❔ Help with a bool not working
Guys I feel like I'm going crazy. I'm making this Password Validator and for some reason the "no T" rule doesn't work (yes it's a very specific rule, it's a test in a book).
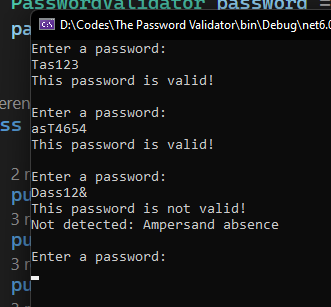
31 Replies
the "no &" is the same thing but it works
aTy
password
a
-> sets hasT
to false
T
-> sets hasT
to true
y
-> sets hasT
to false
Angius#1586
REPL Result: Success
Console Output
Compile: 574.017ms | Execution: 47.629ms | React with ❌ to remove this embed.
You'd be better off using
string.Contains()
Angius#1586
REPL Result: Success
Result: bool
Compile: 405.034ms | Execution: 24.659ms | React with ❌ to remove this embed.
but I never make HasT true again after it becomes false
there isn't a code for making it true
and I'm supposed to use char.IsStuff for the test
Ah, huh, you're right
My bad
That's ok
If that were the case the & rule wouldn't work as well
I guess fire up the debugger
That's what's driving me mad, they are pratically the same thing
I didn't get to this part of the book I'm studying
$debug
Tutorial: Debug C# code - Visual Studio (Windows)
Learn features of the Visual Studio debugger and how to start the debugger, step through code, and inspect data in a C# application.
no idea what a debugger does lol
huh thanks
will take a look
Lets you take a look at the values of variables during the runtime of the program
You can pause the execution, and then execute the code line by line, all the while seeing what value each variable has
oh, that's awesome
Ah
I see what's going on
You print
T absence
when HasT
is true
So you print the error about T not being there... if the T is there
Your &&
set works, because you negate it
&& !HasT
-> "and doesn't have T"yes, it's not supposed to have a T
the password should fail if it has a T
but it doesn't
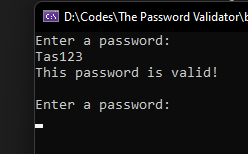
and this part is preceded by a Not detected: haha
Wait, I got myself confused lol
so it should print Not detected: T absence
yeah the code is a bit messy, sorry
Ah
I know
its so I can see what went wrong
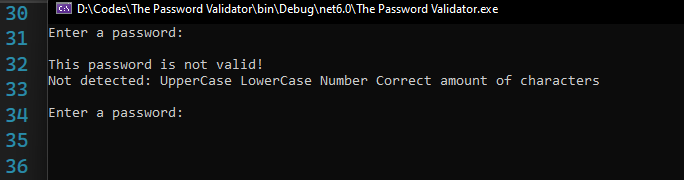
It's a set of if/else if statements
Only one of them will execute
T
is uppercaseOMG
So the code hits
if (char.IsUpper(letter)) HasUpper = true;
and peaces outTHAT'S IT
IT'S WORKING
thank you so much
Nice
nice eye lol, would have taken me a week to get this
ok, I can move on now
Thanks a lot! @Angius
Have a great day
Likewise 👌
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.