How to deserialize dictionary using Newtonsoft [Answered]
I have a Json file with a bunch of pokemon data. I am having trouble deserializing the information. The console is running and showing no errors, but it exits out immediately. This is not a Console.ReadLine() issue because commenting out the:
Pokemon pkmn = JsonConvert.DeserializeObject<Pokemon>(jsonFile);
makes the console stay. I also think I have an error with how I am storing the data. I am not sure how to access a specific item.
22 Replies
name
is not a Dictionary<string, int>
Thank you, what data structure is it?
what pairs are in the
name
element?I am unsure of what you mean. I'm not familiar with C# dictionaries, only python. It is my understanding that the language is the key and the pokemon in the value in the name element.
what you should do to test this is make a dictionary, then serialize it. see what comes out. i'm thinking that you may have to explicitly specify {"key" : "english", "value":"Bulbasaur"} but that's just a guess
no
even python has types
what type is
"english"
Oh, I suppose a string.
and what type is
"Bulbasaur"
A string as well.
so what should be the key and value types in the
name
Dictionary?Oh! I didn't realize that was still set to
int
. I had changed everything to string
earlier to test.there you go
It still doesn't run though lol.
any errors?
oh, jsonconvert 🤢
I apologize for the image. VS wasn't showing an error. Had to print screen the console. I didn't realize the error until now
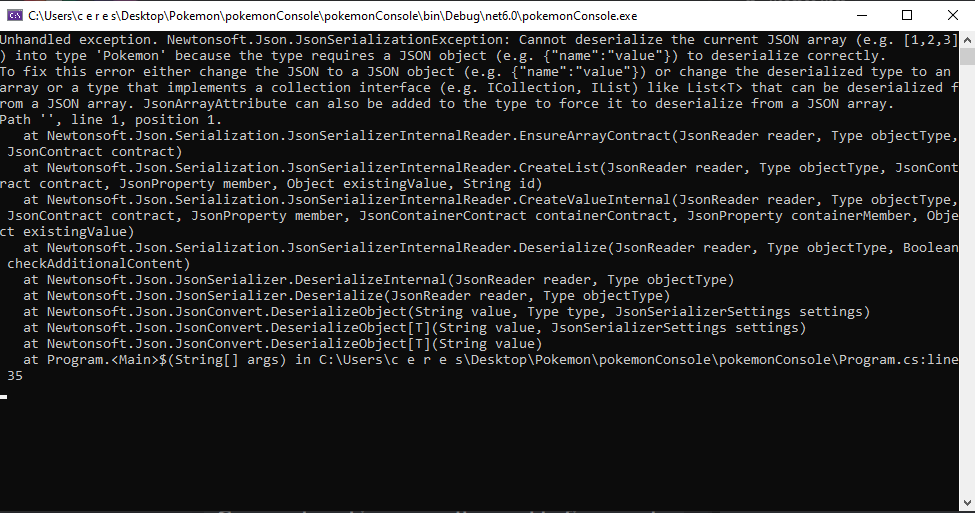
can you show your full code?
but if possible, just don't use newtonsoft
Ero#1111
REPL Result: Success
Result: Pokemon
Compile: 557.944ms | Execution: 54.307ms | React with ❌ to remove this embed.
The entire code is at the top. I'll try
System.Text.Json.JsonSerializer.Deserialize<Pokemon>(json);
Newton was what I found online. How did you color your blocks?$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/$codegif