.NET Program that runs in the background [Answered]
How would I make an .exe program in c# .NET that runs in the background (It will have a tray icon instead). It will be used for capturing certain hotkey presses
32 Replies
What does it do?
And is the tray icon required (may significantly increase complexity)?
The program will help me copy and paste things easily. It will be set up to capture a set of hotkeys for example CMD+G+1-9 (I need help figuring out how to recognize the inputs) and for each number will store highlighted text (again something that I need help with) and will have an associated paste function. This project is very non-concrete and I’m still in the research phase so I may scrap it if this is too hard to do. Another idea would be when CMD+c is pressed, it use the next keystroke (1-9) to store the data copied from CMD+c. It would be much, much easier to just make it a vscode extension, but before I resort to that I would like to see if I could make something that works on other programs like VS too. The tray icon is not required at all and just something to easily kill the program. If it will make it too complex, I don’t have to include it
Ah, that actually clarifies it a good amount. I was initially thinking of a Worker Services app, but that no longer seems appropriate since those have some limitations
You could make an invisible WPF app potentially, since that should let you interact with tray icons and the clipboard
That sounds like a good idea (especially because I’ve worked with wpf before). How would I have a WPF app the doesn’t have any content
Itbwould also be nice if it runs in the background/doesn’t have a tab on my pc
For the tray icon you can use the
Hardcodet.Wpf.TaskbarNotification
nuget. That's what I use👍
In the App xaml you can do something like this
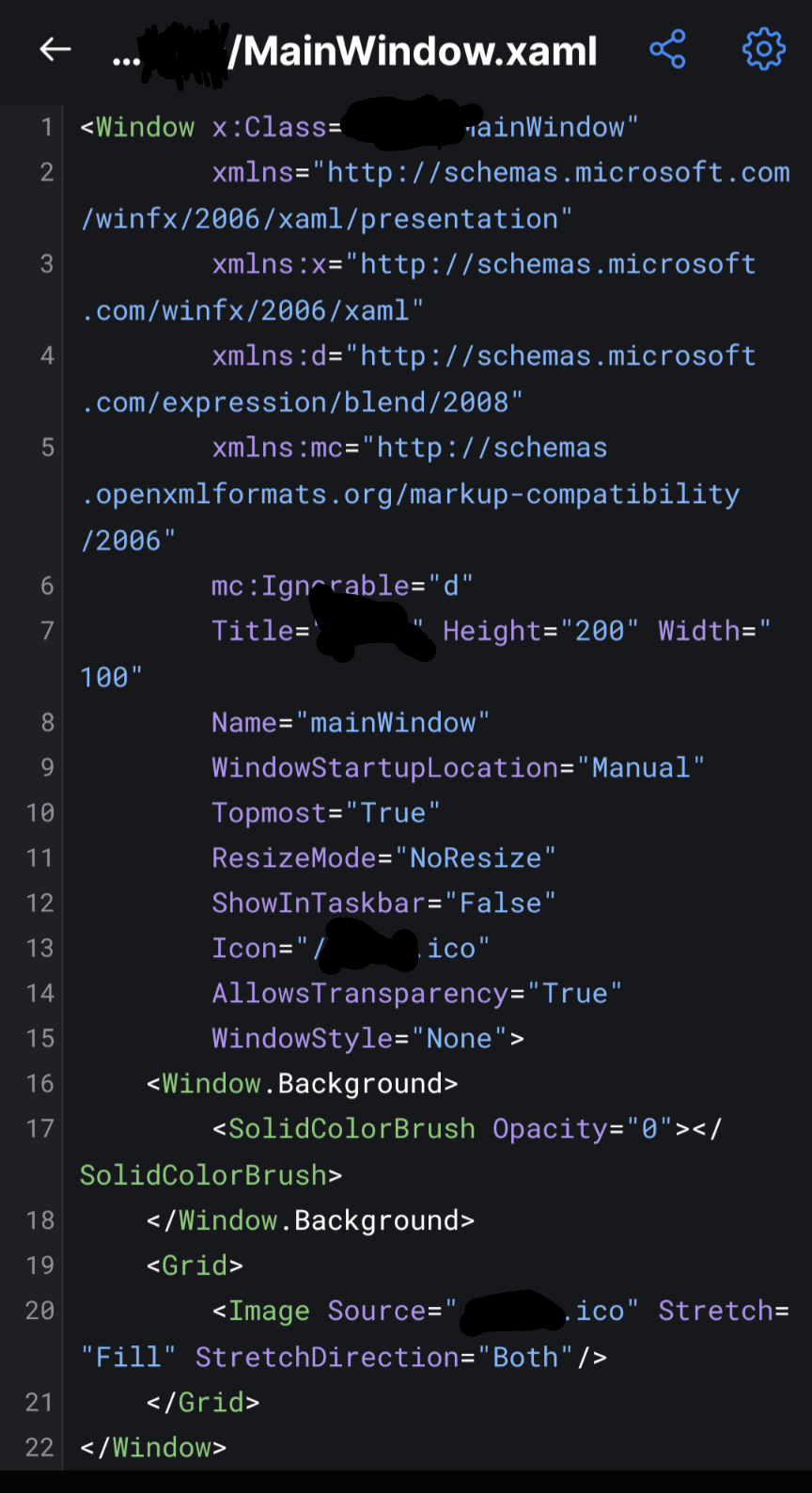
Some of those tags may not be required. I did something similar for a notification popup app
Ok
Thanks
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
you can PInvoke the
RegisterHotKey
function i think that should work
i see you said in another thread you didnt get it to work?I just use
Win + V
¯\_(ツ)_/¯So I have copying selected text working and registering hotkeys with the RegisterHotKey function, but I would have to make 10 RegisterHotKey functions, so Is there a better way to do it? (maybe register if any of the ALT+0-9 keys pressed with a value signifying which one it is)
like some way to get a general 0-9 key as the last param of RegisterHotKey and read it using HwndHook (((int)lParam >> 16) & 0xFFFF)
@The Court Jester ^
@Ezlanding you could use global raw input, then you get all the key presses
basically a keylogger
but its a lot of work to the the interop for that yourself
Can you share a Microsoft docs page on it?
Raw Input Overview - Win32 apps
This topic discusses user-input from devices such as joysticks, touch screens, and microphones.
Thanks
@Ezlanding i can give you some c# code i wrote for this
That would be good
Thanks
Thanks
What’s the unsafe modifier?
im using pointers so the code is "unsafe"
K
RAWINPUT*
has to point to a struct on the stack or a pinned / fixed array
i use the CsWin32 nuget package to do the interop for me, or you could use TerraFXSo this writes the currently pressed to key to out_raw_input?
yes
you call
WriteRawInputToBuffer
on the WM_INPUT
event👍
@The Court Jester I am getting some errors like
The type or namespace name 'RAWINPUTHEADER' could not be found
also how excacly do I use this function? will it block the character or only capture the input?it doesnt block the keys from reaching where they are going
i cant help much rn
that may be a little bit of a dealbreaker as it will overwrite the selected text
is it ok to have 14 registerhotkey functions?
its not possible to block key input or at least not easely
i dont think it matters
k then Ill use a whole bunch of registerhotkeys
✅ This post has been marked as answered!