I want on a click of a button in form1 to change a thing in 2nd form, but can't manage [Answered]
So i have 4 forms - Main menu - then 3 subForms referenced to that. In form1 i want to click button so in form2 a picturebox will take an image from form1 and load it there to form2
56 Replies
you need to pass the image into the constructor of form 2
i don't know where exactly that'd be
thats the second form right?
yea
so u need something like this
and in the 1st form i need this image?
how do u create the second form in the first one?
ah i see problem now, it wants to take the image from main menu
not from form1
form1 is not the main menu?
no
there is Main menu then 3 forms (ItemSelectionMenu, ItemUpgradeMenu, ItemRarityMenu)
main menu is like just background
i can show u on stream if it helps
nah i think i understand
so when form 1 is running and u click the button, is form 2 also running?
i guess
umm that will be bit harder
ok so u want to have form shown event in the second form
yes
and there u need something like this:
Than u need SharedClass which is static class with public image property
and in the form1 u need something like this:
In the form i take image from right?
yes
Error CS1061 'Image' does not contain a definition for 'GetAwaiter' and no accessible extension method 'GetAwaiter' accepting a first argument of type 'Image' could be found (are you missing a using directive or an assembly reference?) SimulatorApk C:\Users\Admin\Desktop\Games\SimulatorApk\SimulatorApk\ItemUpgradeMenu.cs 22 Active
ah yep my bad
its
async Image GetImage()
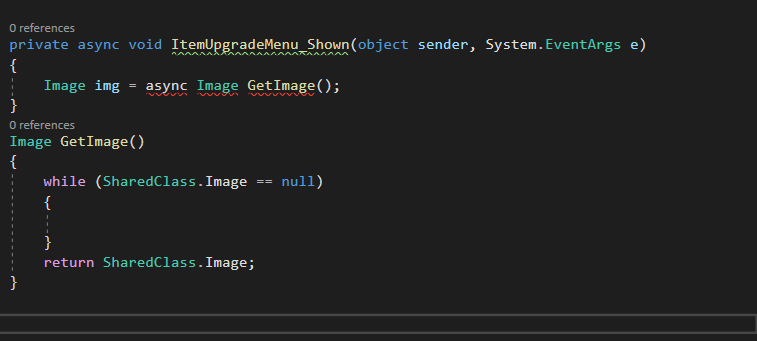
it made it worse or im dumb
wron line
its the method declaration not the call
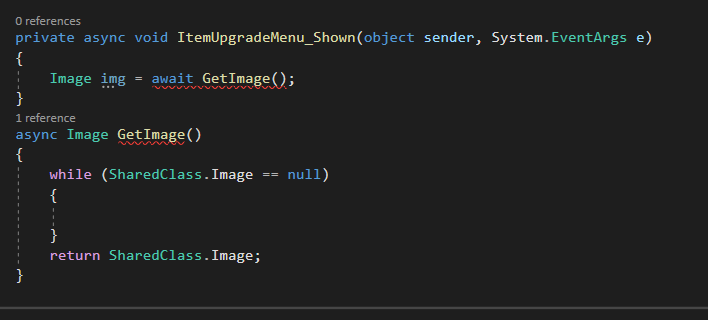
also you didn't register the shown event
ah shit wait
its
async Task<Image> GetImage()
i think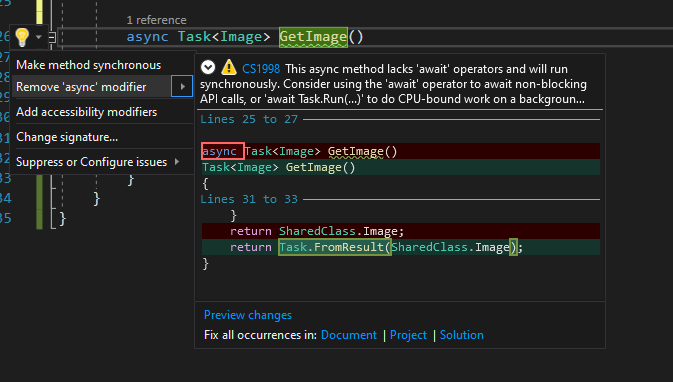
it either wants it synchronous
theres someone dumb here, ither its me or visual studio
XDDD
just leave it as it is for now, i think it should work as i want it to
one more error i think i couldn't fix rn
Error CS0200 Property or indexer 'SharedClass.Image' cannot be assigned to -- it is read only SimulatorApk C:\Users\Admin\Desktop\Games\SimulatorApk\SimulatorApk\ItemSelectionMenu.cs 46 Active
you are missing setter
also you can just one line it
public static Image image {get;set;}
and i assign wanted image in shared class on in the form UpgradeMenu?
?
i mean thsi to the button
but it doesn
work
what doesn't work? i need more info
so like this
has 0 ref
what do i do with it
you forgot to register the event
yea but where?
one the upgradeMenu isuppose
but there are so many events
the event is called form shown
i think
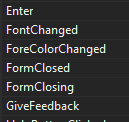
ah its called just
Shown
still not working and i have this error in main menu
Error CS7036 There is no argument given that corresponds to the required formal parameter 'img' of 'ItemUpgradeMenu.ItemUpgradeMenu(Image)' SimulatorApk C:\Users\Admin\Desktop\Games\SimulatorApk\SimulatorApk\MyMain.cs 50 Active
it looks like it still wants the image from main menu
idk
š¦
oh yep that was the original idea, just take the original methods you had there
i mean i didn't change a thing in main menu
it should look like this now
the second form should look like this now
oh yeah
thnak you very much man
u saved me a week of googling stuff
np
does it work well now?
yea!
nice
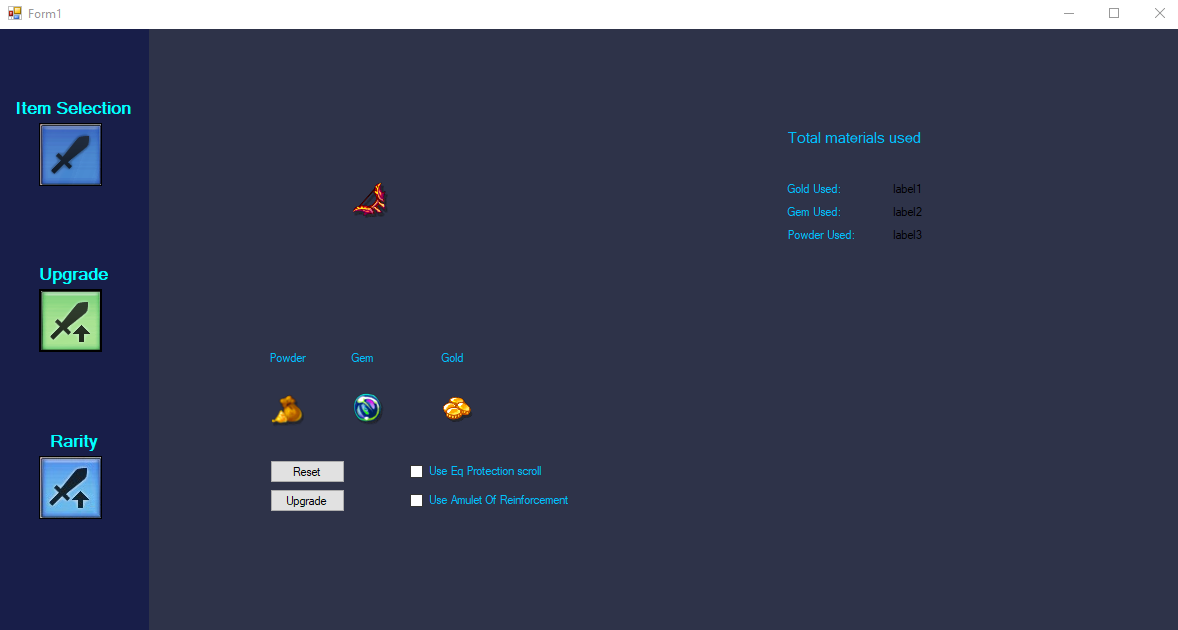
oh that looks really cool
is there github repo for the project?
not yet
i want to make
but didn't quite think of it yet
idk why xd
didn't expect to do this much actually
tbh visual studio support for github is awsome
i was using Rider b4
but something fucked up really hard
and i chanegd to VS like 2 days ago
so VS is giga new for me
it's like my 2nd month of programming
well you learned a lot i guess
if you click view there is git changes button and that just gives you full git support inside vs
@Whiteboy don't forget to close the thread
ā
This post has been marked as answered!