❔ Problem with image hosting API
Hello,
I tried to upload an image to an image hosting API but no matter what I do I get the following error :
System.Net.WebException: 'The remote server returned an error: (400) Bad Request.' (see screenshot).
Code :
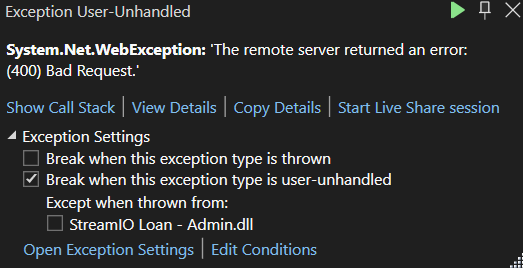
62 Replies
First and foremost, use
HttpClient
not WebClient
. The latter is obsolete and should not be used
And the API wants a POST
request with form-data content
Not data sent as a query stringThank you I'm gonna change webclient to httpclient, but I don't get the part about the POST request (sry beginner here)
HTTP requests can have different types and send data in various ways
GET
is for getting, POST
is for sending, PATCH
for updating, etc
The data can be sent as a query string like ?foo=1&bar=hello
, which is used for GET
requests
There are other ways, like multipart/form-data
that supports sending files
Or application/json
that lets you send structured dataThe API docs say

okay uhh sorry just got back, imma try to understand it all
yeah I don't get it at all lol
I have no clue where to start or even what to do with my code
tl;dr is, you need to send a
POST
request with multipart/form-data
dataMAKOLYTE
C# - How to send a file with HttpClient | MAKOLYTE
Shows how to send a file in a multipart/form-data request using HttpClient by loading it into a MultipartFormDataContent object
Like in this code, except you'd add one more field to the formdata
The field with your API key
tyvm I'll try to make it work somehow <:br_laugh:742854844301312031>
Yeah, feel free to ask again if the code still doesn't want to work
I still can’t get it to work lol I have no clue how any of this works, I’ll just get some sleep and watch a few tutorials tomorrow I guess
Thanks a lot btw
No problem, anytime 👌
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.Still not fixed no I feel like this is way above my very beginner level lol
Show your current code
We'll see what's up
I deleted most of it to try and start from the beginning, but my problem is with this :
https://makolyte.com/csharp-how-to-send-a-file-with-httpclient/
In this example, he sends a file from a predefined path, which is not what I want, I want to send an image that the user chooses from his computer, and secondly, he sends it to a server while I am trying to just use an image hosting API, which I don't know how to change for my usage
MAKOLYTE
C# - How to send a file with HttpClient | MAKOLYTE
Shows how to send a file in a multipart/form-data request using HttpClient by loading it into a MultipartFormDataContent object
I'll post code when I make some progress lol
I want, I want to send an image that the user chooses from his computerSo get the path to the file from the user
he sends it to a server while I am trying to just use an image hosting APIWhere do you believe does the API run?
localhost:6001
and https://mycoolwebsite.com
are the same thing, fundamentallyyeah lol I know it's from a server but he just hosts it to some localhost link and not using an API with the key etc so idk how to proceed
Is there any way to get a file path automatically from a file selection dialog ?
That's actually what the file dialog gives you, the path
And the link to localhost is no different than the link to the API
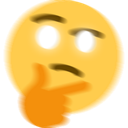
Literally the same exact thing
yeah I know but where does the key go then
Into the form data
Angius#1586
Like in this code, except you'd add one more field to the formdata
Quoted by
<@!85903769203642368> from #❔ Problem with image hosting API (click here)
React with ❌ to remove this embed.
okay thanks Imma try to understand it all because even syntax is complicated to me lol i'll get back to you, tyvm
hey uh I'm confused about something
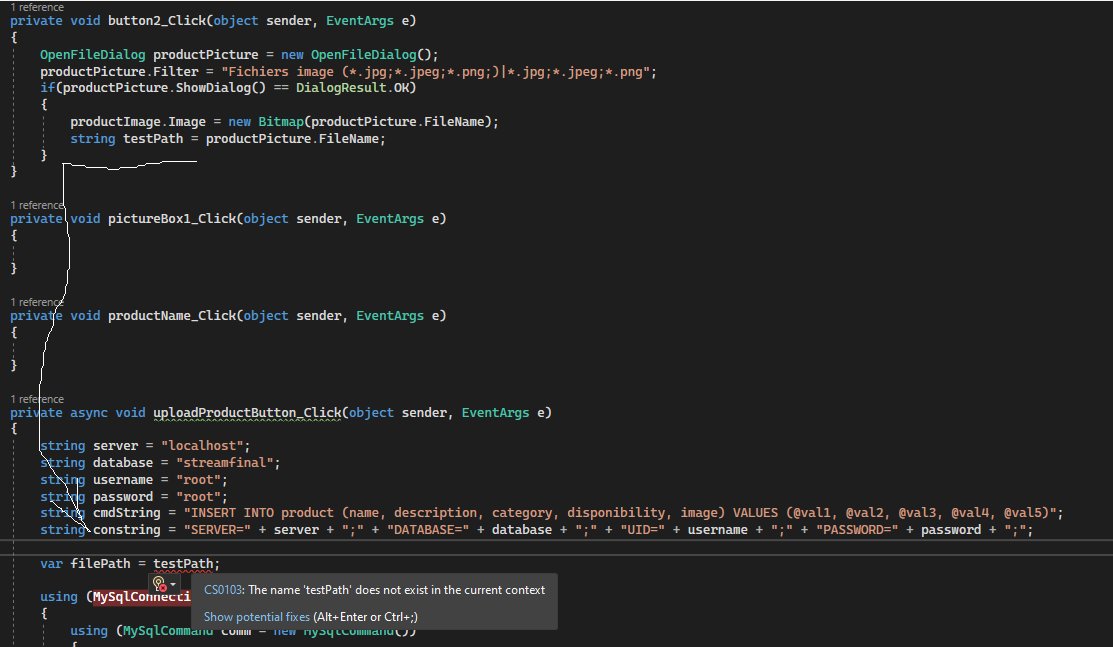
Shoot
$scopes
thing a
is available in scope A
and scope B
thing b
is available only in scope B
how do I access this variable if it's in a private class ? I'm pretty sure I can't but it's private by default and I4m not sure changing it is a good idea
Just place it on the class level
very confused as in how to do that here
Just put the variable on the class level
but if I do that it won't recognize productPicture.Filename; anymore
private string filePath
on the class levelohhhhhhhh
filePath = productPicture.Filename
in one method
var filePath = filePath
in the other
Or just... use the class-level filePath
I thought I had it but no, I'm dense I think
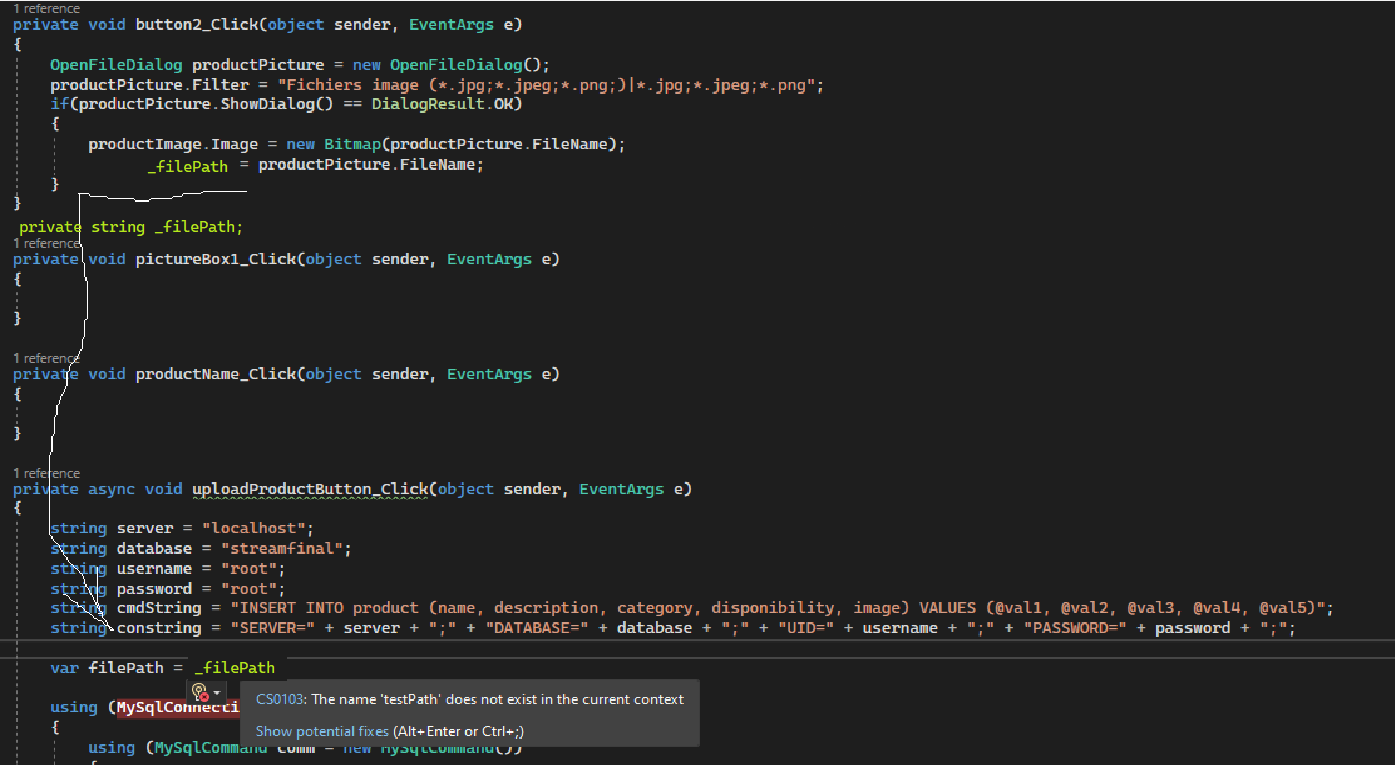
See my edits in lime green
woah it works tyvm, just trying to understand, what's the "_" for ?
Just a naming convention
Private fields have the
_
in front
Mostly for use in the constructors
With _
Without _
okay I think I get it thanks 🙂
so in the page you sent me to send the image to an API, regarding this section :
I'm not sure how to proceed since "house.png" has to be the selected file name here, and that "image/png" should be "image/whatever the chosen image format is"
woops not sure why it quoted the c# part too
$codegif
You need
cs
not c#
And you can get the MIME type from the extensionAngius#1586
REPL Result: Success
Result: string
Compile: 510.352ms | Execution: 41.514ms | React with ❌ to remove this embed.
okay that actually is cool, and for once I understand lol thanks
one more thing before I go to sleep and do the rest tommorow
how do I get the chosen file name, since "productPicture.FileName" actually returns the picture's whole path and not just its name ?
You can split by the path separator and take the last chunk
Or you can use
Uri
class, I believe
Or maybe the Path
class
One of the two
The constructor will take the whole path, and then you'll be able to take whatever from the objectso if the full path is :
C:\Users\secret\OneDrive\Images\secret.png
how do I split it so it always takes what comes after the last "" ?
*the last " \ "
Angius#1586
REPL Result: Success
Result: string
Compile: 470.377ms | Execution: 35.245ms | React with ❌ to remove this embed.
Like this
Kaedrick#5670
REPL Result: Success
Result: string
Compile: 447.716ms | Execution: 36.233ms | React with ❌ to remove this embed.
ok it works even if there's less " \ " in the path
perfect
tyvm!
With
^
you can index from the endohhh
tyvm!!
can you explain why there's 2 \ ?
just to be sure i understand
in (' \ ')
ffs
it won't let me lol
\
is the escape character
So '\'
is treated as "character delimiter, then literal apostrophe"
A closing character delimiter is, thus, missing
'\\'
is "character delimiter, literal backslash, character delimiter"oh okay! thank you so much you were so helpful I feel like maybe tomorrow or the day after I'll have this damn API upload thing working <:gx_Sunglasses_cry:750469526667264051>
but for now, some sleep
good night sir
'Night
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.