how can I use createEffect so it doesn't rerun when certain signals change
GitHub
test/snake.tsx at main · nikitavoloboev/test
Trying new things. Contribute to nikitavoloboev/test development by creating an account on GitHub.
15 Replies
@lexlohr shared this code
but I am confused still how to apply it
SolidJS
Solid is a purely reactive library. It was designed from the ground up with a reactive core. It's influenced by reactive principles developed by previous libraries.
i checked docs for createEffect api
so I have this now
You can use ev instead of event()
ok I think it works
thank you
got some bugs with logic
but at least it doesn't rerun many times
kind of shame that
on
is not documented as part of createEffect
it seems its a common case at least in react to attach effects to only certain valuesI would also refactor this:
It is documented in on.
breaks sadly
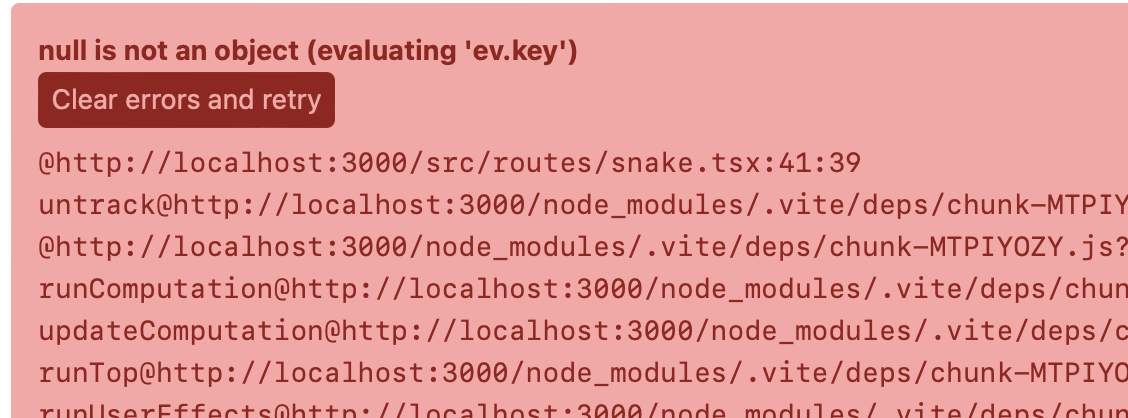
I still need to wrap
ev
to check it exists
so this works
kind of ugly but I guess neededOr you filter event right where you get it.
yea was going to say
but didn't want to bother you more
your solution didn't work
if (ev)
at top works
or rather nvmOr use createMemo to create a derived event:
I'm not bothered.
yea its ok
thank you ❤️
I did not follow the discussion super closely, but If you want to prevent effects from running on certain signals, use untrack.
Or
on
, which tracks only the reactive arguments and ignores the whole rest.
Since he uses grid and snake, but only needs to track event, on
was simpler.