✅ runtime error
I made this algorithm that is supposed to receive an input and gather all the negative numbers into one variable and positive variables into another. in then divided it by the number of items in the given list.
73 Replies
This error was thrown however
for example
if given an input like this
It should print this
Use $tryparse
The TryParse pattern is considered best practice of parsing data from a string:
- a TryParse method returns
true
or false
to inform you if it succeeded or not, so you can use it directly in a condition,
- since C# 7 you can declare a variable that will be used as an out
argument inline in an argument list,
- it forces you to check if the out
argument contains valid data afterwards,
Avoid: Convert.ToInt32 — it's a bad choice for parsing an int
. It exists only for backwards compatibility reasons and should be considered last resort. (Note: Convert does contain useful conversion methods: To/FromBase64String
, To/FromHexString
, ToString(X value, int toBase)
, ToX(string? value, int fromBase)
)
Avoid: int.Parse — you have to use a try
/catch
statement to handle invalid input, which is a less clean solution.
Use int.TryParse https://docs.microsoft.com/en-us/dotnet/api/system.int32.tryparse?view=net-5.0#System_Int32_TryParse_System_String_System_Int32__ Int32.TryParse Method (System)
Converts the string representation of a number to its 32-bit signed integer equivalent. A return value indicates whether the operation succeeded.
Convert.ToWhatever()
throws
whatever.TryParse()
doesn't, just returns false
Also, run the debugger to see what exactly causes this erroralright
Some of the things you're trying to parse to an integer, isn't a valid integer
theres a couple things i should say
so
the main method wasnt written by me
i am solving a problem
and that's just how it was
im only editing the plusMinus method
Cool cool
The answer remains
wait then wdym dont use Int.Parse or Convert.ToInt32?

Line 46
That's where the issue is
thats line 46
uh
Yeah
Well
The result of appending those three formatted strings will never in a million years be a valid integer
It has dots, it has new lines, it has all sorts of things
What looks like
is as far removed from being an integer as something vaguely numeric can possibly be
yes
Why do you even want to parse it to an integer?
It doesn't seem necessary
i wasnt trying to. i sent u the code where i had that there
?
refer to the code i sent in the description of this post as the most up to date one
So... why did you say line 46 has the
int.Parse()
in it..? If the most recent code doesn't..?im not sure. i put it by accident i think
So line 46, even without
int.Parse()
, still throws the format error?
error with int.Parse removed
Ah, I see what the issue is
I didn't see it initially because I never use
string.Format()
since string interpolation exists
"{0.0.000000}\n"
should be "{0:0.000000}\n"
do you suggest i use string interpolation instead?
Yes
im not sure how to do it with that
oh thats what i messed up on. ty
i kind of was doing that from mostly memory
Angius#1586
REPL Result: Success
Console Output
Compile: 590.156ms | Execution: 74.727ms | React with ❌ to remove this embed.
Here's how to achieve the same with string interpolation
holy that is soo much more clean
why did it return all 0s?
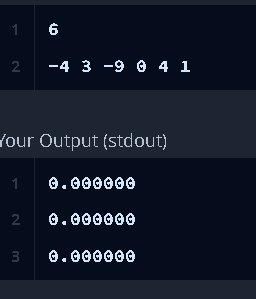
i loop over all the elements to get how many positives, negatives, and 0s there are
What's your string?
Seems something wrong with the output string, not with the values
Although you can, of course, make sure by using the debugger
this is how i calculate the output for positive numbres
Ah, so you didn't use string interpolation
not yet
I thought that might've been the issue
ill change it to that rn
Ah, I know why
Integer division
Cast one of the operands to a
double
(double)positive / arr.Count
for examplealright
it prints "0.000000"
Try
or
or
or
Some parentheses here and there might help
tried those all
same result
Huh
Angius#1586
REPL Result: Success
Result: ValueTuple<string, string>
Compile: 523.124ms | Execution: 48.761ms | React with ❌ to remove this embed.
It should work just fine
yeah i would think so. im not sure where i messed up.
I guess you could try pulling that
(double)positive / arr.Count
to a variable and using the debugger to check what the value isalr
Maybe it's so small that 6 decimal places is not enough to show anything
i might need a little break. been going at this for a while. my brain is tired.
Yeah, get a break, drink some water, come back to it when you feel like it
alright
thank you for helping me
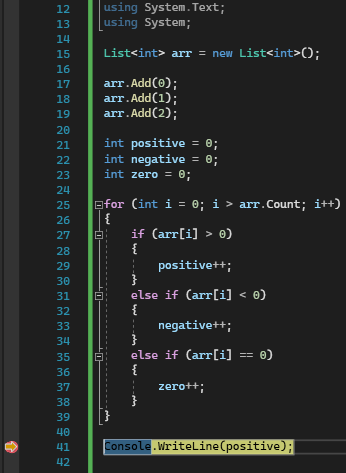
i did some debugging
i just made a list to test this code
there are 2 positive numbers
and 1 zero
i made a break point
when it reaches line 41, positive = 0
how does that make sense
positive should be 2 in this case
@Angius
i think thats been my problem this whole time
although i cant see a reason why it would be 0
That does seem super weird indeed
I'd probably start by turning that for loop into a foreach, just to make sure it's not an issue
Doesn't seem to be and probably isn't
But it's best to get rid of as many moving parts as possible
alright
i dont think ive ever used a foreach
let me figure it out gimme a sec
Let's you avoid the issue that @Box⸬læk⸬<'static>(Box⸬new(Aaron⸩ pointed out, with

turning that into a less than seemed to fix it
i thought it was a greater than tho
i guess i just forgot the syntax a little..
Loop runs as long as that condition is true
It's a run condition, not a stop condition
Also,
foreach
just does that all for you lolshould i use foreach rather than a for loop when i can?
im more familiar with for loops
Foreach is much easier to use
Use whatever suits you, through, and learn as you go
all my tests pass after changing the greater than symbol to less than in the for loop
:/
such a little thing i missed
that took me this long..
im gonna try to replicate this with a foreach
and see which one i like more
It's always the little things lol
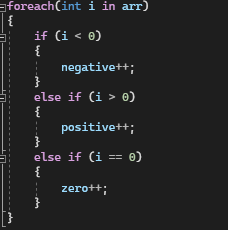
i figured foreach out pretty quick
that seems much easier
are there cases where one is objectively better than the other?
Pretty much always foreach
Unless you need the index, then for
the index?
yeah the tests all passed with the foreach. wow that was a lot cleaner
The number of the element in the collection
oh yeah
is there an alternative to all those if statements?
I'd probably use a switch expression
That said, I'm on mobile now, so it'd be hard to write it out lol
I'll let you do some googling, and if nothing comes up, I'll write the code up when I'm at my PC again
alright
Ah, no, wait, a switch expression probably won't work here... unless we get fancy with tuple deconstruction
Those ifs are prolly your best bet
Easiest to understand and work just fine
I'd maybe turn the last else if into just an else
yeah i was about to say i have no idea how im gonna use a switch here lol
good point. not sure why i had it typed like that.
@Angius is there a method that i can utilize to round to the nearest 5?
here actually imma close this. im done with that problem. ill open a new one if i need help with something else
Closed!