Method works when dependent Method never called?
I am following a Pluralsight tutorial on ASP.NET Fundamentals and we've just created this hard-coded data repository to work from:
I understand what both of these methods do but I don't understand how the
PiesOfTheWeek
method would currently work as the AllPies
has never been called and not called in any constructor? I would expect PiesOfTheWeek
to return Null when it tries it's Where statement.
Also, shouldn't AllPies
have ()
when it's used in PiesOfTheWeek
?
Thank you in advance!60 Replies
...Thinking out loud here but is
AllPies
actually just a property/field? I don't really understand the =>
, in context I think it's a lambda expression but I've never found a description I understand...
I am being an idiot - PiesOfTheWeek
is a property. I see that now, still not quite sure about AllPies
and the =>
though. šAllPies is actually using alternate syntax for a normal method -- it's creating a new
IEnumerable<Pie>
every time it's referenced, just like a method GetAllPies()
would do
that's why you can call it whenever
it's not stateful, it's a method returning a value
incidentally, for the same reason you could rewrite PiesOfTheWeek like this:
public IEnumerable<Pie> PiesOfTheWeek => AllPies.Where(p => p.IsPieOfTheWeek);
get
ers are really just methods, and =>
properties are also really just methods, so they're interchangeable šThank you! I didn't know a new
IEnumerable<Pie>
would be created every time AllPies
is referenced. Is that unique to Dependancy Injection? I was under the impression in something like a Console App, PiesOfTheWeek
would error trying to return AllPies.Where(...)
that's just a part of the C# language - you're not doing any dependency injection in that code snippet
So
AllPies
is a method and it's being called everytime? But shouldn't it be AllPies()
when it's called?the
()
are not necessary when you use the =>
syntax
the idea is that, from outside the class, these things are indistinguishable from 'normal' properties
which also don't have ()
Oh right ok. This counts as an Expression Lambda?
the
=>
symbol is called a lambda, but this example isn't an expression tree
(more technically, a lambda is only when you use the =>
to create a new method on-the-fly, like you do in .Where()
- but that distinction in not very meaningful)Ok, so this Syntax doesn't count as a Lambda in anyway? I get myself really confused with the
=>
not technically. but most people would refer to it as such
the => is confusing at first but makes sense eventually
in
Where(p => p.IsPieOfTheWeek);
, everything before => are parameters to the method, everything after is the actual methodYeah I can see how it works in Linq queries and anonymous methods but this feels new
yeah, it is
it's a more recent addition to the language
they co-opted the LINQ syntax because they don't like introducing new symbols
under the hood they don't do the same thing
If I see it for a property - Is it only read only? Just looking at this:
https://stackoverflow.com/questions/40282424/what-does-operator-mean-in-a-property-in-c
And the example is:
Translates to this:
correct
those two are equivalent
and because there's no
set
, it's read-onlyBut there's no way to do a
=>
and do a set
right?well
you can do this
Ugh
Now I'm lost again. Lol. Ok.
š
it happens
i'm happy to go into detail on any bits
I'm trying not to waste your time but at the same time I'm struggling.
My understanding up until that last code snippet is that
=>
is used for:
- LINQ
- Anonymous Functions which are only one line/don't really need to be named
- And now, Read Only Properties which don't have a Set
But then you've thrown that last one in which has confused me a bit againjust for reference, LINQ is anonymous functions
that is,
.Where(x => x.IsSomething)
is using an anonymous function for Where()
the last snippet i showed is the 'long-form' way of writing properties
it was the first form added to the language
=> is still the same concept of describing a function, though
set => _myString = value;
means 'run this function every time someone tries to set the value of this property'
the value
keyword stands in for whatever they're trying to set it toSo why not
Syntax may be off, I'm still reasonably new to C# but basically just
{}
?you can do exactly that too
š
So what's the advantage of the
=>
more concise
for the
get
you have to write return as well
c# has a lot of syntax sugar like this -- functionally identical, but just a little tidier or fewer keystrokesSo basically
=>
translates to what is on the right of me is a codeblock but it's only one line
?mhm... not necessarily!
š¢
normally, yes, lambdas are one line
but they can also point at
{ }
blocks
which can contain whatever you like
this is because the { }
block is treated as one statementI haven't seen Action or Invoke yet. š
heh
sorry
No problem, I appreciate your help!
that's basically how you assign a lambda to a variable
which lets you pass them around like anything else
a similar concept
So I can do this:
ah, not quite
public int timesBytwo() x => x * 2;
this would complain because it wouldn't know what 'x' isYeah I was just thinking how come you don't have to say what the data type is š
the correct form would be
public int timesBytwo(int x) => x * 2;
Ok, that makes sense
technically, you always have to say the data type.
it's just that sometimes it can infer what you mean and let you skip it
the proper form of Where, for instance, is
myListOfInts.Where<int>(x => ...);
but because the compiler already knows your list is a List<int> (or whatever), it lets you skip it on the Where
it doesn't have that information in timesByTwo
for your crazyMaths method... if you use a { }
block, you have to go back to using the return
statement
unfortunately
rust lets you omit it, but not c# yet šOk so I'm almost there... But what's the point in using
=>
if you're going to do a multi-line.. Thing?sometimes you need to use a
=>
but want to do something on multiple lines, basically
it's not a common thing
but you can do it
if you have a really complex thing you want to check in a Where, for instance, you can do it there
so you don't have a line stretching out super-longSo in the above examples they should be this?
oh, this is my bad for not catching it
but you aren't allowed the
=> { }
syntax for methods
so instead it'd just be
as normal
also
i'm not sure why you say number is now 8 hereLOL because I'm an idiot.
My bad
Meant to say 4
it's not correct to say this is a read-only field -- it's a method that returns the new value of 4 every time. nothing is ever 'stored'
lol
easily done
Ok so whilst it makes sense, that confuses me with that stackoverflow link I sent earlier:
Isn't
property
technically a method then?yes. all properties are methods
every property is literally just syntax sugar for methods
if you go and look at java code, they don't have this syntax sugar
so they literally have to write out
get_myField()
for everything
it's a streamlining of thatOh! Mindblown.
yep!
š
when you compile your code, it also literally turns properties back into those methods
That little nugget of info has made everything slot into place now I think I get it!
Although two questions
Will still show up in Intellisense/Autocomplete as a Property? Not a method right? If you're not sure no worries I just didn't want to make a whole new project to check.
Why don't you need
()
for the methods when you use =>
E.g
1. yes, it'll show up as a property. that's what missing out the
()
does, if it had them it'd be parsed as a method with zero arguments
2. because the ()
is for listing the parameters to the anonymous method, and in those examples, there are either no parameters or they'd go in the ()
before the =>
to show what i mean...
in this example the anonymous method takes two arguments
and so they're listed in the ()
So really if I'm doing a property I should exclude the
()
and if I'm doing a method I should include it, even if I'm not passing through any arguments, just so intellisense picks it up?yeah
this is where it goes into matters of style a bit - when should something be a property, when should it be a method?
i can explain my feelings on that but it's subjective
also, just noticed
public bool myMethod() => return true;
remember that in this circumstance, you don't need return
Ah, only need return when it's multi-line
Ok Thank you I think this all makes alot more sense
And that explains why in the code I was copying from PluralSight, it wasn't returning a null because it's technically just a method
...Even though the method doesn't have
()
when it's called either - Because it's actually a property which behind the scenes, is a method. š
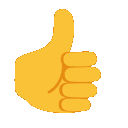
Ahh ok, yeah I find it interesting that I can write code and it works but there's still so many things I don't really know about. It doesn't help when they add loads of new things in each new version - Now you don't even have to use using statements?!
oh totally
it's easier to keep up when you're good with the language, but yeah
if it helps, also, there's never any breaking changes
the new stuff is always just syntax sugars and such
I think that's what I'm trying to say. It's great when you're good with the language and they're introducing things in dribs and drabs but when you're trying to get into the language and everything keeps changing it's really damn hard to keep up with. So many tutorials are out of date so you can easily end up learning the older ways of doing things.
Thank you for all your help, I really appreciate it. Learned a LOT! Thanks
yeah... it is hard. but when you have a 20-year-old language it's a difficult problem to avoid
and no problem! feel free to DM me if you have any more questions
Thank you, I appreciate it š Tomorrow I'll continue with ASP.Net, what a minefield that is
oh god, it is