Enable button from another Form [Answered]
there is
-Main menu
then 3 subforms to main menu
-A, B, C
I want to enable Main Menu button when i click a button in form A or B or C
and the code that moves user through forms
104 Replies
first you need to have a reference of your Main Menu form in your child forms
well is it gonna be a problem if it's opposite?
what do u mean?
the menu is a reference to subforms
like this is menu
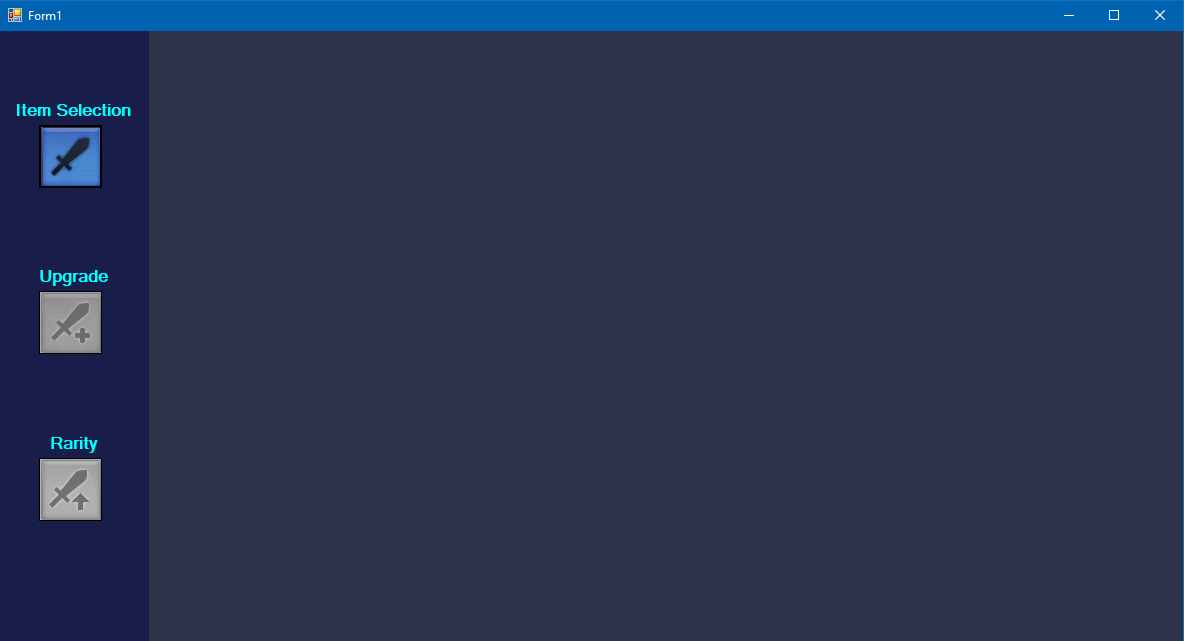
and these buttons take you to forms A B C
so just the right side changes and the left with buttons is always the same
no matter in which form you are
yea
you can atleast pass a reference of your main form to ur child form through a method
u can do whatever this method needs to do, like enabling a button
oh so it's that simple
yes, u can make the method take the argument and assign it to a field of type MainMenuForm
or whatever you called it
then in whatever button you want to click in your childform, just do the logic to enable the Main Menu Button
looks like you call it Form1, so lemme juste dit
it's actually myMain
i f up some names there
it would get ugly if you repeat the same code i wrote on the 3 forms
so let the 3 forms atleast inherit from another form that has the logic
i nee to fix the naming 1st XD
Error CS0122 'MainMenu.btnUpgrade' is inaccessible due to its protection level
so the button needs public modifiers?
never make field public
just define a public property that returns that field
its not recommended to access a button from another class,
better define a method and call it something like
EnableMainMenuButton
same error
let me edit the post above
can you show what you wrote?
is your method public?
and the child form
the 3 forms inherit from ItemSelectionMenu right?
yea
w8 no
from main menu
the MainMenu is the one who should have the method
main menu is like background form with just the 3 button on the left
because only the MainMenu class can access its private members
you cannot access a private member from another class
what class do the child forms inherit from?
MainMenu
why would you do that?
i don't want the user to be albe to go anywhere
he has to go to 1st menu
then 2nd
then 3rd
what do u mean?
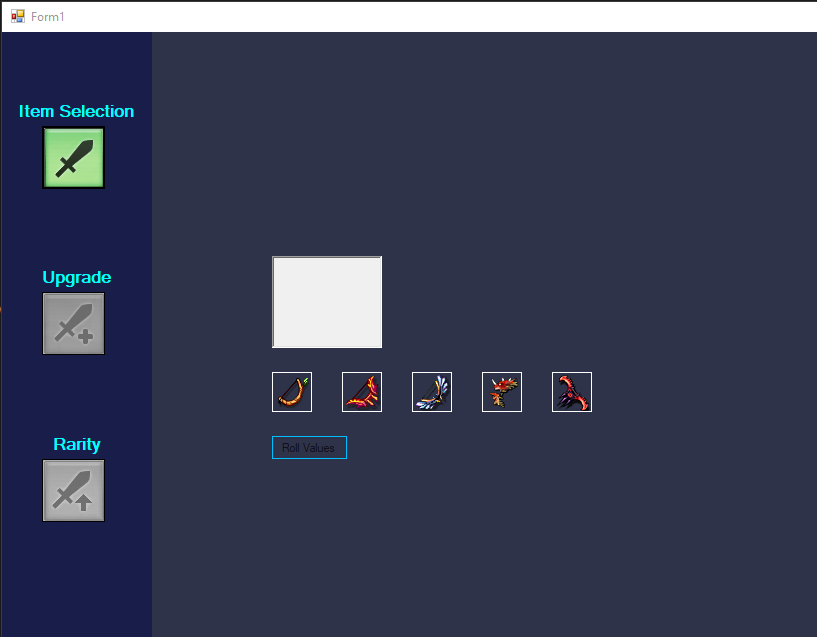
so you have to go to Item Selection
and select item
then you can go Upgrade it
this wont work
since childform is not a type of ItemSelectionMenu, or does not extend it
and only then u can go to rarity
the is operator returns true when the type of the provided variable is of that type, or inherits from it
and if its true, it will create a new variable of type ItemSelectionMenu called itemselectmenu and assigns the value from childForm to it
what are you doing anyway, a game?
i wanted at first
but then decided just to do some 4fun upgrade simulation
i need anything on github that proves that i do like anything connected to coding
to even get an internship or sth
my 3rd year of college starts soon D:
and we learned basically nothing there
what i learned from doing this app is like 10x more than in college
cool
so i passed a boolean through other class menu
but nto i need to click the button again to refresh it
so button upgrade enables
this is the button that should enable the menu button Upgrade
its a private method, so u cant access it outside its class
ah yes nvm i tought it worked then it crashed the app
Error CS0120 An object reference is required for the non-static field, method, or property 'MainMenu.EnableButtons()'
what is MainMenu anyway
a form
Form1 or the sidebar with the 3 buttons on the left?
form1
it's sidebar + background for other forms
you need a reference of your current MainMenu instance
ur accessing EnableButtons as if its a static method, when it isnt
even if you did make it static, you can't access its controls because they are not static
how do i do that reference
what did you try?
where are u trying to call EnableButtons anyway?
because i feel ur just copying code without understanding it
in the button that should enable it
in which class?
ItemSelectionMenu.cs
can you show code?
thats not really a class, thats just a file name
a file can contain many classes
a file's name can even be different from the class name defined inside the file
yeah buti keep it simple
new class = new file
EnableButtons isnt a static method
and u cant make it static because forms arent static
yeah i understand that
you need a reference of your MainMenu instance assigned in a field of your class
_mainMenu.EnableButtons();
for example
will call the method from the instance of your current MainMenu
that is assigned in _mainMenu
I typically do this too, unless I need Extension methods on an enum
Or its a factory
but then you need to figure out how to assign the reference of your current
MainMenu
instance to your fieldim too green to even understand what u just said
so i have this field in UpgradeMenu
private MainMenu _mainMenu;
and this is the method in Main Menu
but when i run it i get "object reference not se to an object"
because your field is null
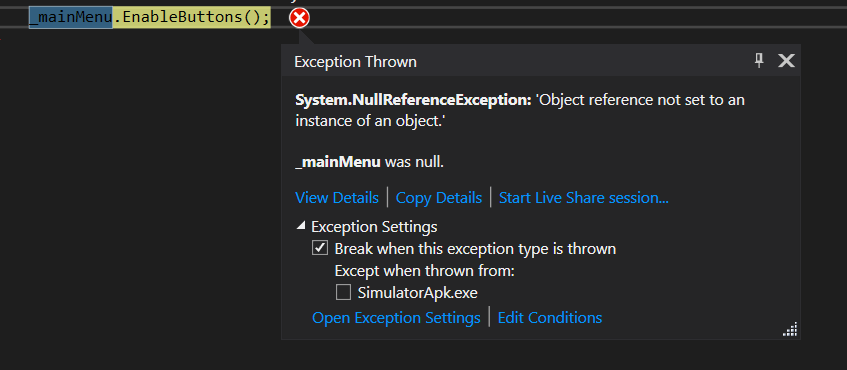
its null, it has no value
you need to find a reference of your current MainMenu Form and assign to it
Probably by passing this through a constructor
how? you can either pass through a method, through a constructor, or any other many ways
you can pass through the constructor, the recommended way
so i have the constructor
yeah that
and it should be where it is
in ItemSelectMenu
not main menu
right?
if you are calling
new ItemSelectMenu
from your MainMenu
use new ItemSelectMenu(this)
where this
is a reference to your current MainMenu
instancebut i am not? ;-;
where are you calling it?
i don't quite understand your question
this is how i open another forms
yes but where are u calling
OpenChildForm
there it is
where is this defined?
in MainMenu
exactly
so do
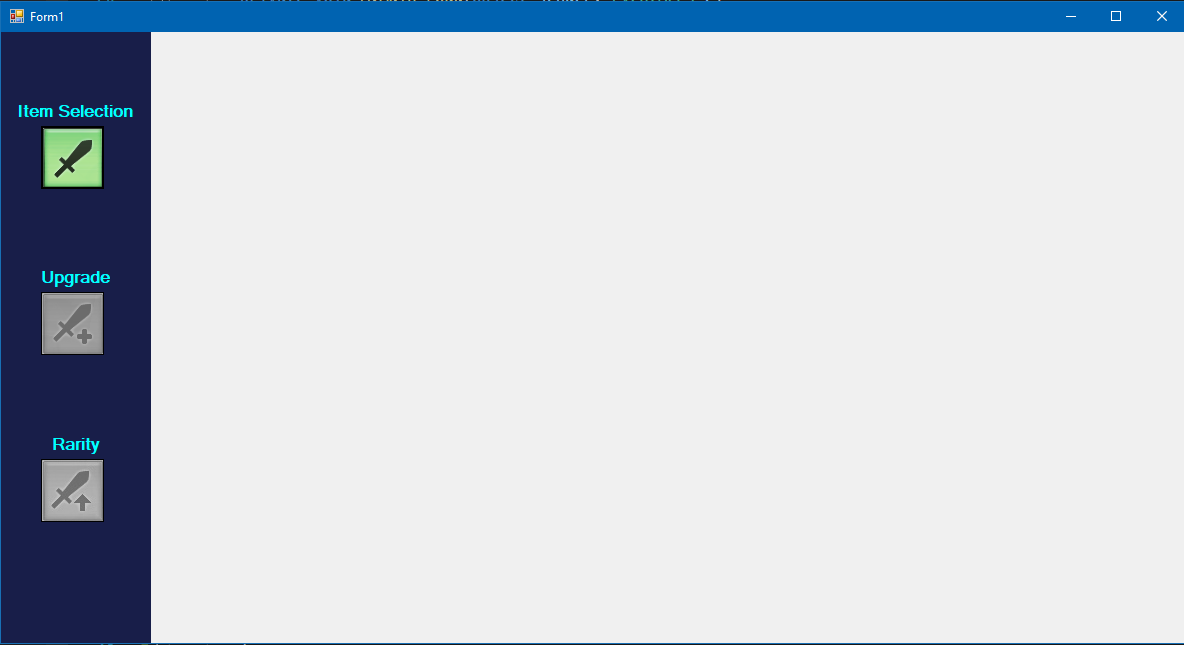
this is not what i expected XD
what is?
i clicked the button
what button
this
weird, did u change something?
other than passing the reference of ur mainmenu to ur constructor
hmm
this in main menu
the constructor is the same
nah i don't know
what is btnUpgrade
the button that i want to enable
comment btnUpgrade.Enabled = true; and see what happens
//btnUpgrade.Enabled = true;
same thing
goes blank
issue is somewhere else probably
i removed "this" from the call of OpenChildForm
and it doesn't go blank
ah then you have 2 constructors in your ItemSelectionMenu()
the other one initializes your components
ah yea
you should only have 1 constructor
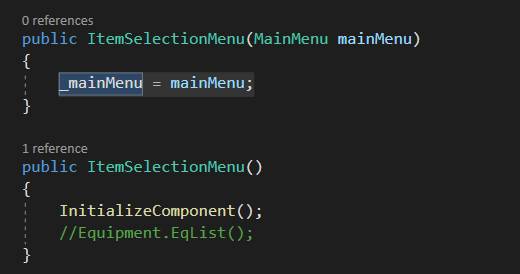
yes, InitializeComponent method needs to be called
the other constructor doesnt
ayy
works
as it should
and ur btnUpgrade got enabled?
yes
cool
thank you
so in the end error was two same constructors xD
✅ This post has been marked as answered!
those 2 constructors arent the same
well yes but it this caused error
because the OpenChildForm never called the 1st one
1 constructor can inherit from another
or didn't call InitializeComponent()
so menu never loaded
public ItemSelectionMenu(MainMenu mainMenu) : this()
this will call the other constructor first then calls its own
in case you want to add more logic that you dont want to repeat in the othermakes sense
ty again