✅ mistery -foreach cycle executed three times for no apparently reason
Here is the code.
I checked more and more times for errors, I always putted some breakpoint in order to see into variables, and I just seeing that when the cycle arrive at the last char contained in input (that's a string), it just rest all index to 0 and start again for a total of 3 executions.
Am I committing error somewhere? 🧐
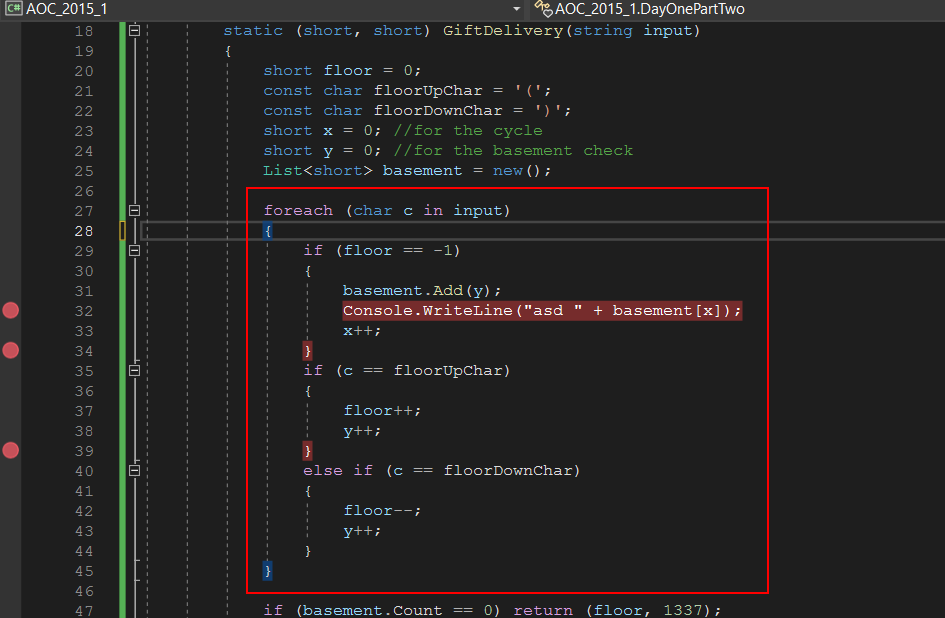
41 Replies
that's not possible
yeah I know, usually stupid errors are committing by beginners in cycle statement, but in this case I'm really going crazy, I'm pretty sure all it's ok
it's like it re-execute those rows again, out of the cycle 🧐
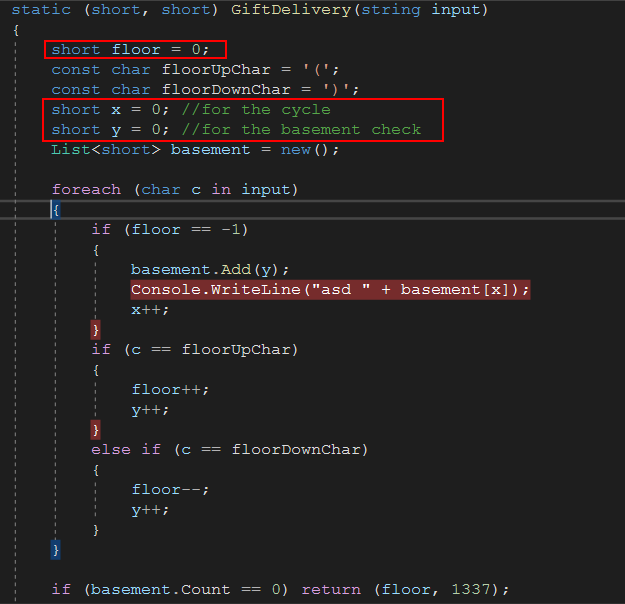
can you show more of your code?
the only explanation is that the whole function is called more times
like where that method is being called?
I think it's so <:picard_facepalm:616692703685509130>
you literally call that method 3 times yourself
damn
4 times even
Console.SetIn(new StreamReader(Console.OpenStandardInput(), Encoding.UTF8, false, 8192));
is absolutely unnecessary (and a memory leak)Call it once, save the result in the variable, reference that variable
Since you're using an unnamed tuple, you could even use tuple reconstruction to make everything nice and named
and
.Item1
will be in foo
and .Item2
will be in bar
yeah I like this
one of the first modifies I made to the code consist in this and I didn't want to reverrse it
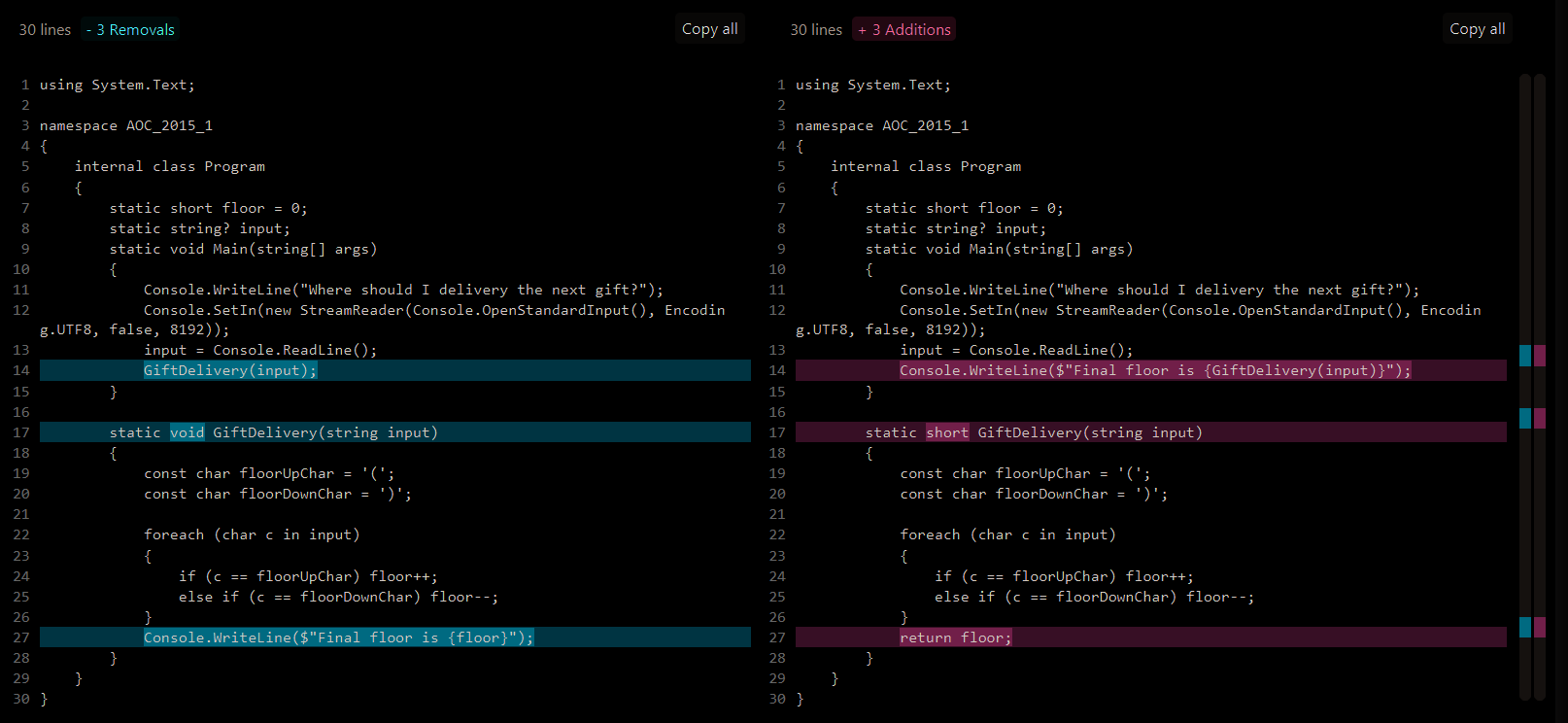
is
var
better than short
?var
is for type inferenceoh ok I have to use it mandatory for avoid calling twice the method
It is
short
if you assign a short to ityeah but I also noticed that
does not works with
short
also if I use short
everywhereWym "doesn't work"?
what do you think about converting rows like this:
to this:
could it be a good practice or it's stupid?
it doesn't seems to accept it, now I try again
the method has to actually return a tuple
Angius#1586
REPL Result: Success
Console Output
Compile: 672.899ms | Execution: 88.116ms | React with ❌ to remove this embed.
and can't a tuple have a defined type?
that's what I tried to do

No
Because a tuple can contain a string and an int, for example
Tuple deconstruction needs
var
ok
as I know, in DB a tuple is a row
I'm reading that a tuple in C# can contains multiple values, isn't like an array so?
Kinda-sorta
Something between an array and an object
interesting
or
(short a, short b) = ...
that's make sense. but I realized I have to use
var
also if all variables are same type
working in this way, but not working if I put short out, doesn't it understand that I want both short maybeShow the code that doesn't work
Because if you're using tuples, tuple deconstruction can't not work
Well, yeah
Because it's not a
short
It's (short, short)
that's ok
but
why at this point not requiring
Sure, you can
But why
(var foo, var bar)
if you can var (foo, bar)
I don't und why the compiler in this case understand that both are var, and it doesn't when I put short
var
is not a type
var
is "go figure out what type it is"
It doesn't understand that "both are var"ok, but is intended to take the place of a type
It understands that it should figure out the types
I guess you could say
var
is special in this case. If you have (string, int, bool)
you can't deconstruct it to a variable of any concrete type
You need var (a, b, c)
or (string a, int b, bool c)
or (var a, var b, var c)
Sure, in your case all elements of the tuple are of the same type
But handling this special case is just not worth it
So the compiler wasn't made to handle it
Since if you want explicit types you can do (T1 a, T2 b)
and if you want the compiler to figure it out you use var (a ,b)
wow
when I thought to have learned all bases 🤣
I imagine it was an hard work to write this compiler, or a compiler in general
Probably, yeah
We have a few people in the server who are on C#'s compiler team, actually
Might ask them about it if you're curious, #roslyn sounds like it might be a good place