Slash Command optional parameter [Answered]
Hi, I'm currently working on a poll command where I'm using SelectMenuBuilder and I want for there to be 2 required answers to create the poll. I want the rest of the answers to be optional. Is it possible to make parameters optional or will they always be required?
So far I've only been able to find the [Optional] but it only hides it when using the command, but I'm still required to enter all 8 answers to make the command work...
60 Replies
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Wouldn't that just make the unused answers pre-filled? 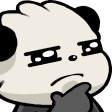
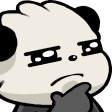
I think OP's question is more referring to Discord command arguments. To answer your question, is it possible to have 2 required answers, then each subsequent answer optional? The answer is no, all of this information is registered at the Discord level as a part of the command itself. There's no dynamic checks on the Discord level to see if the parameters match your logic
Notice how your bot's logic doesn't receive the command until it is actually executed, but Discord knows all of your parameters. This is because you register the parameters of the command initially, and when the command itself is executed then you're able to perform your logic
What I'm looking for is something like this bot. When using the Slash command you can enter different answers but you can leave the unwanted once blank.
@pip If I'm understanding your message correctly then it's not possible for me to do this with my variables as parameters, is that correct? 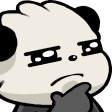
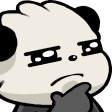
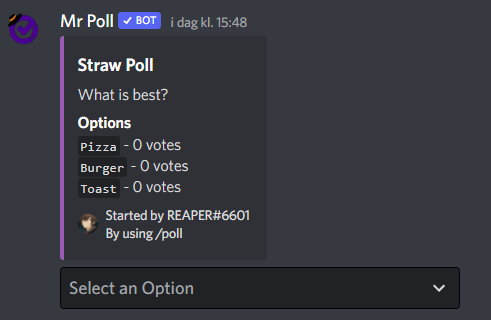
well isn't that just using MaxValues(2)? in your example
that's maybe slightly different from what you described
of creating optional parameters after hitting max values
If I use MaxValues(2) then I will allow the user to vote for 2 things in the poll
Ok I think i see the problem
Yea so you want all parameters optional, but you need at least one parameters for the command to execute
I need the Question and at least 2 answers and then the rest of the answers are optional
So just use MinValues(2)? I'm following with you
I'm sorry if I'm misunderstanding the point, but I've read everything you've written so far
I might be explaining things a bit weird some times š
AH
The MinValues forces the user to select at least 2 things to vote for right?
allow me to fix your code
I'm perfectly happy with my pollBuilder but I'm having issues with the answer3,4,5,6,7,8 in the parameters if I don't enter something in them when using the slash command
public async Task PollAsync(string Question, string answer, [Optional] params string[] options)
{
var pollBuilder = new SelectMenuBuilder()
.WithPlaceholder("Select an option")
.WithCustomId("menu-1")
.WithMinValues(1)
.WithMaxValues(1).AddOption(answer);
foreach(var option in options) { pollBuilder = pollBuilder.AddOption(option); }
var builder = new ComponentBuilder()
.WithSelectMenu(pollBuilder);
await RespondAsync(Question, components: builder.Build());
}
this worked a year ago or so
i'm not sure if it still does with slash commands
so try that out, see if that fixes your problem
the other way to do it is with the parameters you have, then just do null checks on each one. if not null, add to selectmenubuilder
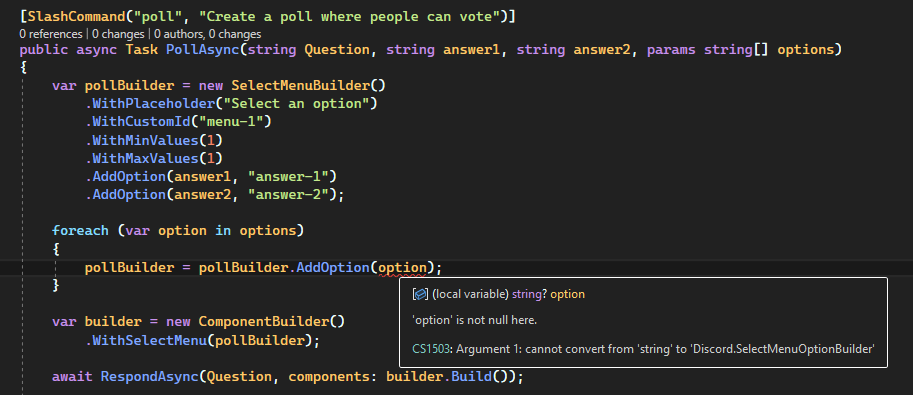
It's apparently not happy with the type
and yea, keep your stuff with answer1 and 2 at the top
This is what I currently have
When I run the bot I get this error?
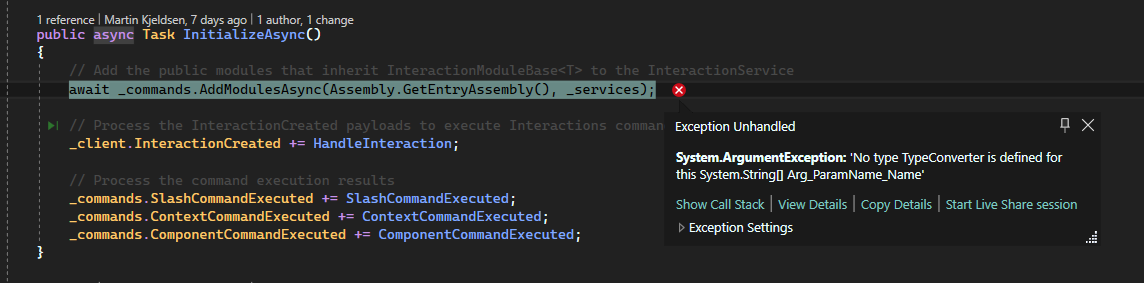
hmm so it doesn't like the optional params
let me check the docs rq
Gotta admit sometimes the written commands were easier to make š
They for sure are much easier. Slash commands are rough to work with
On the other hand they are way smoother to use 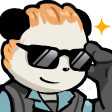
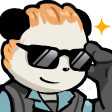
yea better for users
um
just use your initial solution
and do null checks
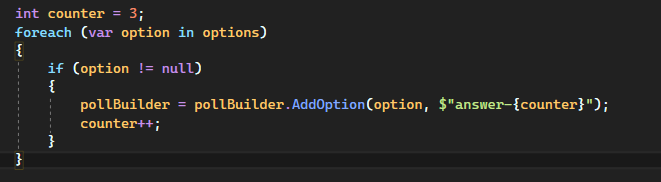
Like this?
nah remove the params thing I told you to add. use your initial optional stuff.
if(option3 is not null) { pollBuilder = pollBuilder.AddOption(option3, "option3"); }
and the same for 4,5,6,etc
it's not pretty but i'm not sure how to do params with slash commands
Is this what you meant?
yup
its ugly af
but it'll get the job done
Well sad news is that it didn't
still can't leave the other answers empty/null
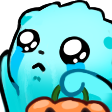
o
Yeah 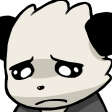
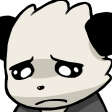
try Optional<string> instead of string? Now im just guessing lol
their docs aren't great
ok that doesn't work
Yeah I couldn't get it to work either
do me a favor
hover over "Optional" and tell me where it's from
It's from
System.Runtime.InteropServices.OptionalAttribute
so here's the thing, all this is possible via the SlashCommandBuilder(this is what I use). I can't find any attributes/methodology for this using the interaction module
the reason i asked this is because [Optional] isn't a part of Discord.Net, I don't think this will work?
Well so far it has worked fine. It doesn't make the the variables optional to fill out but it hides them away so you don't feel obligated to enter them
Like this where it shows the first 3 and for the rest it just says +6 more

I personally don't think it's coursing any issues
I just tested some of the old code we did. If I remove anything that could course issues and changes the optional strings to the params string[] then I still get the compilor error. I don't think Slash Commands like params... 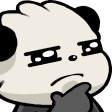
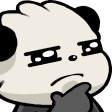
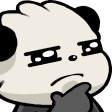
This is the error
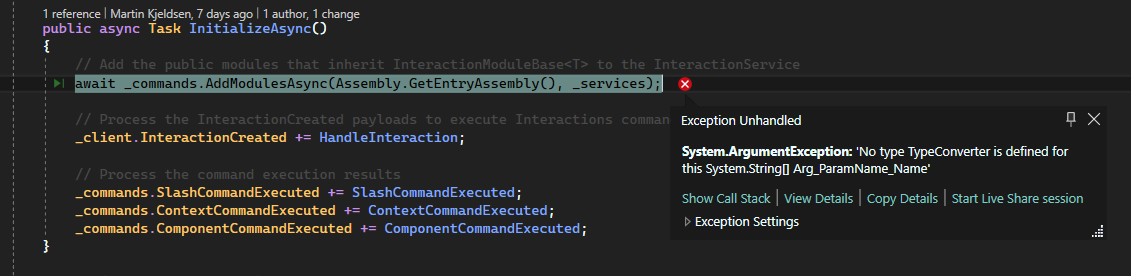
yea i tried it as well, this is something that used to work with regular commands
causing* not coursing btw
Oh yeah sry š
all good, so yea I'm still looking through their docs for examples on how to use attributes for parameters
Discord.Net Docs
Class ParameterPreconditionAttribute
| Discord.Net Documentation
Requires the parameter to pass the specified precondition before execution can begin.
i found the answer
you ready?
it's much easier
than i thought
Thank god š
I like easy š
string optionalParameter = ""
Let me test that before celebrating š
i tested it on one of my bots
OMG you're right it works
was actually close to this result earlier but ended up taking another route... that was a mistake š¤£
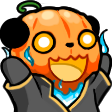
I'm almost mad that it's so easy, I wish this was documented anywhere
š¤£
(or if it is documented somewhere, maybe easier to find)
Oh yeah for sure but on the other hand I'm not complaining xD
Once again thanks for taking your time to help me maintain my bot when I'm running into issues 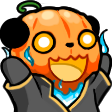
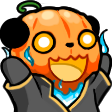
no prob
ā
This post has been marked as answered!
I guess the first guy wasn't that far of then š
yup he was completely right
lmao.
š¤·āāļø
That's actually even more annoying that we could have found the solution faster if we had just listened more š¤£
you're completely right