✅ Mapping an Identity user to an instance of an EF core model ID
Any suggestions on how to make an identity user have a model ID? I need to do this so I can have a custom view for all users . My plan was to just use loops and only show content where IDs match up. Not sure where to begin.
53 Replies
What do you mean by "model ID"?
Do you have an entity
Model
and want to create a relationship between it and the user?Yes
Then just reference it
Or create a custom user class if you want, by extending
IdentityUser
How would I get this to line up? I need to see if the Entity (The Identity User) Is the Student or the Teacher. I dont think the code I have so far would work
You can use roles for that
Instead of two different types of a user
so we were suppose to use claims, And I have working claims. Now how would I make each individual user have a seperate instance? Example would be if a student wanted to view their specific courses or grades. how would that be done?
okay that creates a where clause that connects grades to student. I have that as well
A grade would be something like
wait a minute
so I have this for my Grade
That also works, sure
My example was a very simple "teacher gives grade"
and I have this for my student
so how would I make this student a user?
You wouldn't have a student
You'd just have a user
You can make your student a user if you inherit from
IdentityUser
thoughWe were given this diagram to follow, is it possible following this diagram?
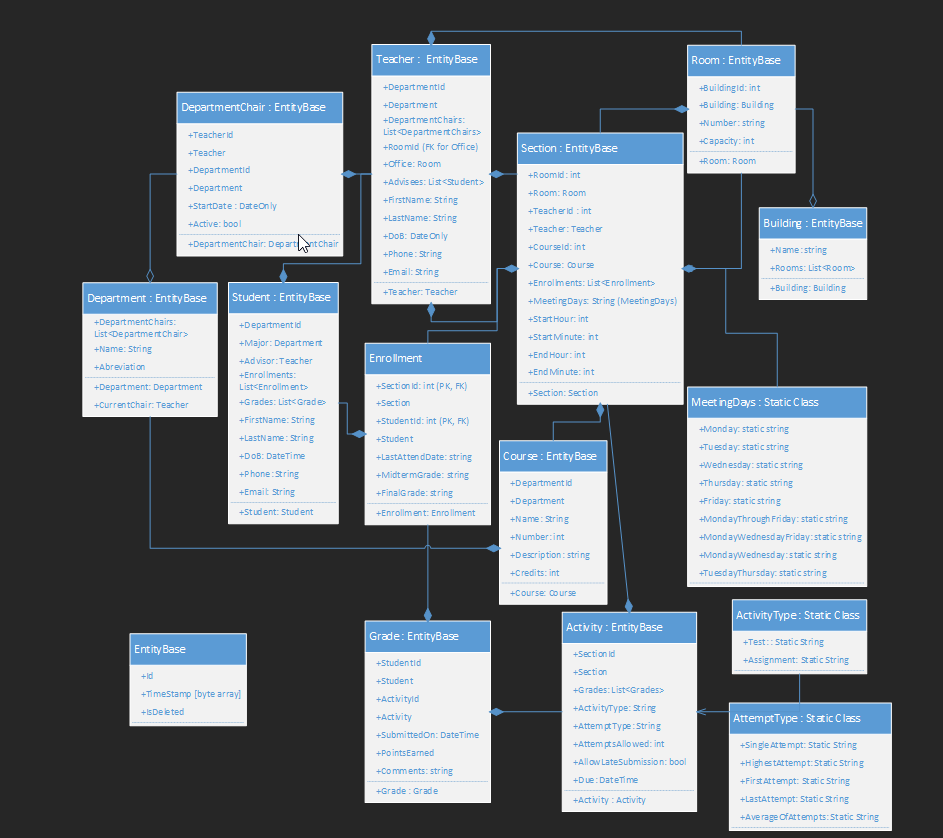
I'm implementing it incorrectly, not sure
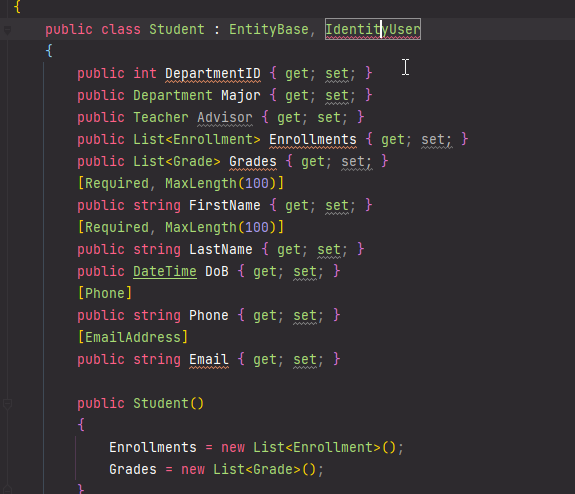

There's no multiple inheritance in C#
yeah 😦
A class can inherit only from one other class
So it's impossible to recreate this graph 1:1 while using Identity at the same time
could I make a middle man class for instances like these?
How would that work?
That's frustrating
Think I should just ignore what the professor was saying then? He wanted us to use Identity with that, But it seems like you are saying what I was thinking in that it's not going to line up correctly
IdentityUser
has to be somewhere
Either as related data, or as a parent classWhat if I made Identity user a field in student and teacher?
You could have
oh that is interesting
And, yeah, put a nav property to
BaseUser
inside of both Student
and Teacher
never heard that term. What is a nav property? Same as a field?
You'd need to at least also change the class your
DbContext
inherits from, from IdentityContext
to IdentityContext<BaseUser>
$structure
For C# versions older than 10, see
$StructureOld
Properties have the
{ get; set; }
oh I see
"nav properties" or "navigation properties" are an EF term for properties that point to other database models
Since you can use them to navigate from one model to another
for example
wait, If I am doing it like that, would I need to put ApplicationDBContext in the student and teacher Models as well?
No, why?
DbContext
represents the databaseIm looking at where you did _context
It's not a table
Yeah, it's a reference to your
DbContext
My imaginary scenario looks somewhat like thisOh okay, now I think I am back on track. I think I get what you mean
Would this map the Identity User to the Model correctly then?
Then
LGTM
yeah! Okay good so I did the controllers correctly
alright I am going to test this out 😅
should I drop the previous database and delete the migrations?
I think I will just to be safe
It's a big change, so yeah, probably
Just make sure to commit what you have
Just so you can revert the code should anything go awry
yeah I've got several backups at this point lmfao
Hope you're using Git, not a bunch of
myproject1.zip
myproject2.zip
myproject3wtf.zip
loloh snap, So I used scaffolding to make my controllers. I should regenerate those as well since the model changed?
Or modify them by hand
yeah.....
code gen it is haha
Generally, it's a better idea to not use scaffolding. The code it generates is... good for tiny little test projects, but not for production, let's just say
Is it normal to have heart palpitations here?
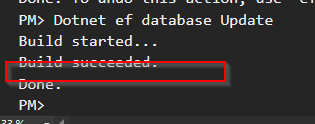
With big changes? Yes 😄
So I made an admin account and I went to add a teacher to the teacher scaffold. It does not look like its asking for a user here?
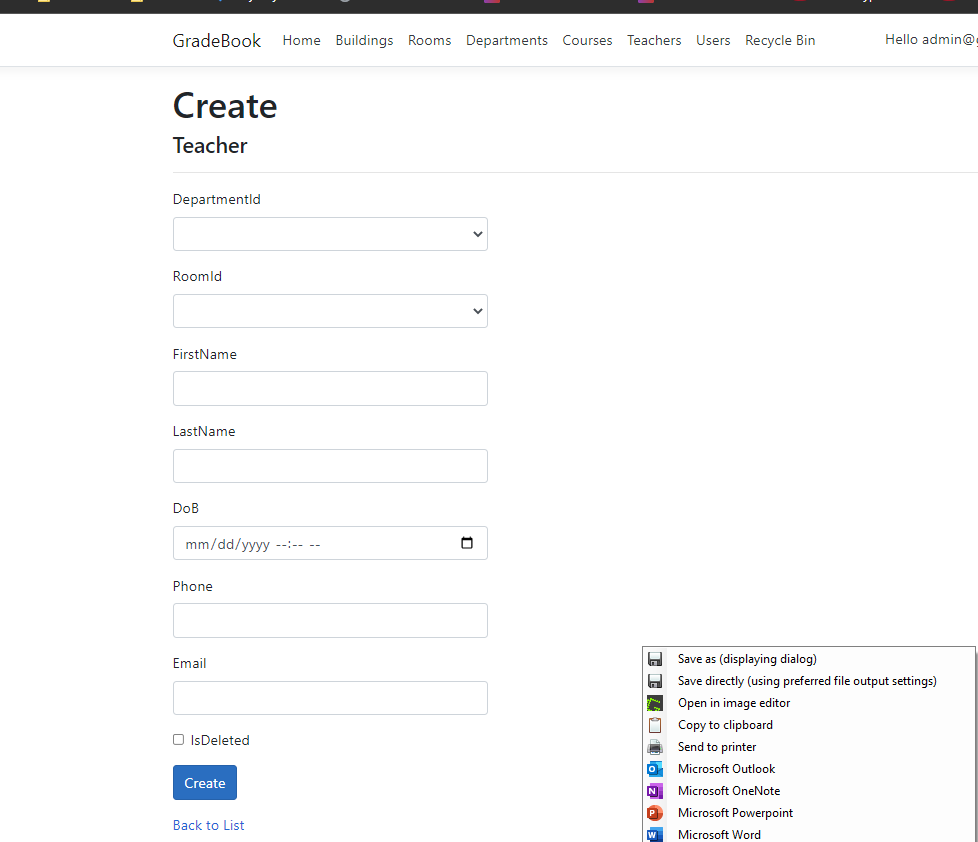
Same with student
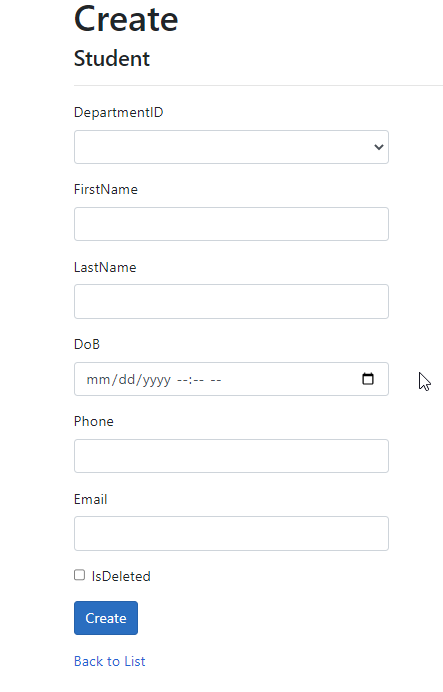
That Did not work
Closed!