Double check if an object is one of provided types
Is there a way to quickly check that? I mean
a
can be either TextBox or CheckBox, doesn't matter which one, I want this condition to success if one of these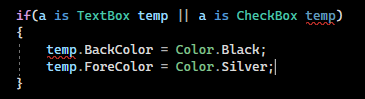
44 Replies
Here is where I use it
temp
cannot be one of two types
Algebraic data types are not a thing in C#Oh, right!
Or you could maybe do
a is { BackColor: _, ForeColor: _ }
But that won't allow for a cast eitherAs I check they don't have any common class that they derive from so I guess I'll have to create an if for each type
e.g.
if(a is Button temp2)
then if(a is MenuItem temp3)
and so on...This doesn't seem to work and this also not
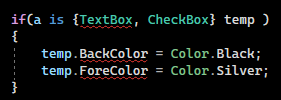
x is {A, B}
checks if x
is of type that has properties A
and B
Yeah but that's going to affect probably entire form as everything here derives from Control class (Windows Forms)
I rather wanted to specify to which particular classes I want to compare with
It fails, needs a cast probably
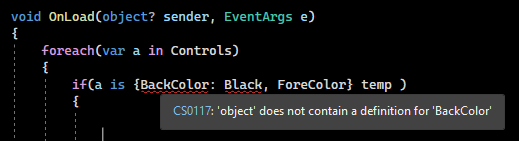
Just do
Easiest way without any nonsense
Yeah, this is the only option that comes to my mind but I didn't want that "naive" approach to copy paste bunch of lines that is the same inside each
if
statementYou could use a switch
That seemed like a great idea but also fails

I already have it
Btw,
OfType<Control>
and not Cast<Control>
What do you mean?
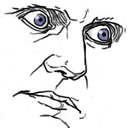
Yeah, these are the only options probably
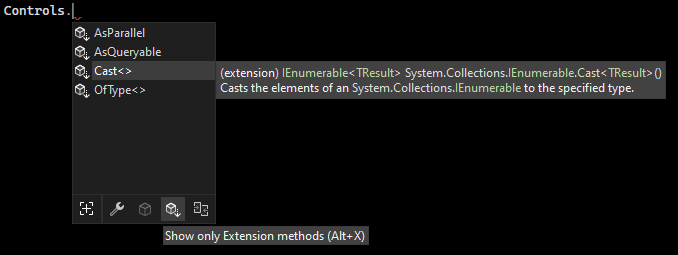
(OfType is slightly better than Cast)
Afaik it sometimes fails in weird places because it can't cast
OfType also makes sure everything actually is of the type, which is especially relevant with non-generic collections

thinker227#5176
REPL Result: Success
Result: ValueTuple<IEnumerable<string>, IEnumerable<string>>
Compile: 508.973ms | Execution: 35.374ms | React with ❌ to remove this embed.
Uhm... so there is no way to do that?
Why not just...
...?
Can't believe no one has suggested this...
It's a collection of controls nonetheless
Wow, great, that seems to be really working...
It doesn't need to be generic
But I want to distinguish only certain control types that all derive from Control
for example TextBox or CheckBox
You can then do
if (control is TextBox or CheckBox or ...)
and do your control.ForeColor
anywayAnd the original variable has type
object
@Ero so it is unknown what type it is
I tried that and I can't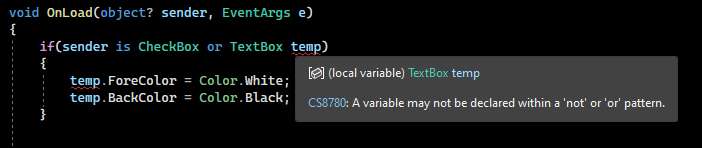
Hm that's also some solution 🤔 But what if an object is neither of Control type? 😄 The app might crash
Actually that didn't work in my case

That's why you specify the type in he loop head
But if I put there Controls I will not use sender variable at all, right?
It entirely depends on what you want this event to do
Nevermind the fact that this is a pretty bad way to set the styling for controls
You should be setting this in a resource dictionary and access the colors from there, at least for xaml based GUI frameworks
Let's say I have 30 text buttons in a form, how do I setup the same back and fore color for them all without doing that one by one?
I'm not sure if WinForms has a similar thing
By doing it one by one, the correct way
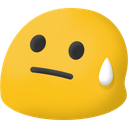
ive got no clue about winforms but i hope there is a way to use reusable styles, right? right???
That way allows you to add theming much easier
As in, light and dark themes
You bind the colors to a resource, and just change the resource
I think it exists but not for all controls
thank you all for your help I honestly didn't expect so much attention and how active will become here
I'll try playing with that, I guess there must be some way 😄

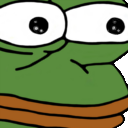
But yeah if you're planning on putting this event on multiple controls instead of putting a loop into it, then you don't have many choices i think
I thought about putting an event on the form to execute it after it loads
to find all controls of a particular type and change their colors

Or an array of types, then
types.Contains(sender.GetType())
...
But that's pretty ugly imo...Aight, so actually that code worked. And I did a mistake by trying to put that in some OnEvent method, the easiest way was to put that in the form constructor method and use Controls property so right after the initialization selected controls of the specified class (CheckBox, Button, TextBox in my example) received Fore and Back color as I specified.
Thank you @calledude if I saw correctly that was probably your idea do use 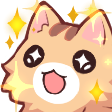
.Cast<Control>().Where()
Also thank you @Ero#1111@ACiDCA7#8219@thinker227#5176@Angius#1586
I can't ping you because I got timed out I haven't seen such engagement before 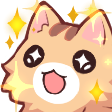