❔ Ignoring Main?
just starting out with c# and have a pretty basic question. Trying to just make a random array of ints and print them out.
output:
53 Replies
First time I see anything like that... I guess it offers some strict checks
Like, in C# the naming convention is usually
PascalCase
, but your class has a lowercase
names
And, yeah, lowercase names are usually reserved for the language constructs like string
, int
, bool
, etc
The second one... could it be about your code missing a namespace?oh yes, if I change to
class Sorting
then it gets rid of one error but still
warning CS7022: The entry point of the program is global code; ignoring 'Sorting.Main(string[])' entry point.
let me tryAngius#1586
The second one... could it be about your code missing a namespace?
Quoted by
<@!85903769203642368> from #Ignoring Main? (click here)
React with ❌ to remove this embed.
Visual Studio supports writing code without a main or classes. Could do that if you're just looking to play around.
Oh I would like a main and classes. Just trying to get this setup before getting more into it
moving over from Java, but just not understanding what the issue is here
is this all of the code?
or is there something above what you are showing
this is all of it
No other file in the project?
Besides the
.csproj
of courseI'm struggling to reproduce that with just the code you shared.
two empty .cs files
Is one named
Program.cs
by chance?no
huh
What are they named?
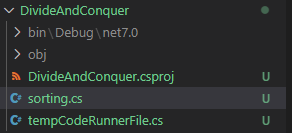
Ahh you're in Visual Studio Code rather than Visual Studio
yes
I just went to dotnetfiddle (online ide) and it runs like I want it to
How did you create this project?
dotnet new console
?yes
not even a
using System
?No need
Global usings since .NET 6
even with that it doesn't work
Uhhhhh... A long shot, but rename the
Sorting
class to Program
, and the sorting.cs
file to Program.cs
...?
It shouldn't matter, since only the Main
matters for the entry point, but who knowssame thing
so this seems like it might be a vs code thing?
Shouldn't be
put an explicit entry point in the csproj...
like
<StartupObject>Name.Sorting.Main</StartupObject>
(if i remember correctly)When you run
dotnet build
is the warning displayed under "Build succeeded"?Or just yeet the main and use top-level statements lol
warning CS7022: The entry point of the program is global code; ignoring 'Program.Main(string[])' entry point.
Weeeeird
Aight, time to experiment
this is as proper of a code as it can be, with as few moving parts as it can have
No namespace, no class, no main
If that doesn't work, I'm at a loss
error CS8802: Only one compilation unit can have top-level statements.
cursed pc
Delete all the other files you have that are empty
omg
Leave just
Program.cs
with this code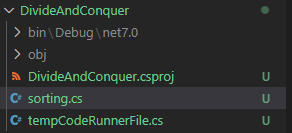
it was that tempCodeRunnerFile.cs
i didnt make that
Oh, so deleting that fixed it?
I was about to come onto that :p
thank yall 🙂
Woohoo
What was in it?
If the file was completely empty, chances are the compiler saw it as a top-level statements entry point
its an extension I have on vscode I guess it does that for some reason
Even if I try adding that file, I can't reproduce it
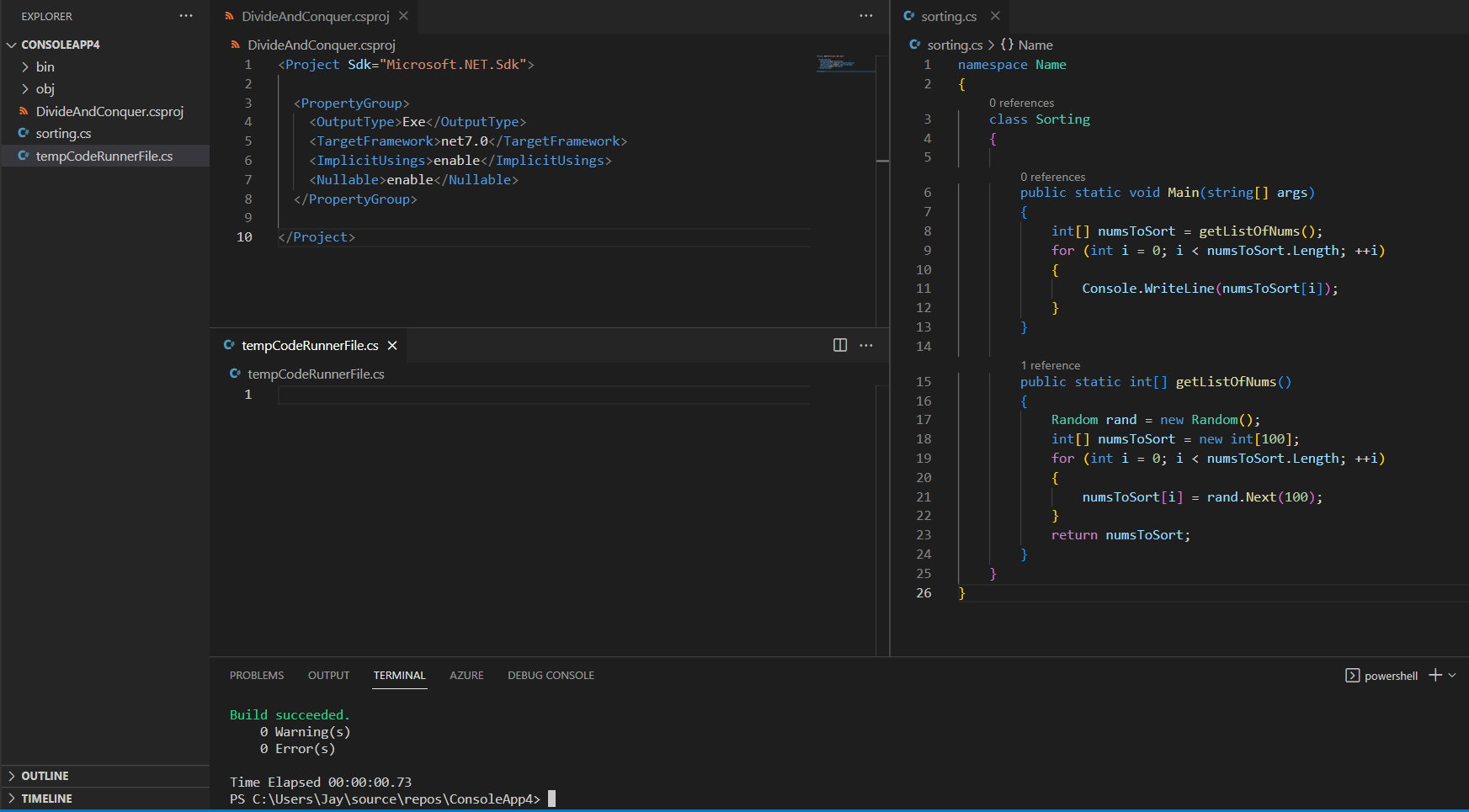
I am not sure why 😄
Oooh
Woohoo! Adding that code reproduces it.
That makes sense
You have one file with classes and one that's just standalone code. Feels weird to me to have it like that.
thank yall for the help, I was starting to go crazy lol
It doesn't know whether to run the Main or the standalone code
No worries
Indirectly I learned about having just top level statements so that is nice
TLS are great
I didn't know they were called "top-level statements" so I learned a thing.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.