❔ OOP Product Inventory Project
Hi I'm making a product inventory project to get better at OOP, and I have another class called Inventory where I have a method that calculates the value of the entire inventory. I'm trying to figure out how I would go about doing this, and so far my logic is whenever a new product is initialized, I want to add the quantity of that product and price of the product to a list so I can access that list in the Inventory class and then go about calculating the sum. My question is: for the constructor, when I initialize a new product in my Program.cs file, will it add the desired information to the list? I'm not sure if I'm doing it right.
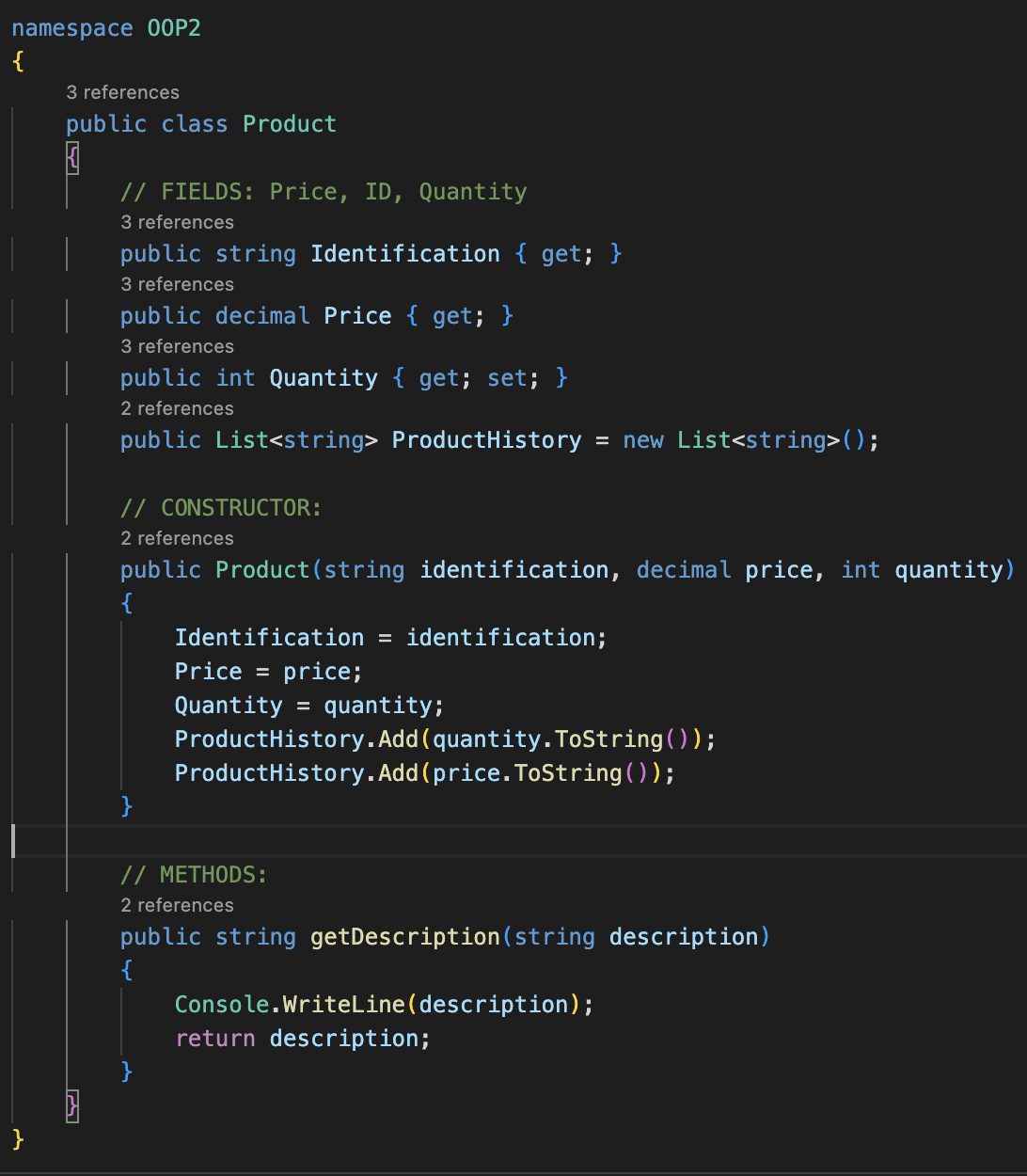
48 Replies
Product Inventory Project - Create an application which manages an inventory of products. Create a product class which has a price, id, and quantity on hand. Then create an inventory class which keeps track of various products and can sum up the inventory value.
Uh
Price and quantity are already properties
You can already access them
oh so i don't even need a list in the first place
Yeah
jeez haha
i'll look into how to implement that then
i guess in this case it would be good to have a list just so i can have all the products and their properties as a history reference?
Loop over the products in the inventory, then add
product.Price * product.Quantity
to some total
Lastly, return the total
You mean, historical quantities and prices of the product?I mean everything about the product
price, ID, and quantity
Why?
To be able to compare that the product used to cost 7.99 and now it costs 8.78?
just so there is an option for the user to see everything at once
So.... not historical data
You just want to place properties, that are already public, inside of some public list... to do what, exactly?
ah now that you say it like that it doesn't make sense to do that
i just want to have a nice interface when the user runs the program
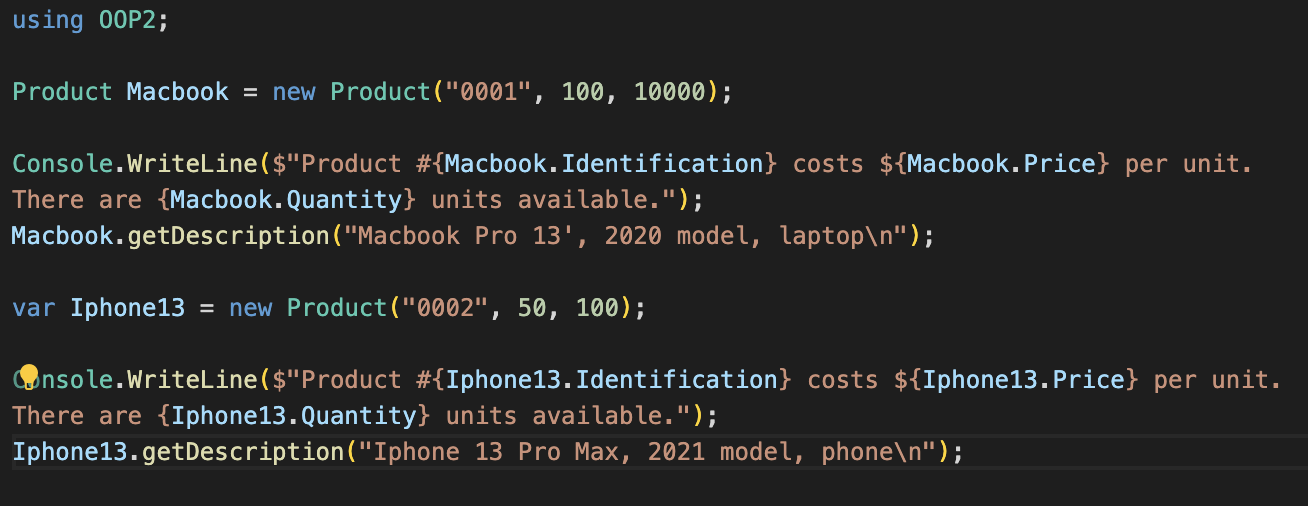
yeah this is what i have in my program.cs
Ah, you're storing each product in a separate variable?
But i feel like its redundant
Why not a list of products?
exactly
i think thats what i was trying to get at
i want to add the product and its information to a list
that has all the products
Then
to display all of them
perfect
Lists and loops
awesome gonna try and implement that now
thanks
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.could someone point me in the right direction on how to calculate the total sum of my inventory?
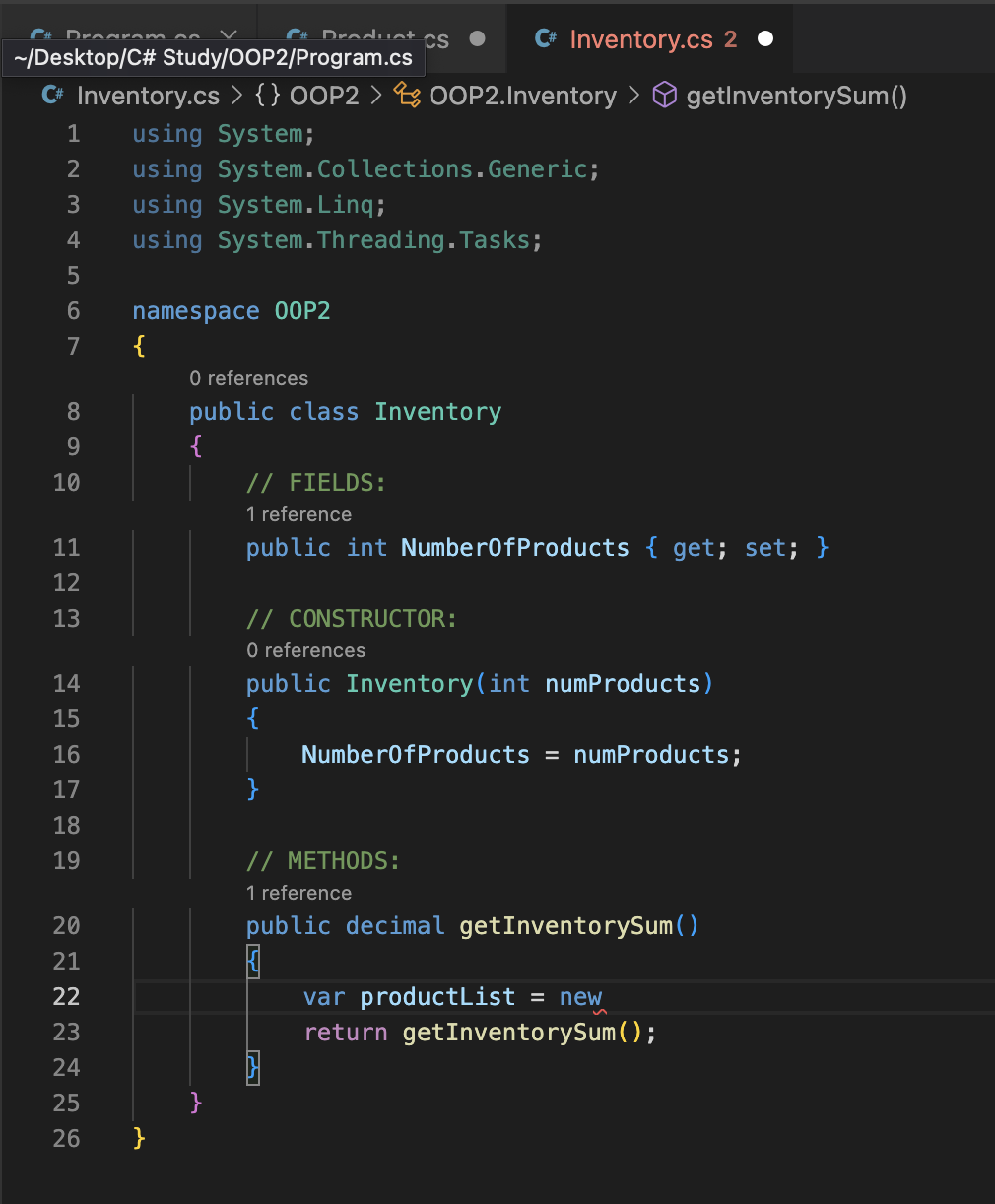
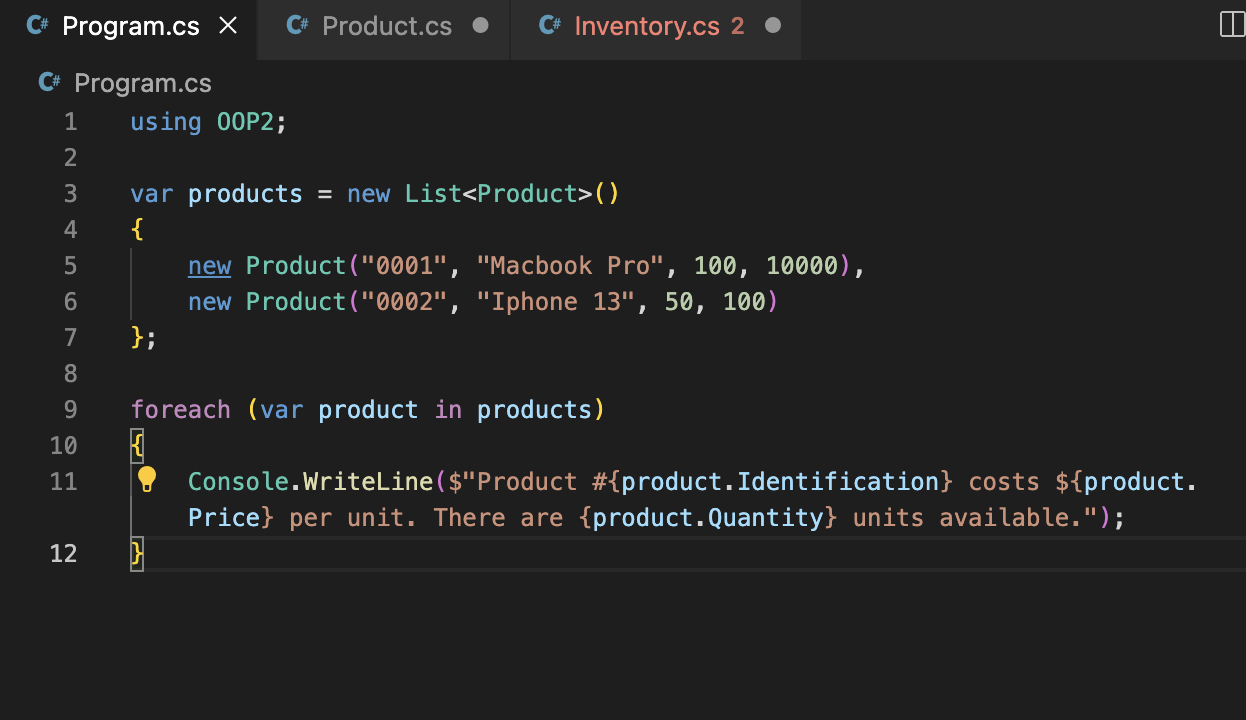
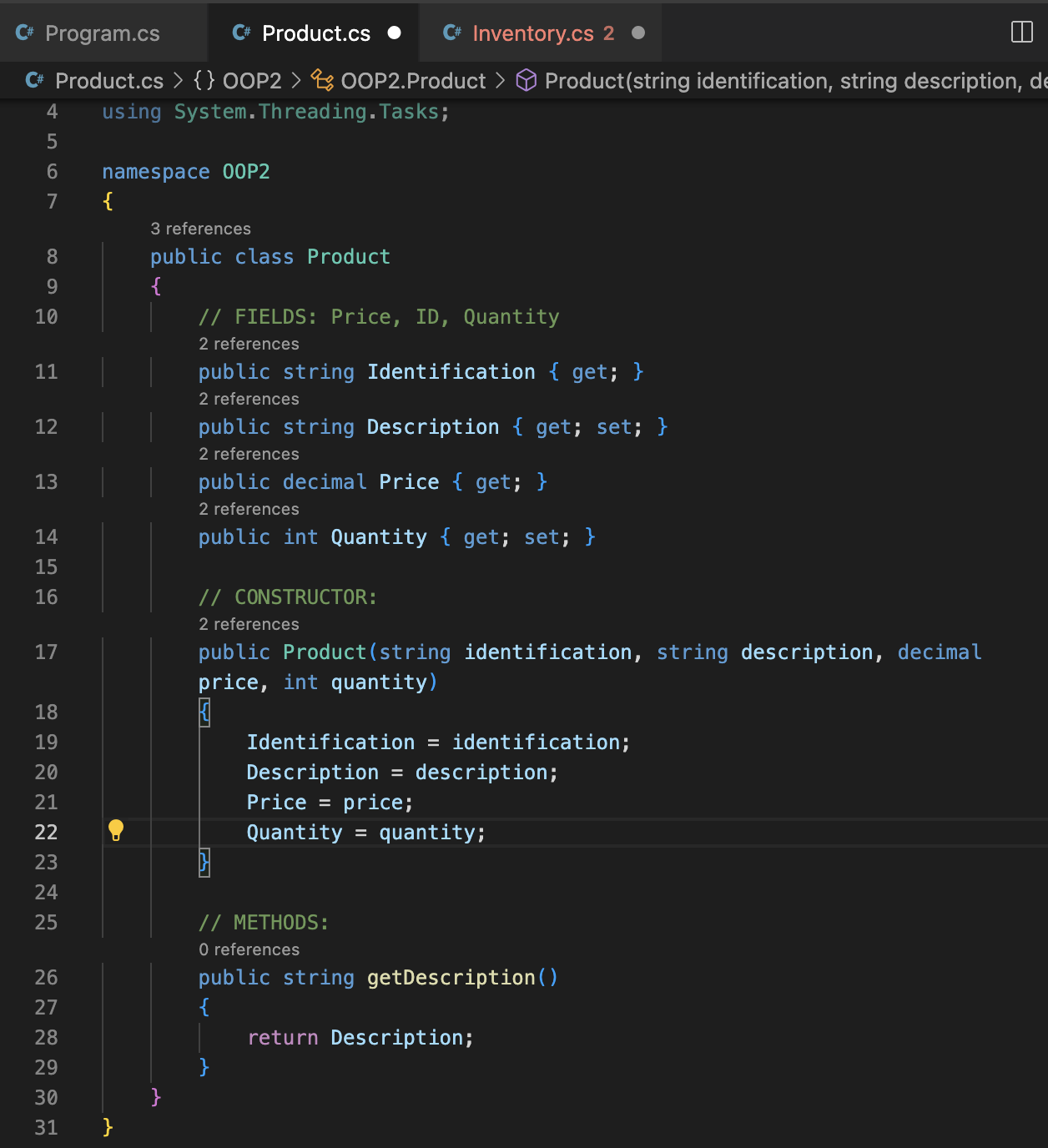
earlier in the post i was told to loop over each product in the inventory and then calculate the total fromthere
but im not sure how I would do that since I already initialize a list of the products in my program.cs
i tried looking into how to access a list from a different class in c# but i dont think it would work in this case?
https://stackoverflow.com/questions/3717028/access-list-from-another-class#:~:text=To%20make%20it%20accessible%20to,public%20property%20which%20exposes%20it.&text=To%20access%20the%20list%20from,MyList%20to%20access%20the%20list.
Stack Overflow
Access List from another class
can anyone tell me how to create a list in one class and access it from another?
Would i create a list in product.cs and then somehow add each initialization of a product object to that list?
Keep the list of products in the inventory
Not in the top level
this makes a lot more sense and i implemented it, but now i'm confused on how I would call this
Instantiate
Inventory
And work with that instance
Also, you never seem to use NumberOfProducts
yeah i was just gonna talk about that
i need to think on that one more actually
but yes exactly i forgot to instantiate it thanks
ok ive been struggling on how to implement NumberOfProducts in the code and i need some clarification please
could I just do something like this?
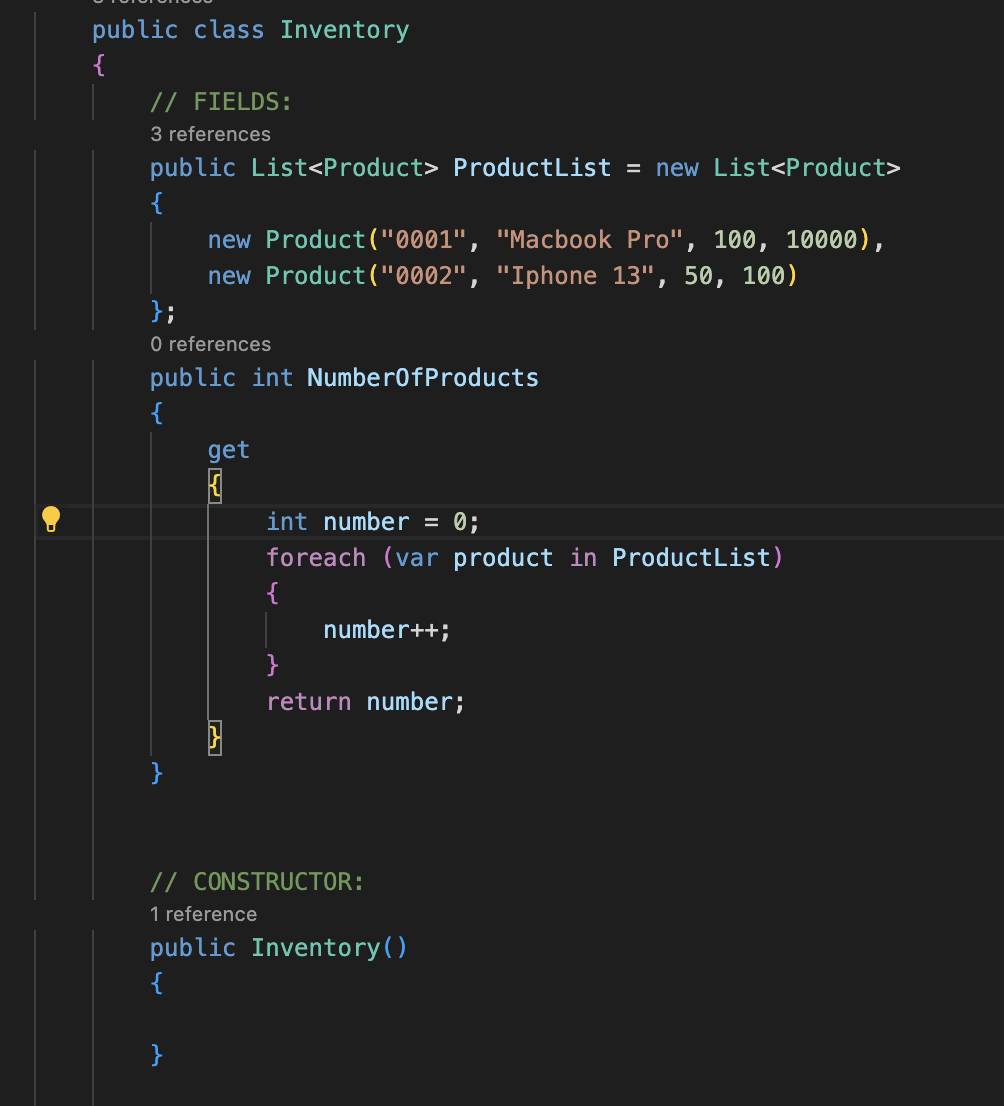
because from what i had originally i would have to manually say how many products there are and that doesn't really make sense
im also not sure how I would add the list information to the constructor, do I even need to do that in the first place?
ProductList.Length
List<T>
has a Length
propertyoooh ok i'll try and implement that
how would I put that into the constructor? and did you mean the count function?
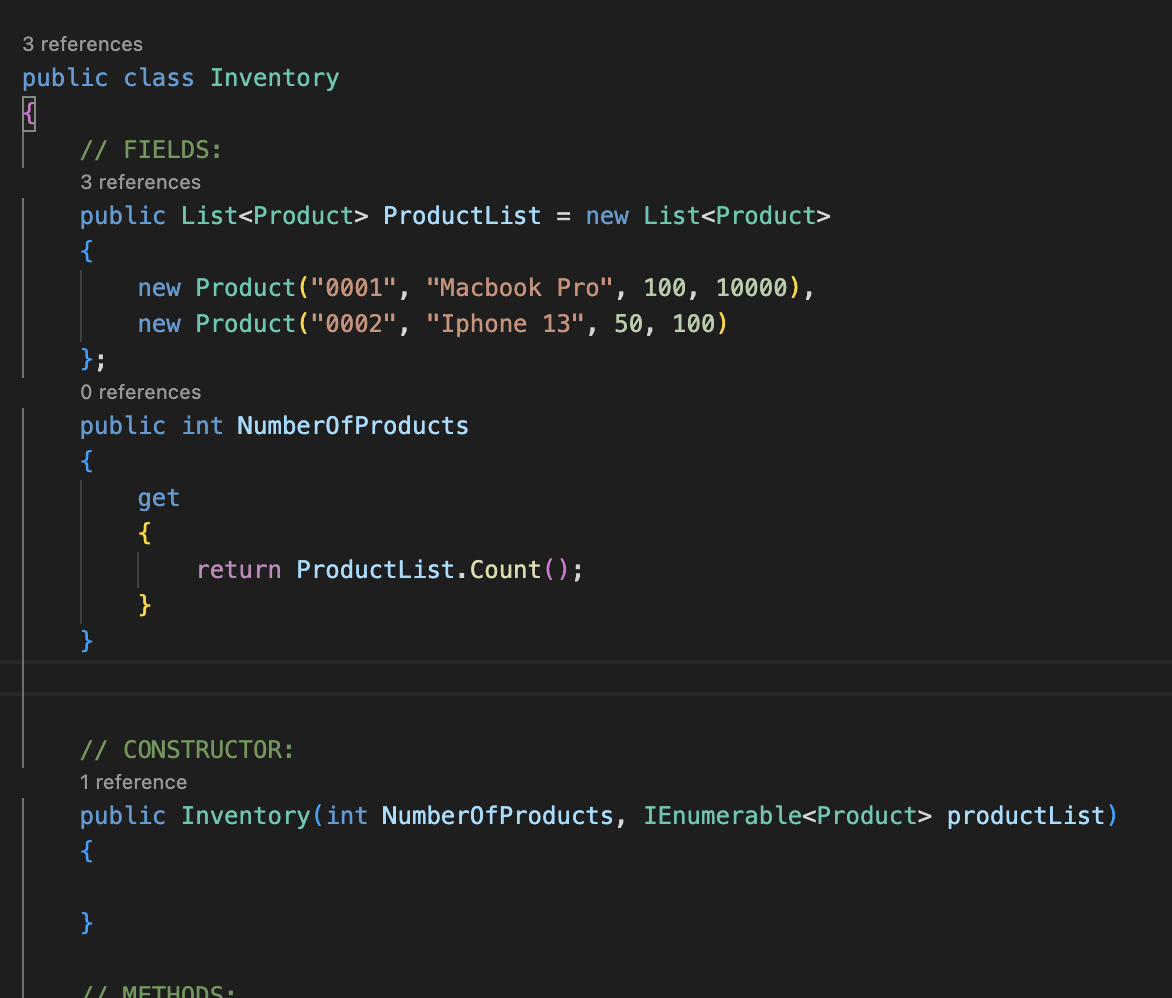
Why do you need it in the constructor?
to be honest im not even sure if i need a constructor
i thought you did for oop
Every class has at least a default constructor, that's what allows you to do
var f = new Foo();
But a constructor doesn't need to have any parametersoh ok
so i can just leave the constructor blank?
Or you can just not have it
A blank constructor is implicitly there
gotcha
yeah it works perfectly now 😄
well with the exception of number of products not being referenced
i'm thinking I should just make it a method, what do you think?
You can make a pass-through property
Or reference the list of items, and its
.Length
property, directly
inventory.ProductsList.Length
Or make a public int ProductCount => ProductsList.Length;
property in the Inventory
and use it with inventory.ProductCount
ok trying to process the info i'll try these out
i think i just like mentioning the property directly in the program.cs
but other than that, i think the program is finished
thanks as usual z, youre a real lifesaver
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.