When to use Null Forgiving (!) vs Non-Nullable casts
In this example, n needs to be cast from int? to int
My question is why null forgiving (!) syntax does not seem to work (new int[n!];] and where null forgiving syntax would actually be applicable.
60 Replies
That's a very weird thing you're doing there tbh
Parsing once, discarding the result of the parse, then parsing again..?
it's supposed to be
I omitted the while loop because the question is about null forgiving vs non-nullable casting
didn't wanna detract from the main query
You're still discarding the result of parsing for some reason
I'm discarding the parse in the if statement, I'm only assigning if the if statement is fulfilled
what's wrong with discarding if it's just a conditional checker?
Because then you parse it anyway
can just be
This is the actual code
I will correct it to
You can even, instead of doing
do
and guarantee that
n
will never be null
Also, you're using int.TryParse()
, something made explicitly for the purpose of avoiding throwing format exceptions... to throw a format exceptionUnknown User•3y ago
Message Not Public
Sign In & Join Server To View
oh so that's what's triggering it
smh
so parse is throwing the exception
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
that's good actually
it severely shortens my code
Your best bet is to parse once, with
.TryParse()
and then just catch the exceptions after
i will do this
thank you
No
Why catch exceptions
int.TryParse()
lets you avoid exceptions
Don't make your life harder for no reasonI'm trying to intentionally throw and catch exceptions
it's a learning type of thing
I'm just gonna throw them more efficiently
Rethrowing an exception isn't any more efficient than letting it bubble up lol
exceptions are never efficient
yea
But sure
As long as you never actually do it this way in the wild
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
Kinda like learning to use a spoon by peeling an apple with it
But whatever works for you
Sorry @surwren your message contained blocked content and has been removed!
I'm ret(@)rded ok
i need to learn by getting my hands dirty
this is my own way of learning exception propagation
but you guys have been a great help, thank you
why doesn't this board let me insult 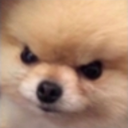
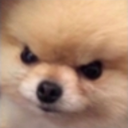
because it just detects the insult, not whom it refers to
it's not letting me do it
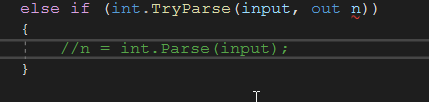
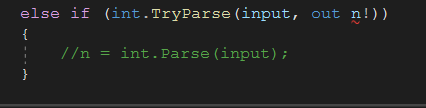
because its a syntax error
its
out <type> <variable name>
u r missing the type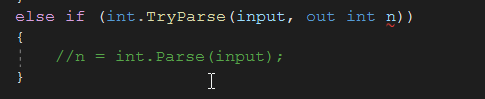
or is
n
predefined?int? n = null;
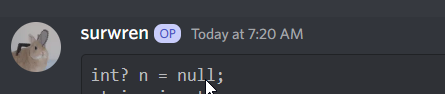
then u cant use
n
as out
variable
because int?
and int
are different typesyea
that's why this thread was asking specifically about nullable casts
vs null forgiving
so like
I have to do this then
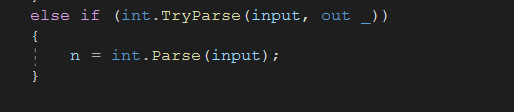
like i was doing 

Why do you need
n
in the outer scopeAngius#1586
Quoted by
<@!85903769203642368> from #When to use Null Forgiving (!) vs Non-Nullable casts (click here)
React with ❌ to remove this embed.
This guarentees
n
will never be nullit would be more (but still not really) correct to do something like
Check for the result of
int.TryParse()
insteadinstead of
out var tmp
u could also use out var tmp
out int tmp
as var
will be inferred to int
huh
Prolly meant one of them to be
out int tmp
uups, yes
*fixing*
oh its the other way around from my message 😂
I believe you can do
Since
out
kinda "promotes" the variable to the outer scopenum
out of scope after the while
loop?yeah i thought this would be it too
in c#10 it still is
Ah, huh, errors out
My bad
again, I still don't know what the proper context for usage of null-forgiving vs non-nullable cast is
Null-forgiving: use it rarely if ever
It's a workaround
A hack around the sorry state of nullability in C#
It's best to simply ensure the value isn't null
And either do something if it is, or provide a default
should do it
Ye
For example
In case
num
is null
, replace it with 0
99% of the time, anything is better than null-forgiving
what syntax is this
ternary?
x ?? y
is short hand for x != null ? x : y
so sorta yesNull-coalescing
I'm just gonna stick to casting int?n to (int) n
for now
Are there other commonly used examples similar to int.TryParse that also help you to avoid exceptions?
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View