On the frontend

Yeah double checked, both of them are being invoked
And each only once
Any other ideas @FusedQyou?
Can you try this?
I fixed this by going to the Properties for my .Net Core app in Visual Studio. I selected Debug from the menu on the left hand side. There was a checkbox on the page called Enable SSL along with a URL (https://localhost:44358/ in my case). I browsed to this page in Firefox, got a warning page, added a security exception for it and everything worked as expected after that.Self signed certificate bullcrap can also cause this Maybe try adding localhost on post 5000 as an accepted origin Probably not the problem but I'm getting confused by the error. Not sure which one is the frontend url now
3000 is frontend
5000 is backend (dotnet)
Is your frontend using a self signed certificate?
Since you only accept an unsecure connection
No, both without SSL
using only http
Does your .NET pipeline force http to https?
Nope
Like I have
app.UseHttpsRedirection()
here
Then I'm really confusedSince, I use it with thundercleint, and I get responses fine
Also, I'm on VSCode on Linux, setting up SSL will take me a bit, I'll get back to this thread once I've set it up
I don't think that's the problem though
But idk what else you could try
Also, another note, the site is opening fine in browser
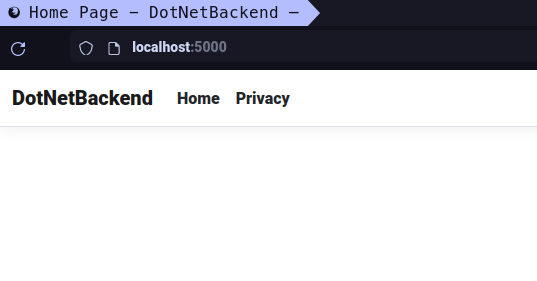
Might be a header you're adding/is missing
Update: trying it with
*
does workDoes
AllowAnyOrigin
work?Why doesn't
http://localhost:5000/
? 🤔I did this
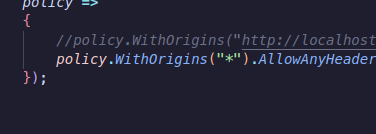
I don't want to allow all origins, I want to specifically allow only
localhost:5000
Well it has to be
http://localhost:5000
, without the slash
With the slash represents a full url, not the host. It's weird, I know
Did you do it with the slash the whole time?Yes, I did
Let me try that real quick
Then that was the problem lol
I told you to do it without the slash at the end in my very first message <:peepo_cry:640304738512142352>
That, and the fact that you're not actually referencing the policy - but I assume you fixed that
Yup, that was it! hahaha
it works now, lmfao

I have this habit of not reading properly 

Same
Thank you very much, my dude
Appreciate you sticking with my dumb ass for this long haha
No problemooo