Is there a way to improve the way I do type definition of the argument here with the zod object?
5 Replies
You can move the input Zod schema outside, then infer the type from it
I haven't worked with zod that much, can you show a simple example or just link a reference? Anything is helpful, thank you so much
One sec, not on my computer lol
@amanuel could do this
Yes, this is perfec
Thank you so much for the example
yeah no worries 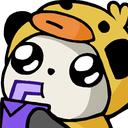
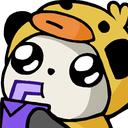