Keyset pagination with filters
Hello everyone!
I use this piece of code (probably very inefficient piece of code) to get employees from database using keyset pagination.
This code works perfectly fine unless i activate filters. I get an exception that ef core can't translate my shitty Linq to SQL and that's kinda understandable. But the question is, how can I make this work? Afaik loading all entities to list, filtering and then taking, for example 50 entities isn't a good idea.
12 Replies
sorry for my english and thanks for help!
Post us the exact details of that "can't translate" exception please. Then, I've often found that is the result of using custom functions in your where clause like
GetDepartmentName
. One solution is to first query and get a dictionary of all departments for you employees
collection and do a dictionary lookup instead of using GetDepartmentName
It would add complexity to this method, but ill try that
also somehow this can't compare two chars, but can compare two strings, so when I convert gender char to string, first filter works
It's not failing on your
GetDepartmentName
, but it looks like it's failing, for the first time at least, on e.Gender[0] == allowedGender
. Looks like it doesn't like indexing on a string. A possible solution, assuming GenderFilter.GetAllowedValue
returns a char
, is to use e.Gender == allowedGender.ToString()
.yeah, I did that and it works
thanks
Oh, you already found that out. It's not that it can't compare chars, but it can't use indexing on a string like
e.Gender[0]
im trying to do something with this now:
One solution is to first query and get a dictionary of all departments for you employees collection and do a dictionary lookup instead of using GetDepartmentNameIs there any better way to do this? I don't want to store in memory, for example 20 000 salary records wait a minute, i have an idea... nevermind, it's worst linq i have ever seen and it does't even work
You could use a subquery to get e.g. department name and trust that the database will optimize those lookups
Wow, it works, thanks!
query looks like this:
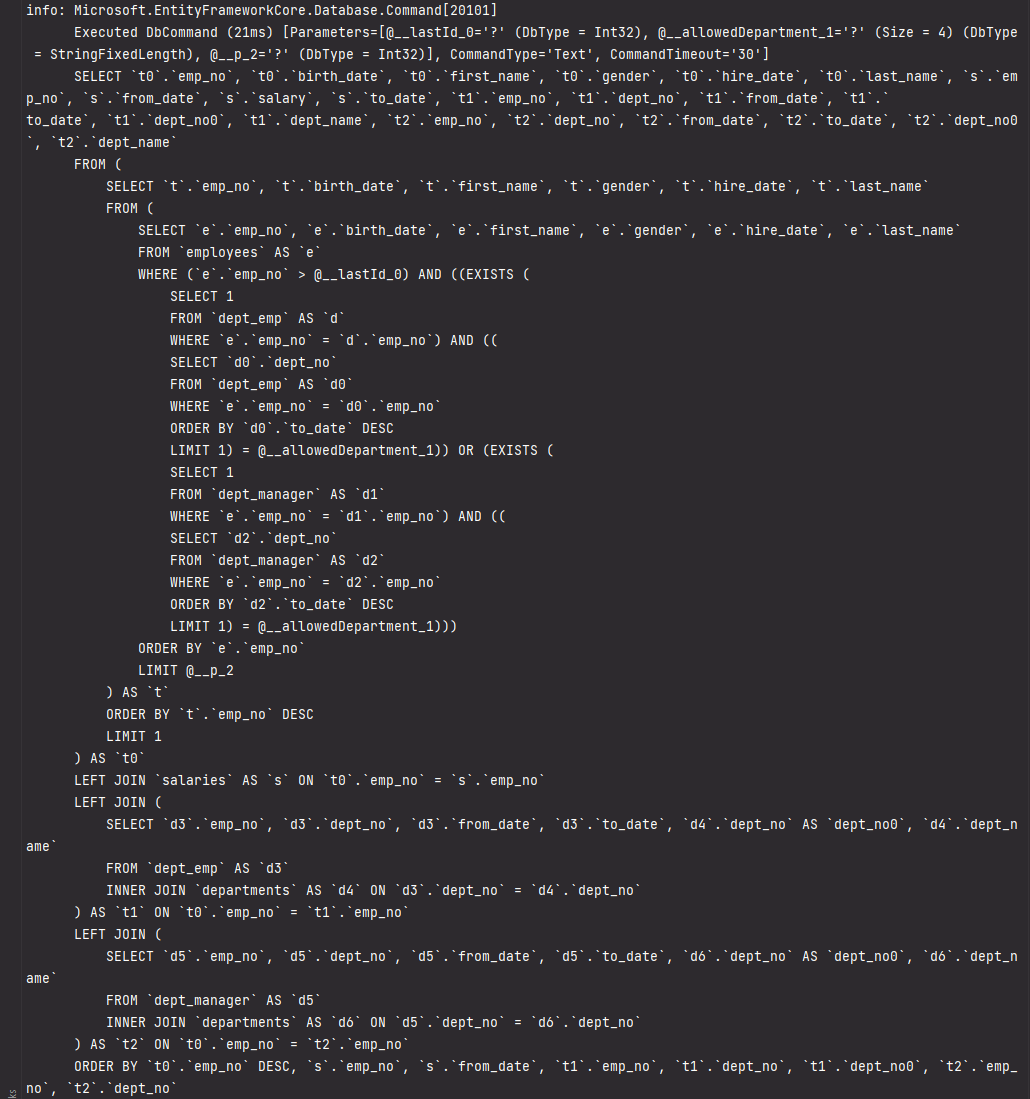
it probably has horrible efficiency, but it works 😄
It doesn't look too inefficient. Mush better than separate queries for departments and salaries for each employee