Object null when using the same action controller
I have my GetById Action controller giving the result like this when request directly from it notice that the Breed not null (the first image)
And I have the Create Action Controller which basically using the GetById to give me the animal using the below return
And the second picture is the result I got which is the breed completely null
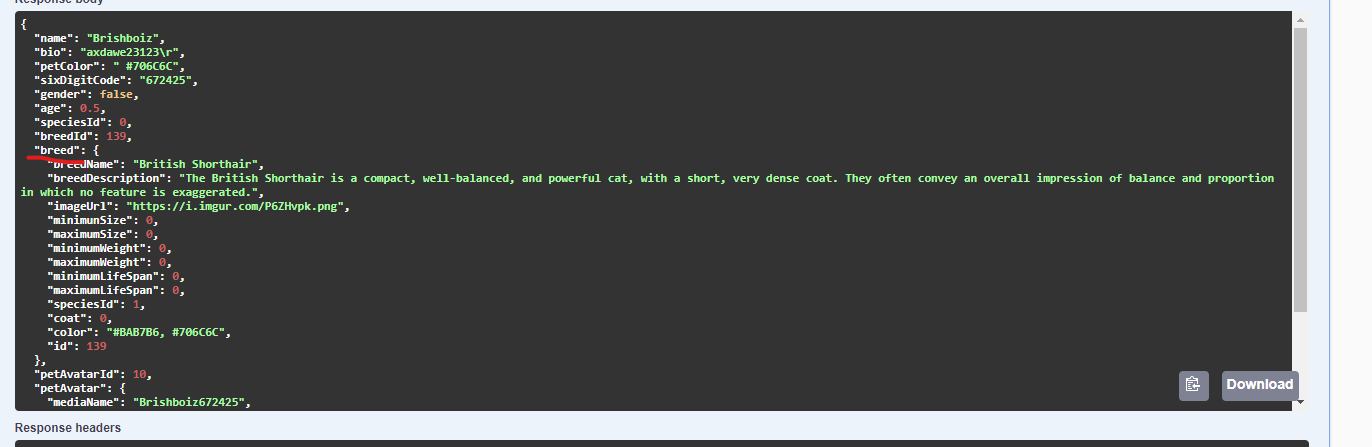
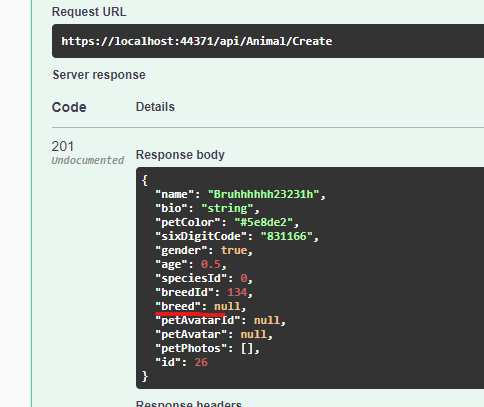
44 Replies
You need to
.Include()
related models
Or better yet, .Select()
the whole thing into a DTO
So, a fast way:
and a proper way:
Also, reconsider using repositories altogether
From what I see, your repository doesn't do anything EF wouldn't already be doingon the method that use return CreatedAtAction ? or the FindById ?
Where do you fetch the animal from the database?
There
findbyid
let me give you the full code
My answer stays the same
Wherever you fetch the animal and need its breed, you need to
.Include()
or .Select()
it
but FindByIdAsync does include the Breed

And when call directly to it do give me the breed
Maybe your mapper doesn't map it, then
The problem is why other method use
return CreatedAtAction(nameof(GetById), new { Id = animal.Id }, _mapper.Map<AnimalDTO>(animal));
doesn't include the breedDunno, I loathe the repository pattern and I don't use the automapper
What's the full method that returns it?
Seems to me like this
animal
might not have the breed includedHope you can read it
it's ugly I know
I trying to get better in writing cleaner code
You seem to be setting the
BreedId
of the animal
not the Breed
of it?They both return
animal.BreedId = breed.Id;
Yeah, but you use different ways to get that animal
there
In the first method you use a repository, in the second you use some other demented repository-thing
After the animal.BreedId = breed.Id;
line add animal.Breed = breed
See if that helpsOkay I will put that after the SaveChanges()
Maybe?
Idk
Repositories are a mess
because EF will create another breed if put it before the savechange
Fuck repositories
All my homies hate repositories
what's your pattern ?
can you link me the blog or docs to implment your style ?
CQRS and services where needed
services ...
Yes
Instead of duplicating the functionality that
DbSet
already gives you for free, you create specific methodsdo you have a simple project repo ?
Afraid not
cqrs
heard that many
but never understand it
But the idea is that instead of
which is just a useless abstraction over EF, you do
etc
The idea behind services
is Unit Of Work also a service ?
woah you totally right
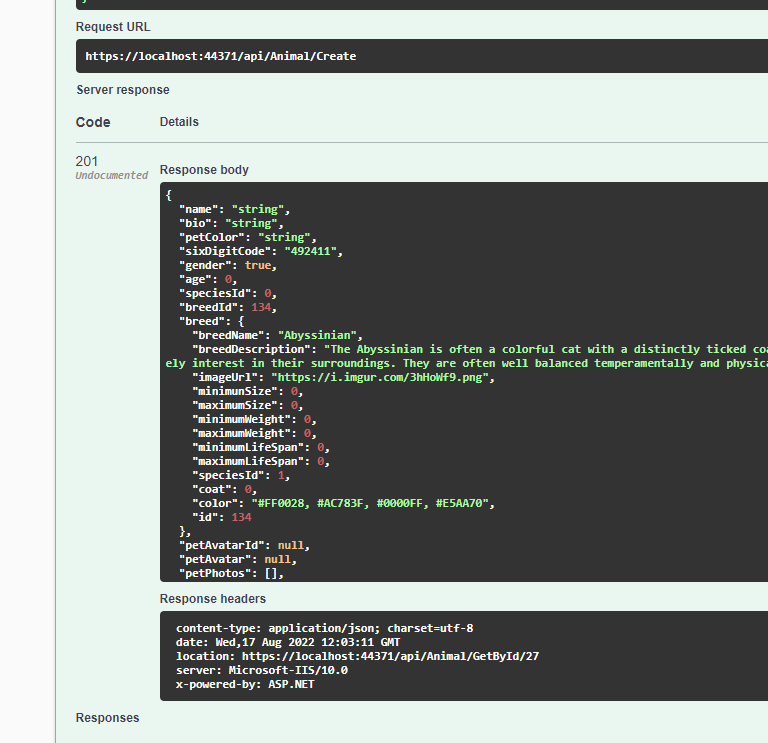
EF already implements UoW
aww man CreateAtAction suck
I thought it just route the action and all the behavior stay the same
Nope, it doesn't call the actual action
Just constructs the URL and returns some data
but aint it suppose to do the same ?
just pass the parameter call the right route and define what it need to to return ?
If have some additional logic inside GetById. It will not work ?
¯\_(ツ)_/¯
Here's a CQRS sample from one of my projects: https://github.com/Atulin/CQRS-Sample
Press the
.
key when you open the repo in the browser so you can browse the code more easilydid you just create a freaking repo for like 5 minutes ?
Yeah, why?
git init && git add . && git commit -am "init"
already gets you halfway there lolyou re freaking beast
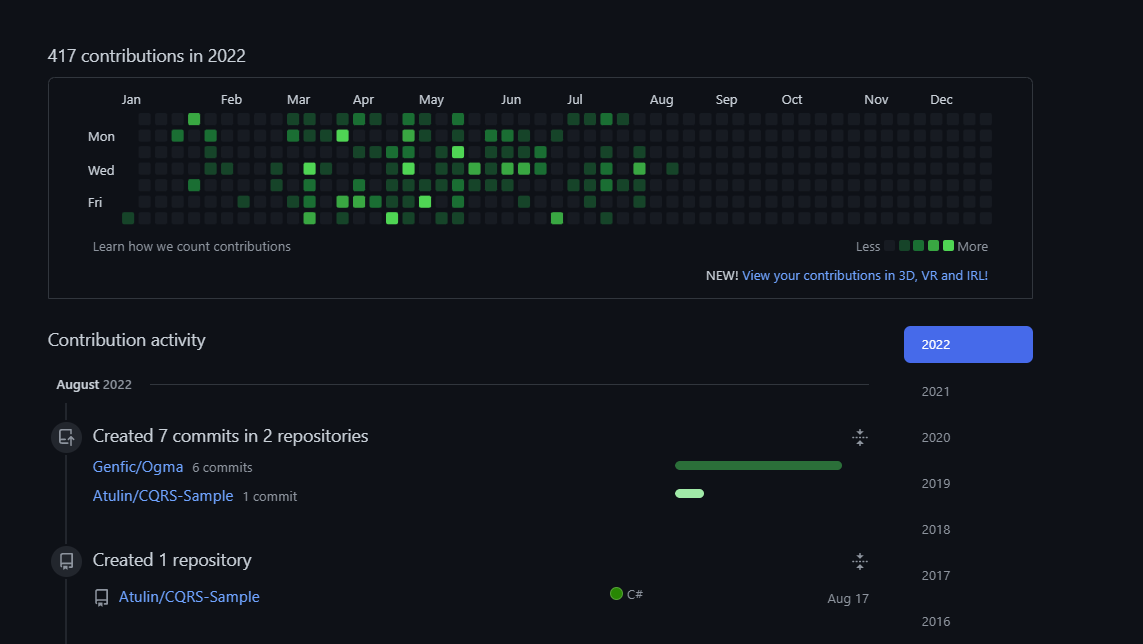
Ah, the
BaseHandler
might be a bit confusing, but all it is is just provides some shims to handle returns types easier. It's just a bunch of
methodsyou mean the return
CreatedAtAction(nameof(GetById), new { Id = animal.Id }, _mapper.Map<AnimalDTO>(animal));
?Nope, in the example code I sent you
All the
Handler
classes inherit from BaseHandler
that isn't a standard MediatR class, thought I'll clarifyokay let me see
Mediator
is that cqrs thingy ?
It's a library that makes CQRS easier
Very commonly used. In fact, I don't know anybody who wouldn't use it for CQRS lol
ohhhhhhhhhhhhhhh
I see now
say thanks for helping me all these time
can I have friend request ?
You can ping me if you need help