❔ Help compressing image!
I need help compressing a jpeg that comes in as a base64string. It currently gets compressed via gzip but this is wrong! I want to shrink the image down therefore lowering the res:
18 Replies
You'll need something like ImageSharp to resize the image
Compression is not resizing
Thanks for responding! How would I implement it? I currently have:
but outstream is giving me an error
What error?
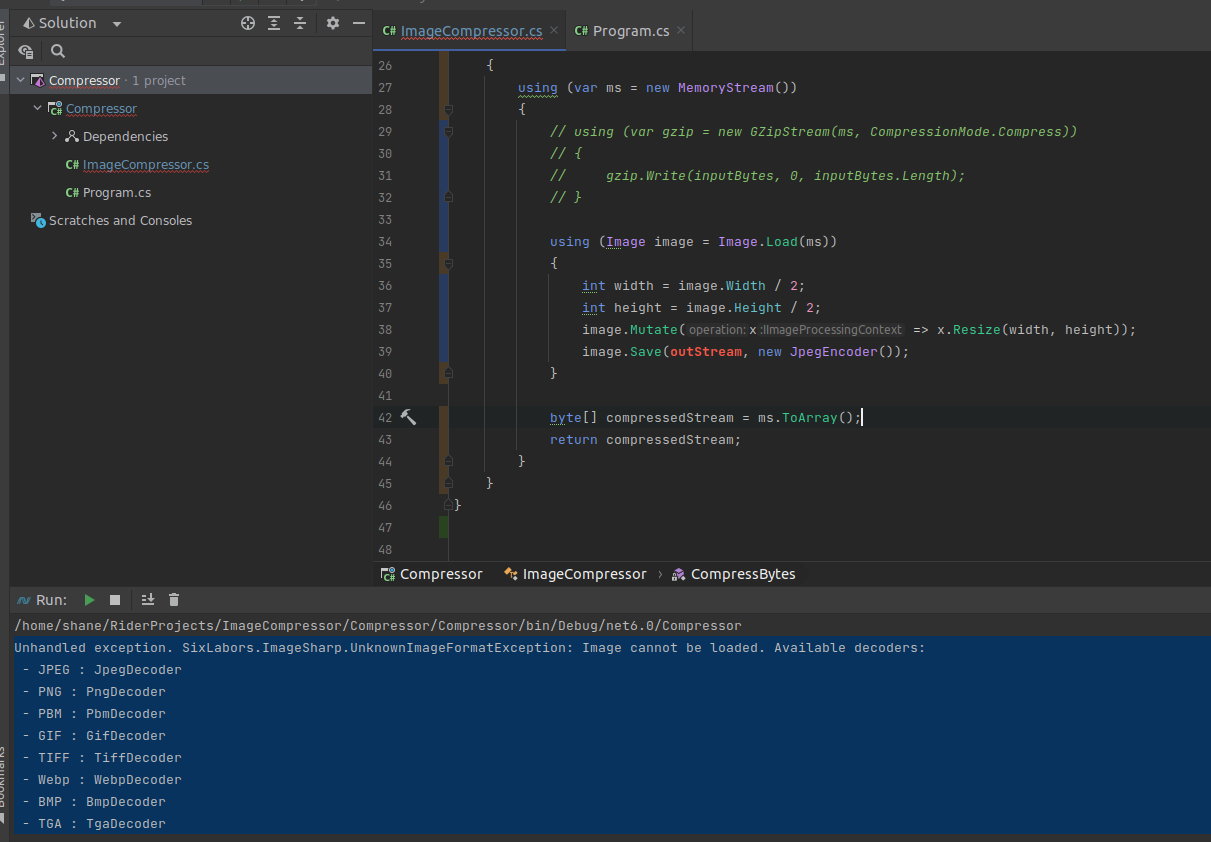
Doesn't seem like you have defined
outstream
anywhere
Can't use something that doesn't existoh! What is outstream suppose to be?I found the example here:
https://docs.sixlabors.com/articles/imagesharp/resize.html
Hover over
Save
with your mouse and see what the docs say
Probably a MemoryStream
or FileStream
ah
well I am already using the memory stream so should it be:
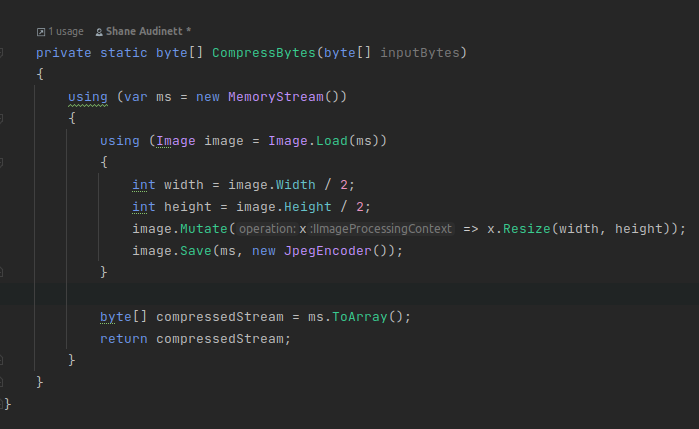
Sure, give it a shot
ah failed haha
Image cannot be loaded
ah ok. Ive changed the Image.Load() to the inputbytes as obviously it needs to receive the input
but the error is now:
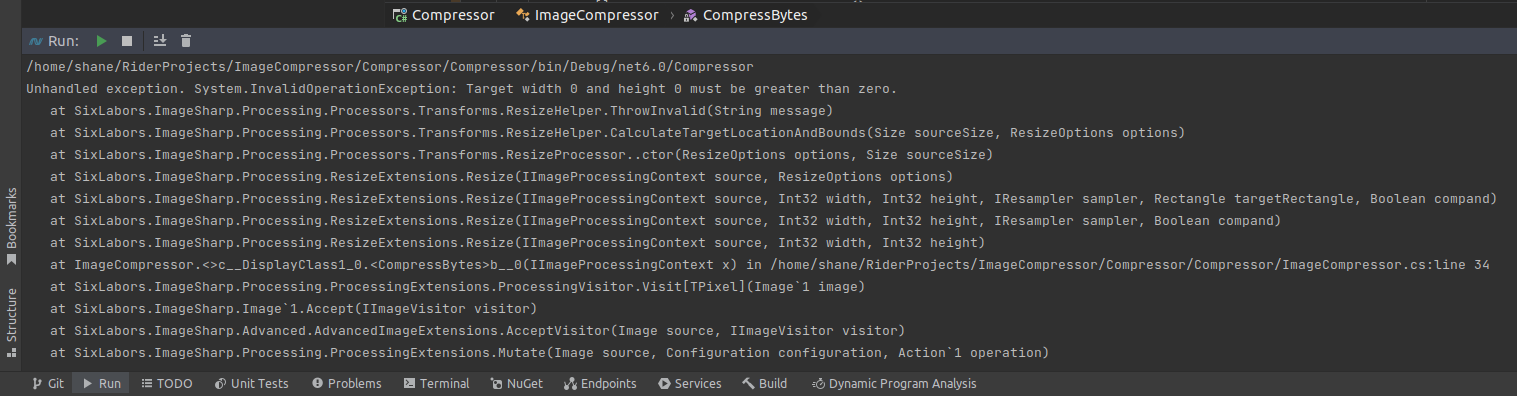
@Angius
Seems like the width and/or height divided by 2 give you 0
yes, seems this way
should I not divide it by two then?
1.5?
Idk what the original size is
oh crap I think I did it
Ill send it one sec
I cna pass in the width and height directly
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.