[ASP.NET] Middleware Stops Controller From Receiving Login Request
These are my buttons in /Views/Login
These are the buttons in /Controllers/LoginController
This is the middleware code in program.cs
For some reason, it looks like the middleware is preventing the user from using the controller IActionMethods in /LoginController to get a session ID. But this seems to be happening anyway even though I am checking that (context.Request.Path.StartsWithSegments("/Login/")).
FWIW, even
will not let me through to the IActionResult.
What is going wrong here?
30 Replies
Just change
if (context.Request.Path.StartsWithSegments("/Login/"))
to if (context.Request.Path.StartsWithSegments("/Login"))
lmao it actually works
why doesn't /Login/ work? technically the full url is /Login/Index, so why is .StartsWithSegments() not able to get the correct path
Actually a better question would be how do I troubleshoot this because nothing appears in the call stack variables
The way
StartsWithSegments
defines a segment is basically
/Login/
is a whole segment, so /Login/AddLoginCookie/
can't match the whole segment of /Login/
Meanwhile /Login
means something can come after it because it's not terminated with /
Unfortunately if you don't know the behavior of StartsWithSegments
it would be a bit troublesome, your best bet would be to attach a debugger and investigate which part fails
btw off topic, your pfp is really cute š
You mean like x64DBG?
I did put in breakpoints in MSVS but it just skipped over the initial conditional
No no, with a managed debugger in your IDE
Were you in release build?
I think it's development, how do I change it?
You can change it from the top menu here
Ok, I'll try it in a few min when I'm back at the pc
You were probably in Debug build anyways, I thought Release might've caused it to not get hit
hmm
yea, I am in debug

When you placed a breakpoint what happened?
It stops at the breakpoint but the variables of the call stack don't show any helpful info whatsoever
lemme grab a screenie
like
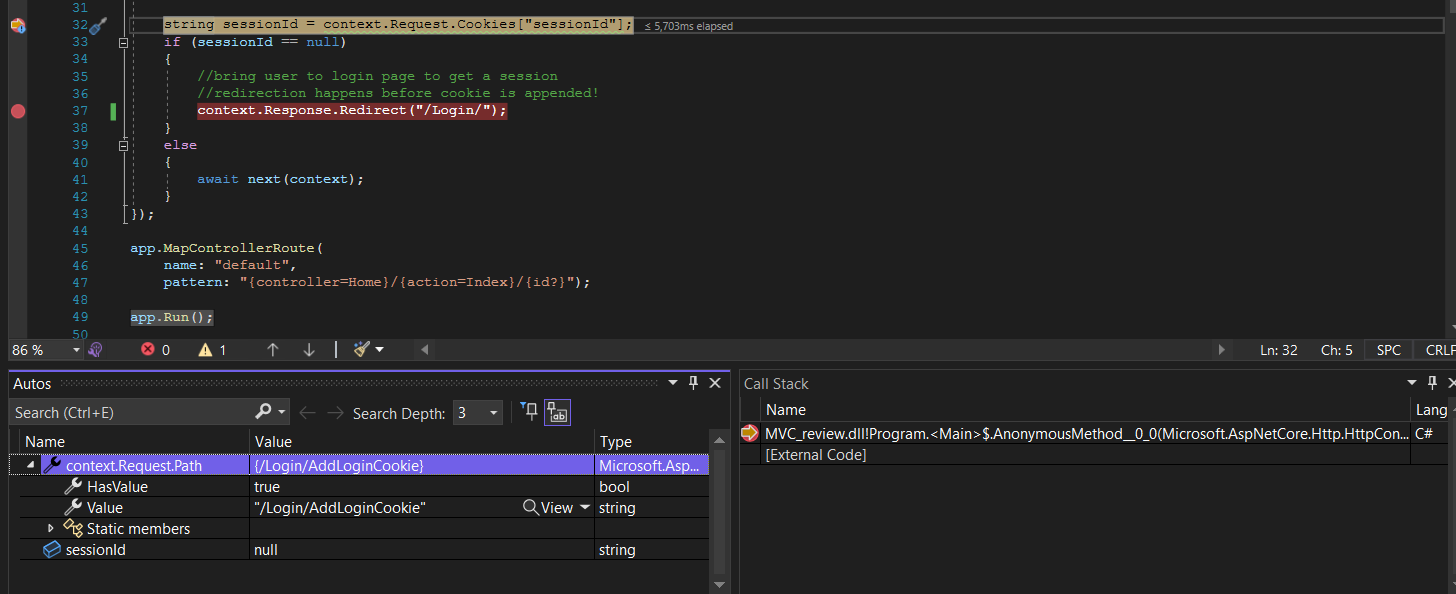
it shows the path
then it just skips and doesn't give any indication why it evaluated false on the path
this too
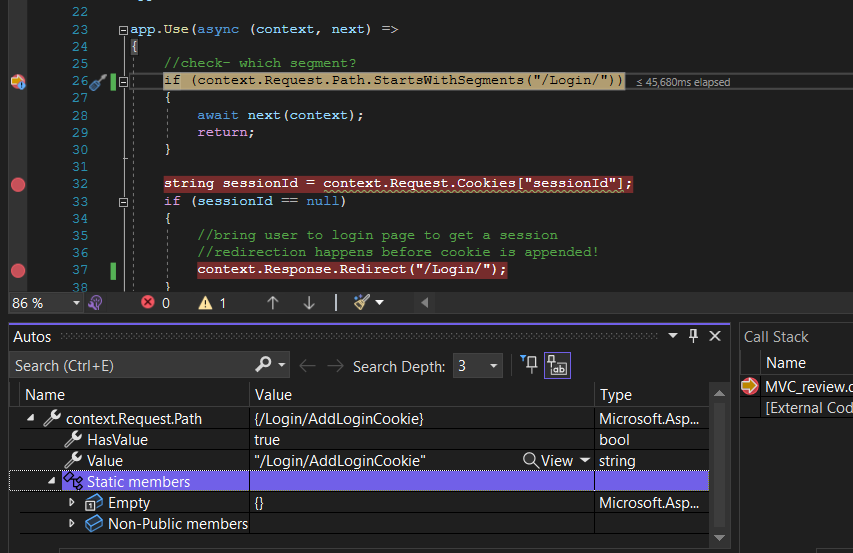
I mean I understand the solution now, but if something similar were to occur I would have no way of troubleshooting it on my own in the future
š
Oh, yeah, that would be normal
StartsWithSegments
only returns bool
so there is no way to really know why it failed with running the code.
You can however run code in an interactive code thingy (I forgot what's called š
) and see what works with StartsWithSegments
or if you're comfortable with reading the source code for it, you can find it on githubI'm confused, what keywords should I look for for this interactive code thing? Or do you mean the source code/BCL for ASP.NET is on 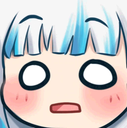
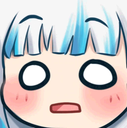
Yeah, I meant ASP.NET on Github, only go that route if others fail first and you're comfortable with that š
Lemme just open VS and show you what I meant with interactive code thingy
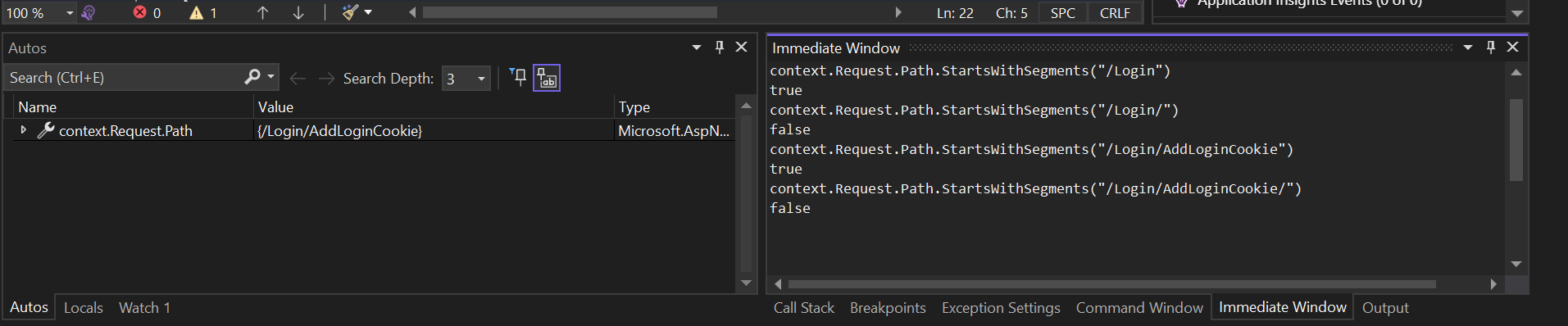
You can evaluate different expressions in
Immediate Window
Is there some setting to activate it? My immediate window is blank
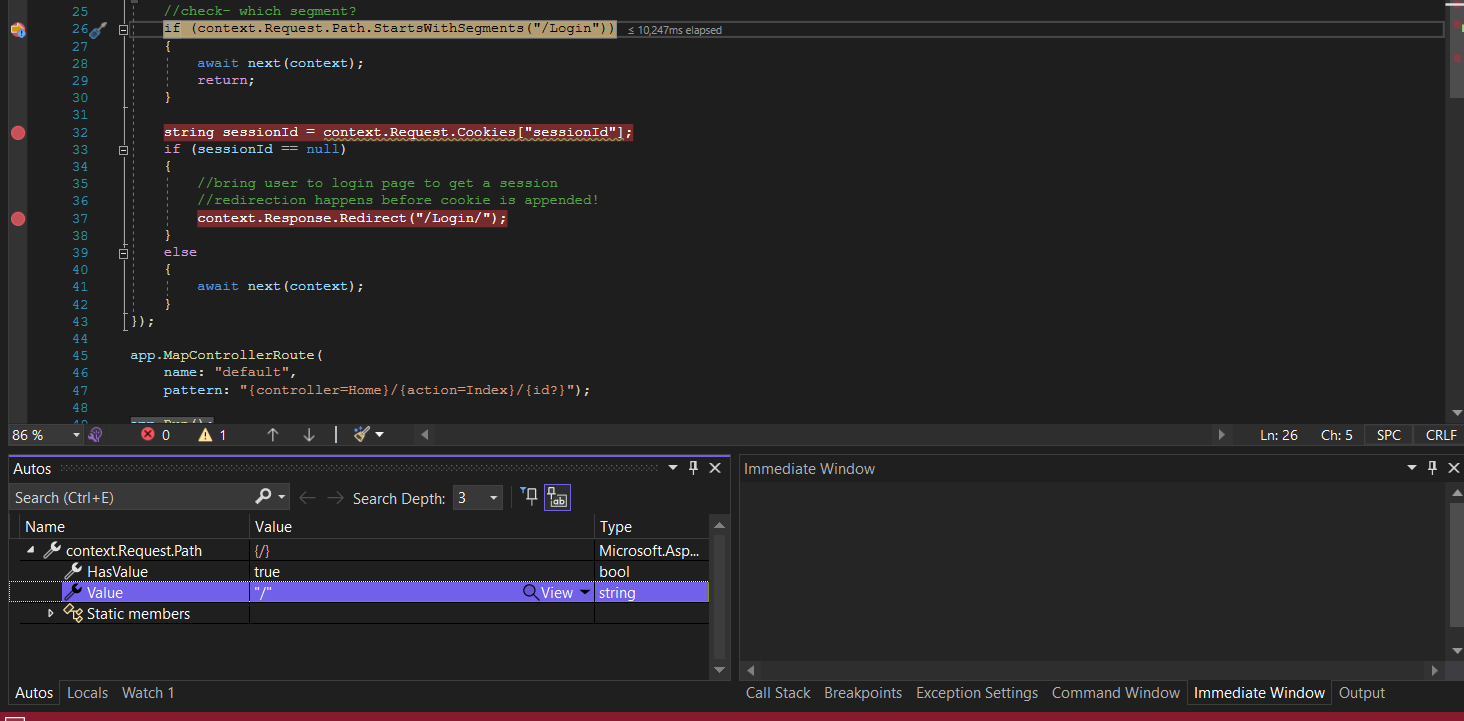
You can just type any expression you want!
it works!
thank you
I have an additional question @Kouhai
Why does middleware use the async syntax/keyword?
Is it mandatory for middlewares to use async?
I feel like I am lacking some comprehension of middleware necessary to connect the dots but idk what it is
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
Are middlewares always lambdas or is it this specific middleware?
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View