Azure function returning error
Here is the error I get from the Azure function test:
Here is my Azure function (in two or three messages because of its size)
36 Replies
and here is the first of the two methods I created to merge the JSON objects:
and the second:
dynamic
Scrap the codesay what now?
There's no reason to ever use
dynamic
unless you're doing some weird interop
Frankly, it should be hidden behind a compiler flag or somethingI am getting a JSON from a user with strings as keys, but the values can literally be anything. So dynamic sounded like the best option... what else would I do..
Also instead of coming at me with "scrap the code", you could've started the conversation different... now it feels more like an attack than actual help...
JsonToken or JToken depending on which library you use.
Newtonsoft.Json is the lib I'm using
Then if you can't create a model closer to what they're sending, use
JToken
imo.I'll use JsonToken instead then. Sure.. though this is already a lot less "provocative" than earlier comments 😅
For your issue at hand, I'm trying to find where you call
MergeSingleAsync
.Sorry for that,
dynamic
, ArrayList
, and the like, create a very visceral response in me lolNot just in you. I generally just ignore anyone talking about $dynamic
please no
in the return of the first bit of code
return mergingProperties.Length == 0 ? MergeAll(mainObject, secondaryObject) : MergeSpecific(mainObject, secondaryObject, mergingProperties);
I understand the response, don't get me wrong. I've got the same response when I see it in dart or any language for that matter. But this is my very first setup of the program, after which I would clean such things as dynamic up into actual types and such... I needed help with an error I didn't understand, not the dynamic part 😅
now it just felt like a huge attack instead of the help I asked for. But I'm being helped now, which is already a huge relief!Oh, it's the call to the AzureFunction itself that fails, not within the method?
Oh, right. I read you wrong. yes the MergeSingleAsync() is the Azure Function itself
So what is the actual exception?
It's the call itself?
all the way at the top is the exception I got in the filesystem logs
However now I'm trying to remove the dynamic parts I'm getting issues with the way I am getting data from the req.Body
Currently I'm trying this:
But the
["main_object"]
part gets the following error: Cannot apply indexing to an expression of type 'object'
wait, I have an idea for the latter errorThe error you have listed there doesn't help much. It's either the way you're calling that AzFunction/Method or somewhere within that's not shown. When you run it locally where does it break?
sadly I haven't been able to figure out how to run the azure function locally since I'm trying to run it via Rider, and I keep getting this weird error on that part.. something along the lines of:
No idea how, sorry. I'm on VS so I just press F5.
I'm trying to call the function in the portal with this as the body:
You want JToken for newtonsoft. JsonToken is for System.Text.Json
Ah, okay. I'll use JToken
Instead of serializing, use JObject.Parse
Can you show the window (with the url it's calling, but without the key) you're calling from?
sure, give me a sec
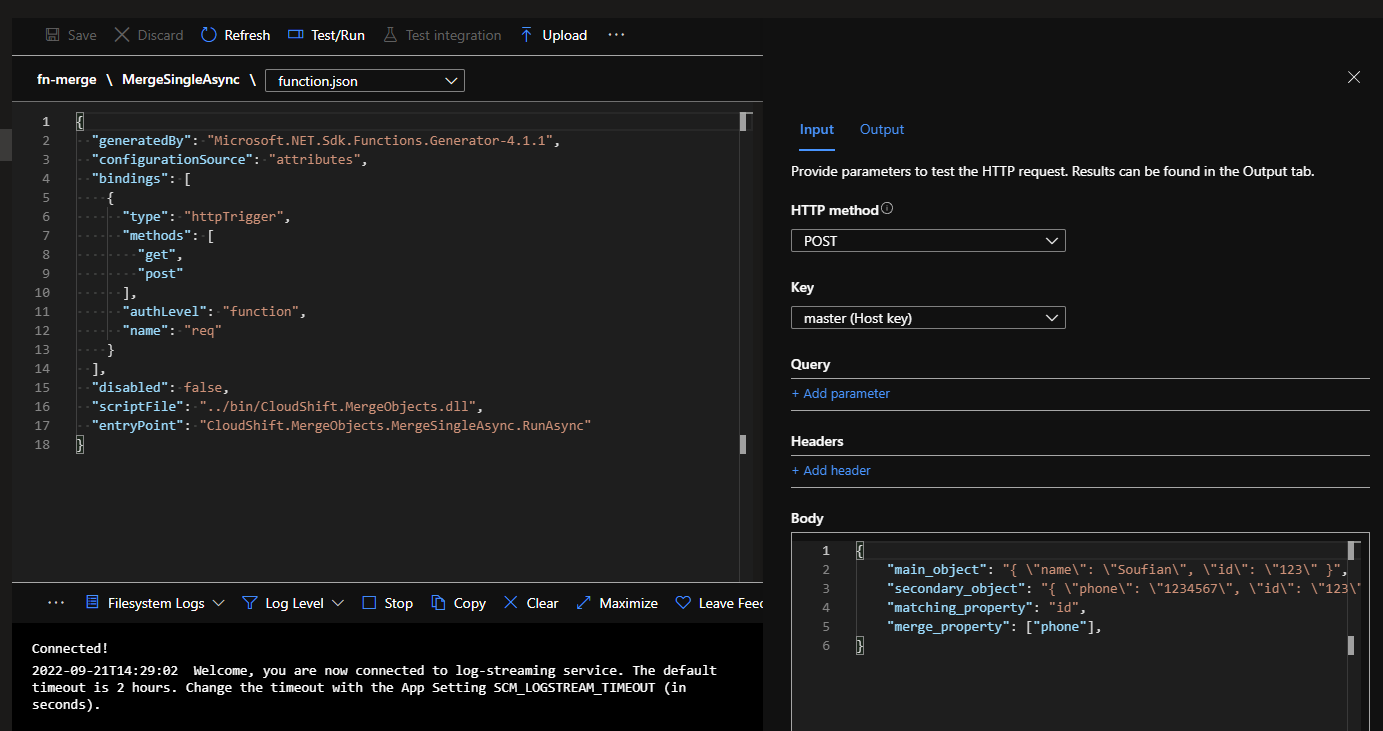
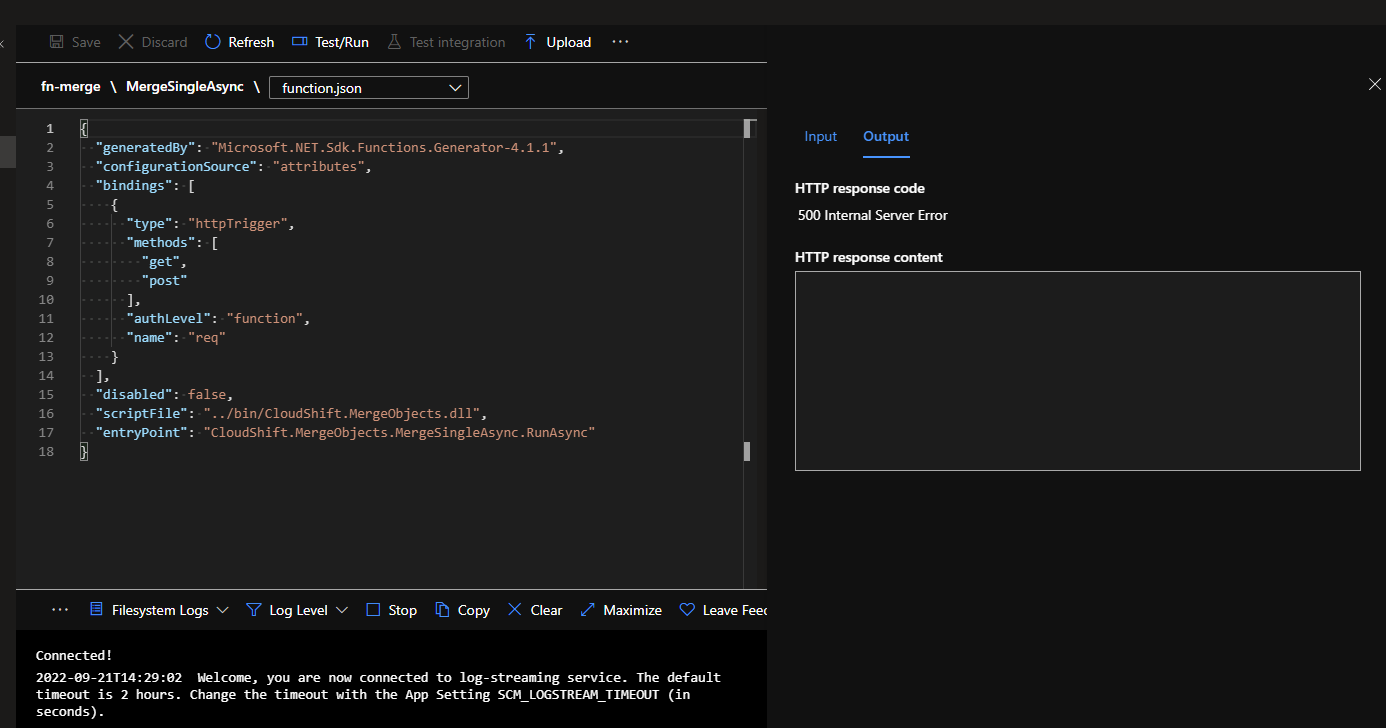
I feel like in this message, 2022-09-21T14:04:51.850 [Error] Executed 'MergeSingleAsync' (Failed, Id=f50af7c5-6b25-4c1a-ab39-7f8aff066255, Duration=2ms)The best overloaded method match for 'string.this[int]' has some invalid arguments, the
string.this[int]
is in reference to the POST, and it can't find a method that fits within your dll. Could it be that your DI isn't setup properly?
I'm just throwing an idea, but I'm unsure since you can't run it locally.I'm mainly getting now "Could not cast or convert from System.String to My.Namespace.Inputs"
Here's my new Azure Function with dynamic removed:
with the Model for the input:
and the req.Body I'm using:
(you still have dynamic in there btw)
Oh I do o.O
OOps, that should also be
JToken
sorry 😅 consider that editedBut if you get that message, it's running or it's when building?
it should be running..
imma publish again to the dev function i created, and wait a few minutes before testing again..
your request body is 4 properties of strings, not 4 properties of json objects
if you want to turn those strings into things your app can deal with, you need to further process them (JObject.Parse, for example)