❔ ✅ how to sort numbers in list without using funcitons
how can i sort numbers in a list without using reverse?
154 Replies
without using functions?
why?
yea
thats the requirment
i thought of how to do it in python but in c# i just cant
oh its an assignment
yea
so they want you to implement a sorting algorithm?
yes i wanted to mb check which number is higher or lower and doing it like that
they didnt say which algorithm to implement?
no just sort without functions
And what do you have so far?
creating list by the user and i want to sort this list
i searched it online
but couldnt find it
Look up "bubble sort", it's one of the more basic sorting algorithms
like sorting numbers
i only found sorting with lengths of words
Lengths of words are... numbers
just take the "length of words" as ur numbers 😉
wait
there are dozens of algorithms for sorting. the simplest would be a nested for that goes through every element and swaps them if one is less/greater
Bubble sort
Bubble sort, sometimes referred to as sinking sort, is a simple sorting algorithm that repeatedly steps through the input list element by element, comparing the current element with the one after it, swapping their values if needed. These passes through the list are repeated until no swaps had to be performed during a pass, meaning that the list...
but how can u make it so it checks eachother
well u have a
List<int>
i assume, right?yea
so with
yourList[index]
u can access each element in the list if u provide the corresponding index, like yourList[0]
will give you access to the first elementthe list was created with inputs and than adding them so i dont know how many elements are there
thats why the list has a
Count
property, its giving u the amount of elements stored in that list
so yourList[yourList.Count - 1]
would be the last element in ur list
(the - 1
is needed because indices are starting at zero)but what about the middle part
by "middle part" u mean the elements between first and last?
yea
ah okay: u dont write that down manually.
do u know about
for
loops yet?yea
this is how i made the list
List<int> Numbers = new List<int>();
Console.WriteLine("how many numbers do you want to input:");
int num = Convert.ToInt32(Console.ReadLine());
for (int i = 0; i < num; i++) { Console.WriteLine("input number "); int Input_Num = Convert.ToInt32(Console.ReadLine()); Numbers.Add(Input_Num); }
for (int i = 0; i < num; i++) { Console.WriteLine("input number "); int Input_Num = Convert.ToInt32(Console.ReadLine()); Numbers.Add(Input_Num); }
okay, now think about how u would write each number from the
Numbers
list to the console in a for
loop
(just try it, if its wrong thats okay, ill explain what was wrong)
also $codeTo post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/$codegif
(for better readability)
oh ty
create seperate count =0
and create loop printing the elements
but adding +1 to count
show me some code ;p
wait
it didnt workk
lemme try
oen sec
try to ur hearts content 😉 when u r stuck ill help out
wait should i use foreach?
nope, i want u to use a
for
loop (like u did for reading the user input numbers)
this is essential for the sorting algorithmint Count = 0;
for (int i = 0; i < Numbers.Count; i++) ;
Console.WriteLine(Numbers.Count);
Count++;
couldnt find that on my keyboard sorry
above tab
oh
Numbers is the list
the semicolon after the for loop is a statement
no brackets means only do the following statement
oh yea
so that for loop will do nothing
ty
will that work for printing that element
btw
Count
is the same as i
so no need for itk changed that
btw an easier way print lists/enumerables is
imagine u entered 3 numbers, what would this print?
0
1
2
but thats not the point, its the first step to implement bubble sort
dont spoon feed them D:
oh i thought you were trying to print the list
welp you asked
oh thats different person
lol my bad
yeah, to help them understand whats going on 😉
ok ik whats wrong
i didnt print out the list
just the order or number of the list
0 1 2
not like 59 90 103
ESGO, try to turn that loop into printing the elements of the list instead of the indices
u will just have to adjust the line
Console.WriteLine(i);
correct ;p
so for now u have learned how to iterate over the elements by accessing them by index
now its about sorting that bunch of numbers, we dont look at the big picture yet, but only at the two first elements
Number[0]
and Number[1]
.
to sort them, u must compare them first, how would u do that?looping comparing first and second elements and than adding i+1?
dont think about the loop for now, but just
Number[0]
and Number[1]
(we will come back to the loop)kk
basically, if
Number[0]
is bigger than Number[1]
u would have to swap them. write that in code 😉if?
using if
yep
oh, i just noticed that i had typos, its
Numbers[...]
instead of Number[...]
about ur code:
the condition must be in brackets so its if (Numbers[i] > Numbers[i + 1])
yea forgot
i just wrote it here
Swap
isnt an existing method, u have to manually do it. so basically Numbers[i] = ...
wait its not?
dam
Numbers [i+1] = Numbers [i]
will that work
thats only a part of the solution.
u basically just set the value of the next element (
Numbers[i + 1]
) to the current value (Numbers[i]
)
so if u have a list [15, 1]
that would result in [15, 15]
, right?oh and do
Numbers [i] = Numbers [i+1]
but wouldnt it alr be set to 15?
like the example
yep
thats why u would need to store the value first in another variable
Numbers [i+1] = New_num
Numbers [i+1] = Numbers [i]
Numbers [i] = New_num
the first line would be incorrect
int
i am too used to python
ong
yeah, python is a bad starting language imo
lemme think of using this in a loop
for (int i = 0; i < Numbers.Count;)
and do i++
in for loop wont it work?
and i need to add another if statement
u mean
?
yea and adding another if statement
keep ur if statement idea in mind, there is a flaw in the current code:
dam
for (int i = 0; i < Numbers.Count; i++)
will do the code for each index/element of the list right?1 2 3 4 5 6
it will go to 1 to 2 than 2 and 3
wont it
so for the list
[15, 1]
, the i
will be 0
and 1
so lets assume we are at the last iteration of the loop, so i
will be 1
, basically the very last element of the list.i wont trust the loop suggestions anymore ig
but our code looks "forward" by
Numbers[i + 1]
an element that can not possibly exist
thats why we have to adjust the loop condition now
we dont want to iterate over all elements anymore, but over all but not the lastOOOh
so the
i < Numbers.Count
must be adjustedat the and of the list
it will break
i am dum
for (int i = 0; i < Numbers.Count - 1; i++)
u aint dumb, im leading ya to some flaws 😉
yes thats correct
les go
so thats the up to date code which runs smoothly
now second statement
now imagine u have a list
[15, 2, 1]
and think about what happens if u run that code
whats the end result of the list?one sec
2 1 15
correct
what if we make it so it checks it 2 times
i would phrase it differently: we sorted the first element at index
0
into its appropriate place
but that also means sorted only one element, now we have to do that for each element.
that means, we need a for loop, that executes the current code for each element
wait what if we do i = 1
and in if statments we do 0 index as i-1
then we start always at the second element
i-1?
the first for loop (the one with the ???) should loop over all elements
so at each iteration u are looking at one element.
the second loop then moves that element to its correct position
oh
i am dum
one sec
but currently, the inner loop always checks the first element, ignoring the outer loop
waht if we do the same loop
after that loop
finishes
it should work
can u show what code u have in mind?
got erros
its incorrect
like if we run the same loop twice
on the list
doing the same checks
show me the code ;p
and the errors
for (int i = 0; i < Numbers.Count - 1; i++)
{
for (int i = 0; i < Numbers.Count - 1; i++)
{
if (Numbers[i] > Numbers[i + 1])
{
int temp = Numbers[i + 1];
Numbers[i + 1] = Numbers[i];
Numbers[i] temp;
}
}
}
didnt work
same thing

in the outer for loop, rename the variable
i
to something else (eg k
)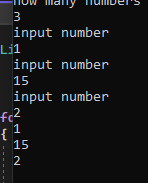

Numbers[i] temp;
needs to be Numbers[i] = temp;
(sorry thats my fault, my keyboard is a bit broken)i swear i did that it was the same error
dam
ty
lemme try
doesnt print the last output
like the l;ist
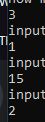
oh
i am so dumb
i forgot to write to print out the list
;p
cap5lut#0057
REPL Result: Success
Console Output
Compile: 539.171ms | Execution: 60.722ms | React with ❌ to remove this embed.
i forgot
LESE GOOOO

fuk
i should have just printed it out myself
show me ur code
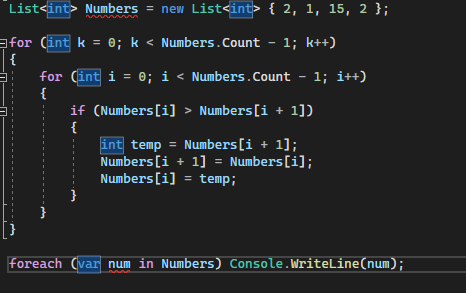
List<int> Numbers = new List<int> { 2, 1, 15, 2 };
<-- that line was just to fake user input.
that part is already done by u (and above the code u have shown)i didnt even look at it
i am
retar
Sorry @cap5lut your message contained blocked content and has been removed!

can u show the whole code? its simply about variable names i guess
\
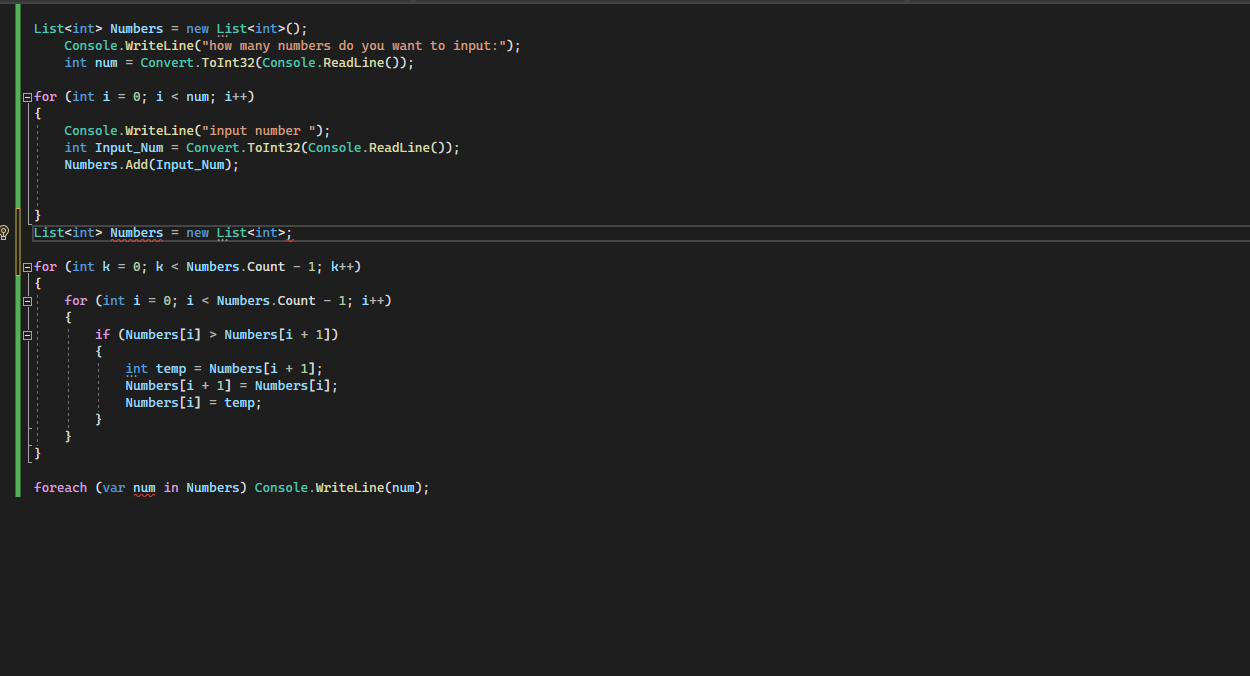
and u are neither dumb or retar..., u r learning something new, so its okay to make some errors 😉
bro i swear ty ily like i wasted so much of your time
that
List<int> Numbers = ...
between the reading user input and sorting for-loops should be removedi am blind
ty
as said, that was to fake the user input which u have done already

in the last
foreach
loop, the num
contradicts with the num
of ur user input (how many numbers should be entered)
so u can just rename it for the foreach
loopoh yea
i am blind
its basically complaining that a
num
variable already existsyea saw it i like checked num and didnt saw the other num change colours
ty
like
u spent so much time on me
ily
sorry for your time
and thank u
i love myself too ;p
U SHOULD
so, before we stop here now:
foreach (var num in Numbers) Console.WriteLine(num);
<- dont do it like that, i was just lazy typing.
u should always use curly braces
spot the huge difference on what it is doing:
assuming there are 10 numbers in Numbers
, one will print "some text" 10 times and the other only oncei always forget those
last task i was stuck 30 mins bc of it
it wouldnt run normally
f python
well, u come from python, so its sorta understandable ;p
yea
shi to easy there
ty again
i learned alot
dont get me wrong, python has its benefits too, but for a beginner its simply the wrong language
so do u have any left over questions regarding this topic?
if not: $close
Use the
/close
command to mark a forum thread as answered(and im glad i could help :3)
$close
Use the
/close
command to mark a forum thread as answeredty
btw
theres no wrong language
yeah i should have added "in my opinion". imo having duck typing languages like python, js, etc shouldnt be the first language to learn, a statically typed language is imo the better choice
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.