80 Replies
"We define a three-digit positive integer as "beautiful" if the multiplication of its first digit by its last digit is equal to the square of its second digit."
so my qustion is
am i supposed to just type out a random number between 100 to 999 ?
and then after i do that how am i supposed to do the second part of the assigment
A definition isn't a problem. What is it asking you to do?
We define a three-digit positive integer as "beautiful" if the multiplication of its first digit by its last digit is equal to the square of its second digit.
The program must receive 20 positive integers and check if they are three digits, if so you must check how many "beautiful" numbers there are.
An appropriate message must be displayed.
what i am asking
is where do i even begin this program
how do i defiene an intger as a whole class that needs so many critearies
i need to make sure that its three digits and then that multuplication thing
Yeah, that's ambiguous as to how you should "receive" the data. I would ask for clarification or go based on how input for previous exercises in the same course were done.
i did do something similar to this but this is a whole nother level
i did some stuff with for and count
There's nothing that requires you to write a
class
. This is an exercise in (potentially) string parsing, array/string indexing, and numeric data types.
It's a bit hard to give advice without solving the problem for you.
There are a few approaches: 1. requires the number in both string
and int
form. 2. You can use modulus and division to get the digit.
eg. int secondDigit = (num % 100) / 10;
num1 = num / 100;
num2 = num / 10;
num3=num % 10 / 10;
is this how i am supposed to do the part of dividing
i dont exactly rember how to divide corectly
num 1 is supposed to be the first number from the left
num2 is supposed to be the number in the midle
num 3 is supposed to be on the right
Second needs a modulus.
Try running a some inputs. Like
num = 853;
and see what each result gives you."num2 = num / 10%10;"
like this ?
No.
num%10 ?
I suggest you try checking it instead of guessing.
The compiler and debugger are great teachers.
$debug
Tutorial: Debug C# code - Visual Studio (Windows)
Learn features of the Visual Studio debugger and how to start the debugger, step through code, and inspect data in a C# application.
Demonstrates how to view your app state at runtime instead of wasting time adding in
Console.WriteLine
s to inspect your results.int num, count1 = 0,num1,num2,num3,num4,num5;
for (int i = 1; i <= 20; i++)
{
Console.WriteLine("enter a three digit num");
num = int.Parse(Console.ReadLine());
num1 = num / 100;
num2 = num / 10 % 10;
num3 = num % 10;
num4 = num1 * num3;
num5 = num2 * num2;
}
}
}
}
am i on the right path
(i have checked the dividing part its now corect)
hold on
how do i check if num is a three digit number in the first place
There's multiple ways. You can check the string representation if it's 3 characters in length (assuming the parse passes). You can check the logarithm. You can check if the number is in a certain range.
num/100==0
is this it ?
i tried 100<=num>=999 but it gave me an eror massge
Have you tried it?
Because the last one isn't valid syntax.
but is this working ?
is it coreect
Try it and see?
You don't have to ask a random guy on the internet for something that takes 5 seconds to test.
static void Main(string[] args)
{
int num, count1 = 0,num1,num2,num3,num4,num5;
for (int i = 1; i <= 20; i++)
{
Console.WriteLine("enter a three digit num");
num = int.Parse(Console.ReadLine());
num1 = num / 100;
num2 = num / 10 % 10;
num3 = num % 10;
num4 = num1 * num3;
num5 = num2 * num2;
if (num / 100 == 0 && num4 == num5)
{
count1++;
}
Console.WriteLine("count was equal to=" + count1);
}
what is wrong with this
i tried puting it in but it gives me a zero every time
i put in 222
2 times 2 equals 4
meaning its supposed to give me a count equals 1
but it kept giving me zero
Have you inspected the local state of your variables like the debugging tutorial shows?
what do you mean by that
i know i assigned count1=0
but you have to do it otherwise it wont work
https://docs.microsoft.com/en-us/visualstudio/get-started/csharp/tutorial-debugger?view=vs-2022#set-a-breakpoint-and-start-the-debugger
I mean to actually check your program state instead of guessing.
i am not gusseing i am running it and puting in numbers
Are
num1
through num5
correct right before the if
statement? How can you be sure of that?
Something is wrong between your assumptions and the real program state.and your sure its inbetween num5 and num1 ?
100<=num<=999
Not after actually running it and checking the state. 😛
what is the problem with this statemant
why is it red
num1
though num5
seem fine, so the issue is your if
statement.
Because that's not how inequalities work in C#.
You can't have a compound inequality like that (barring pattern matching, but that's not a beginner concept).
Can you write that as two inequalities and combine them with a logical operator?if (100<=num && num4 == num5&&num<=999)
perfecto
now it works
thenk you for the assinetence
👍
Honestly, take some time to go through the debugger.
It's the most valuable ~15 minutes of programming-related material you'll ever go through.
i still dont understand how to use it
I don't say that lightly.
like i mean litarly how to activate it
Are you using Visual Studio (Community)? Not Visual Studio Code. They're two separate products.
the file is called visual studio 2019
its not called comunity
and its not called code
so i have no idea
Ok, good enough.
So you find the point where you want to examine the program state, then left click in the gutter to set a breakpoint (red dot).
Then run with F5 (Start with Debugging).
Then examine the Locals window (below).
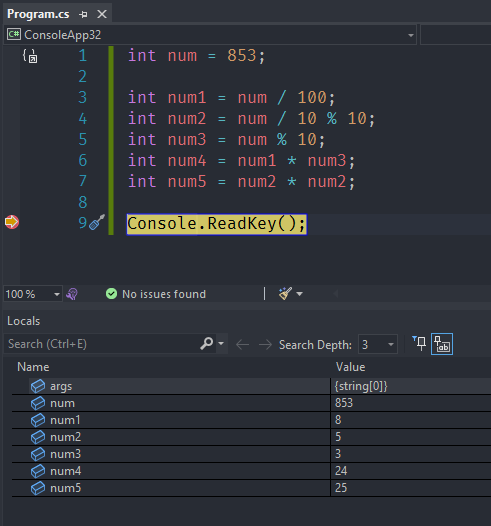
The arrow there is a red dot, normally, when execution isn't stopped there.
It's really helpful to check intermediate results and see the code execution path (with stepping or adding many breakpoints).
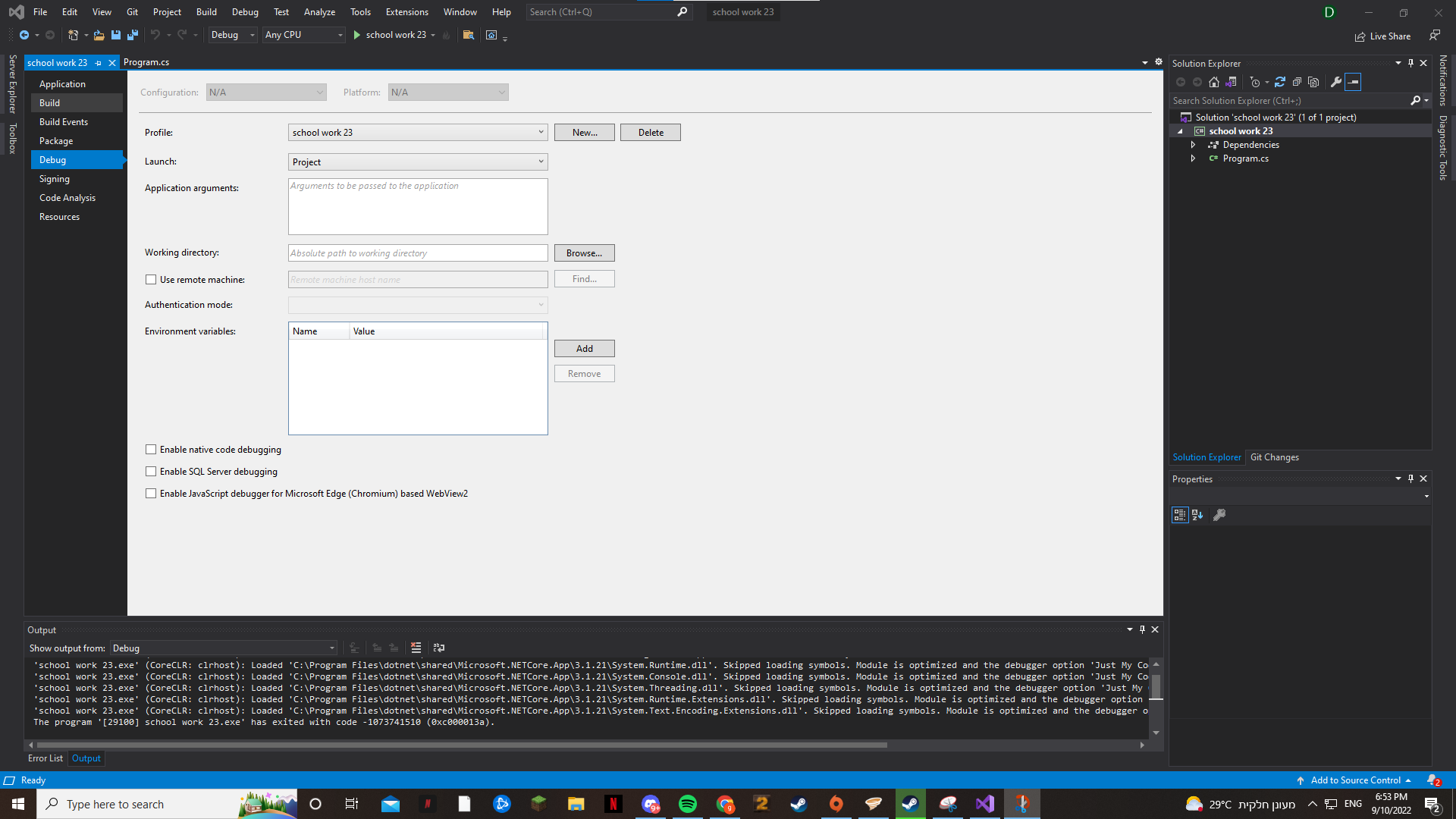
There are other features in the tutorial, too. Breakpoints, stepping, and call stack are the most important.
is this it
No, you don't need to go there.
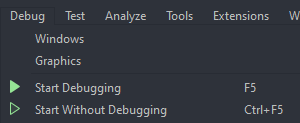
what do you mean by the point where i want to examine the program state
then left click in the gutter to set a breakpoint (red dot).
Say something isn't working how you think it should. Maybe it's a loop. So you put breakpoints before the loop and within, so you can examine the preconditions, and how the loop works each iteration.
left click on what
There's a small bar to the left of the line number that's left clickable.
In VS2022, if you hover, a grey dot shows. I'm not sure if that was there in VS2019.

If I wanted to place one on that line.
i do have that blue bottle but only on certain lines
Not the brush, the dot.
Just keep left clicking to the left from your code until you see red filled circle
i dont understand still how do i enter this debuging mod
i just left clicked
You need to place the breakpoint first, before going into debugging. At least in this scenario.
and it dealted lines of code
Well, undo that. I'm not sure what you did. You're not clicking the right spot.
static void Main(string[] args)
{
int num, count1 = 0,num1,num2,num3,num4,num5;
for (int i = 1; i <= 20; i++)
{
Console.WriteLine("enter a three digit num");
num = int.Parse(Console.ReadLine());
num1 = num / 100;
num2 = num / 10 % 10;
num3 = num % 10;
num4 = num1 * num3;
num5 = num2 * num2;
if (100 <= num && num4 == num5 && num <= 999)
{
Console.WriteLine("count was equal to=" + count1);
}
} now its not working
} now its not working
The breakpoint?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
what breakpoint
what does it even look like
A literal red dot after you click.
Which is what the tutorial I linked before showed, too.
i dont have that
how do i make the program work agien
i litarly have the same exact code and its not working
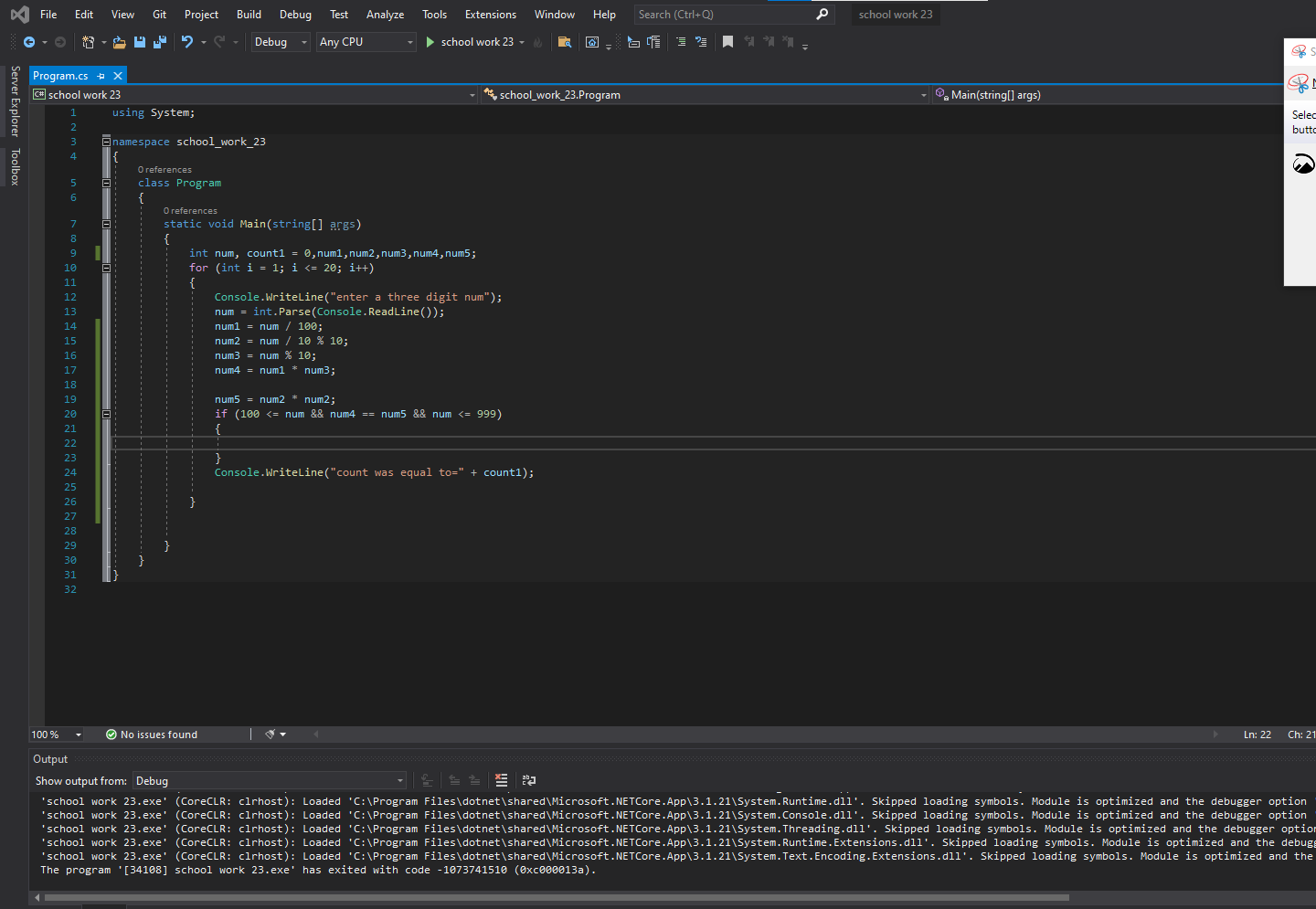
what is wrong with the program
it worked just fine before i left clicked on the side
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
You clicked the part that cut lines. I don't know why you didn't just undo.
man
i cant undo
i dont know how to undo
You have nothing in your
if
condition now.
If you actually read your own code.Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
This area here with the red line should be the gutter.
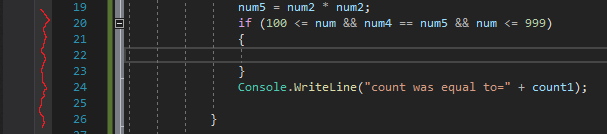
what
that dosent make sense
i can clearly see its filled with words
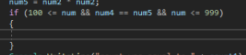
and leters
It executes nothing.
i tried placing the command isnide
Because there's nothing in the body.
oh
yeah it works now
thx
Anyways, you can start a new project to play around with the debugger.