Parent doesn't update view when state changes.
Problem: when the child component modifies the state of
Auth
, the rendering doesn't update.
Auth component:
Login component:
15 Replies
Early return issue. Either wrap the ternary in {} or even better use <Show>
Longer explanation: solid.js runs components only once. You have effects, memos and JSX expressions (basically everything between {}) to opt in to reactive handling. Everything else will stay unchanged.
So using
return c ? 'a' : 'b'
will never update, regardless of state changes. This is by design; it allows solid to achieve top performance by not doing anything unnecessary.I see. I currently have this and now the state is true even when the button is clicked
Oh, I overlooked that. You return the function in the event handler instead of calling it.
<button onClick={toggleState}
Instead of () => toggleState.
did not change the behaviour, the state remains as true 🤔
Ah, missed the () behind the event. Stupid mobile formatting.
Can you show me your current code?
Specifically that of the login component.
Thanks
and auth:
Ah, I can't believe I missed that: destrucuring of props. This breaks reactivity.
Try using props.viewState instead.
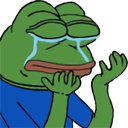
Login(props:any)
, props.viewState(false);
Strange, it works for me: https://playground.solidjs.com/anonymous/3bb85b94-2a69-4183-ab1a-f9ba04b7906e
Solid Playground
Quickly discover what the solid compiler will generate from your JSX template

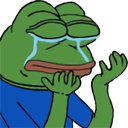
Use a function to wrap the ternary.
This works: ✅
That's even more idiomatic 🙏