✅ Need some help converting PHP code to CS
Hey, I think I wrote this right but I'm getting different results. Perhaps someone knows what I did wrong here?
I'm trying to convert a PHP function to a C# function. Here is what I wrote in C#. The original PHP snippets are the comments above.
Upon running this, I do not get the expected result of:
VrOlH8EFHZWQeyrP2on5MR7Q8QMC08ZnHfLv8UE54ZwL7CIJ6vKhT3EpdYriab.oA/yv9wP/yyWUHQC9.18y0$S$D
Instead, I get:
/..l1..F1..Q0..P...5...Q0..C0..n1..v0..50..L/..J...h1..p/..i0..o...v1../0..U1..9...y0
23 Replies
just in case, here is the original PHP function in full:
C# just has
Convert.ToBase64()
method if you need to encode stuff to base64I’ve attempted to use that, however whatever weird function drupal uses to hash it’s passwords outputs something completely different from a regular base64 conversion. Using
Convert.ToBase64
and phps built in Base64 encoder produce the exact same string on the input, but unfortunately I need the C# program to output the Base64 string that’s encoded through this methodWhy does Drupal use some weird base64 encoding to hash passwords instead of an established algorithm 💀
That's a security nightmare
Let me look into it, maybe they are using some existing algo, just named it weirdly
Drupal fucking sucks, which is why I’m trying to move away from it and just build everything from scratch haha
Only thing I found so far is this: https://www.drupal.org/forum/support/module-development-and-code-questions/2014-07-04/_password_base64_encode
Perhaps the comment at the top of the PHP function above might ring some bells for you? I’m completely lost when it comes to this stuff unfortunately. I appreciate your help
Ah yes the classic “just use something else” hahahah
Okay, so, apparently... Drupal uses some weird shit instead of the built-in
password_hash()
function of PHP
It returns bytes, that need to be encoded as base64
And this method is supposed to do that
Which... doesn't answer the original issue, but at least it's a clue to the weirdness lolYup I’ve successfully written the password hashing function in C#, and verified that it’s hashing the passwords correctly. The last piece of the puzzle is converting the hash byte array to the weird string it stores in the database
It also uses some
ord()
function to get integer value of a char...
vs
Let's make them more alikeord() function is a inbuilt function in PHP that returns the ASCII value of the first character of a string
Hmmmm
Wait does c# have the =| operator?
yeah
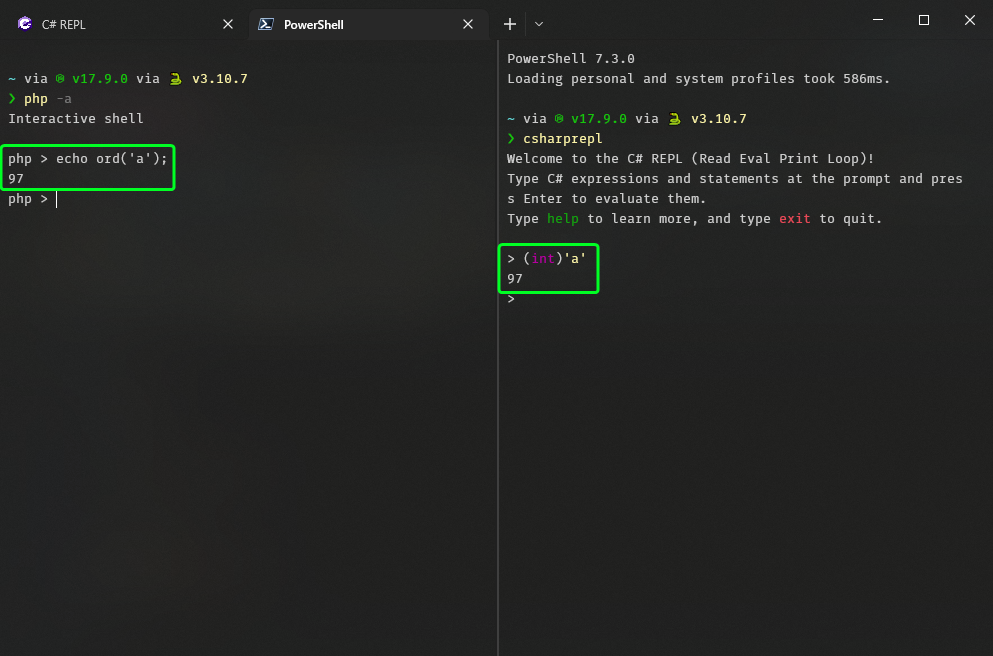
Aight, we know that
ord('a')
is equivalent to (int)'a'
So
can be
oh my god yup that did it
'Ere we go lol
thank you so much for your help!
Anytime
time to ditch drupal forever hahah
I heard newer versions aren't that bad
They're based on Symfony components, use Twig for templating, etc
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.