CSV Invalid Header Reading [Answered]
I am currently a CSV with a header of "province;red;green;blue;name;x", however my program expects to be able to read CSV's that are strictly
int;int;int;int;string;x
as opposed to (oops!) all strings as given.
I cannot change the CSV; not allowed under any circumstance. I need to implement handling of invalid entries like this but I am uncertain how, especially in a way that won't bog down my program.48 Replies
This is the given context in code:
I forgot to mention the error specifics,
province;red;green;blue;x;x
as Row 0 (first row) is obviously the problem, but being that i am not allowing myself to physically change the file, I am at a loss.Just skip the first line
Advance your StreamReader by one line before passing it to the CsvReader code (probably, I don't know what the CsvReader does...presumably it doesn't do something dumb like seek back to the origin)
your csv has header, why set HasHeaderRecord = false
heh, I didn't even notice that
Most files don't have
province;red;green;blue;x;x
, this is more or less just handling the exception
I figured I needed to skip the first line, but with this reader i'm not entirely sure how to detect when exactly I need to skip it, or when a CSV file's first line is perfectly readable as is.try use BadDataFound
if your CSV is good then you can just set it to null
you might get a blank record for header line if i'm not wrong
Tried. Doesn't have any effect.
it still throws error?
Yeah.
at which line? is it CSVHelper exception or your code
Line? I'm not sure, 1 second.

can you show your CSVConfiguration?
Yepyep.
hmhm
have you tried debug and seen records value
before your Parallel
is it error?
I mean check the records value there
Error might happen because you're trying convert those header to Int inside your Parallel foreach.
You need to clean them first or put a check
I cannot get the record's value, but I'm certain I know what it is
Because the first record is
province;red;green;blue;x
. Formatted, this equals to string;string;string;string;string;x
which is very bad, because i need int;int;int;int;string;x
I'm certain it's the first record that's the problem; given the error kindly notes it. records
shows null, due to the aforementioned bad record entry.I mean you can check if your record is valid first before process
is not valid
?put your own condition there
I'm not sure what condition to put there
An invalid record only exists at the beginning aswell.
do you know int.TryParse?
Yes
lets check if it can parse provinceID as int successully
then continue else bye bye

Regardless, this check would be running 3000+ times when the problematic record only occurs once. It just feels... weird to me.
er
remove "record is not"
does it still show error?
The error is within the tryparse. Removing
record is not
has made no affect.zzz. You have to remove that, it makes syntax wrong
take a look at it man
calm down
?
Alright,
if (int.TryParse(Convert.ToString(record.provinceID), out int value)) return;
Does not spit out an error.
However during testing, the same error still appears.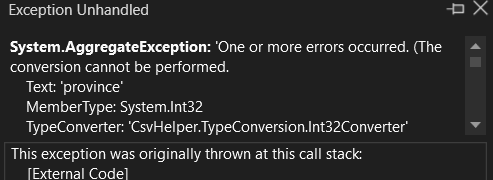
BadDataFound = x => Console.WriteLine($"Bad data: <{x.RawRecord}>")
set BadDataFound = null
and try use normal for loop
debug and see
A normal for-loop? Alrighty then.
I don't have good exp about parallel for each so i can't tell
From my understanding, this use of Parallel is just a for loop that is split between different threads. Meant to be faster, and boy, it sure is.
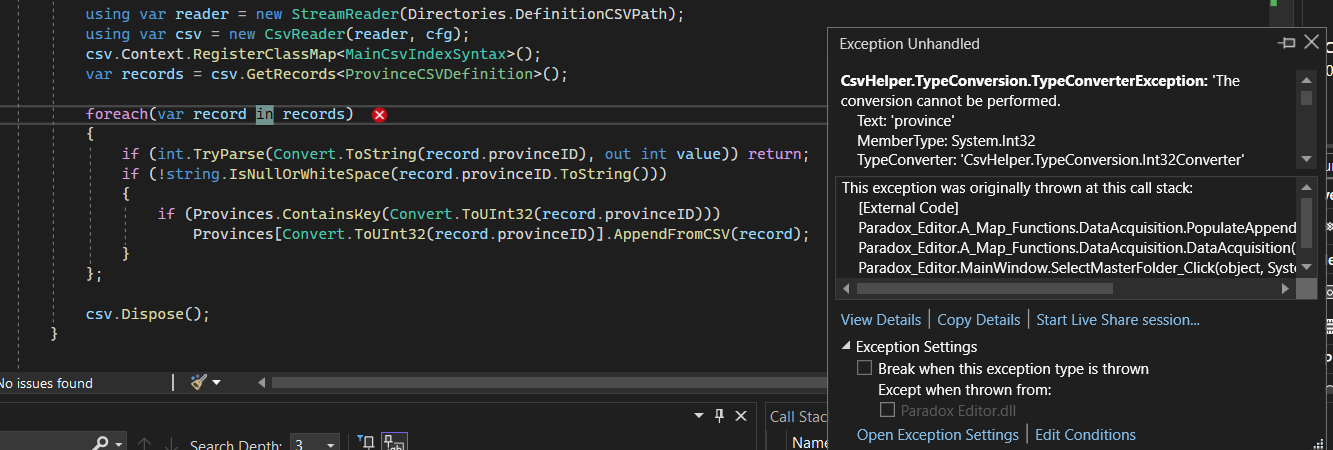
Same problem sadly still occurs. I deduce that its within the
csv.GetRecords()
that it actually begins to read the first line, and since it can't understand what that line is supposed to be since it's types are all out of wack, it throws a fit when records
is actually used.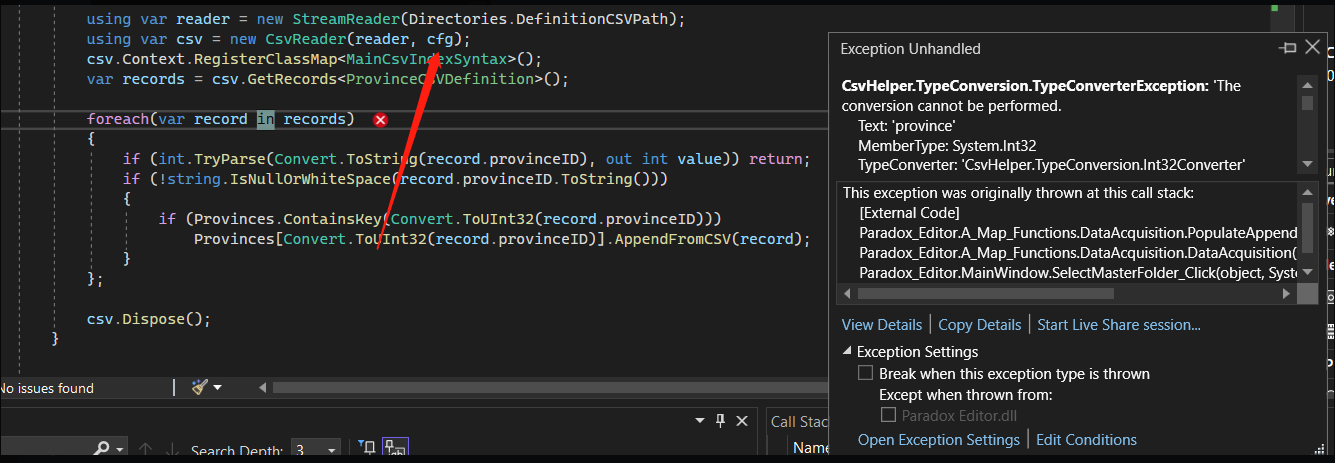
right click this,
add watch
Ayeye
No option given to add watch.
hmhm
what is there?
Alright, just got it sorted. Had to do it the old fashioned way.
Frankly i don't think
cfg
would be changing anytime soon, but here's what i got:
BadDataFound = CsvHelper.BadDataFound
you didn't set it to null?
Ah, I had, but it made no difference.
that is weird because set BadDataFound = null will ignore error and give you a blank record if i'm not wrong
It worked fine for me before
Strange.
To me it seems like the crash stems from
var records = csv.GetRecords<ProvinceCSVDefinition>();
As cfg
does not change at any point, but records is certainly not meant to be null.no, i didnt mean cfg would change but cfg will effect behavior of csvreader
I think I may be getting far over my head in trying to optimize something I shouldn't.
I've sorted it out and fixed it. Turns out theres no real easy way to manage a csv file like that with the way I had it, and am going to have to contend with directly accessing the csv variables from within
ProvinceFile
.✅ This post has been marked as answered!