[Bug?] gremlinpython is hanged up or not recovering connection after connection error has occurred
Vertex hashmaps
aggregate()
is probably your best approach. in java, you would probably prefer subgraph()
because it gives you a Graph
representation which you could in turn run Gremlin on and as a result is quite convenient. we hope to see better support for subgraph()
in javascript (and other language variants) in future releases.Benchmarking
How to improve Performance using MergeV and MergeE?
g.mergeV([(id): 'vertex1'].option(onCreate, [(label): 'Person', 'property1': 'value1', 'updated_at': 'value2']).option(onMatch, ['updated_at': 'value2'])).mergeV([(id): 'vertex2'].option(onCreate, [(label): 'Person', 'property1': 'value1', 'updated_at': 'value2']).option(onMatch, ['updated_at': 'value2'])).mergeV([(id): 'vertex3'].option(onCreate, [(label): 'Person', 'property1': 'value1', 'updated_at': 'value2']).option(onMatch, ['updated_at': 'value2']))
So I'm send 2 requests to neptune. The first one with 11 vertexes and the second with 10 edges in two different requests and doing a performance test using neptune. The duration of the process for this amount of content is like 200ms-500ms. Is there a way to improve this query to be faster? For connection I'm using gremlin = client.Client(neptune_url, 'g', transport_factory=lambda: AiohttpTransport(call_from_event_loop=True), message_serializer=serialier.GraphSONMessageSerializer())
so I send this query by gremlini.submit(query)
...addV()
, addE()
, property()
steps will be faster than using mergeV()
or mergeE()
. The latter can also incur more deadlocks (exposed in Neptune as ConcurrentModificationExceptions
). So it is also good practice to implement exponential backoff and retries whenever doing parallel writes into Neptune....Why is T.label immutable and do we have to create a new node to change a label?
Why is T.label immutablei'm not sure there's a particular reason except to say that many graphs have not allowed that functionality so TinkerPop hasn't offered a way to do it.
and do we have to create a new node to change a label?...
Simple question about printing vertex labels
g.V(0L)
in Gremlin Console to return the vertex that you created.Defining Hypergraphs
One possible generalization of a hypergraph is to allow edges to point at other edges. There are two variations of this generalization. In one, the edges consist not only of a set of vertices, but may also contain subsets of vertices, subsets of subsets of vertices and so on ad infinitum. In essence, every edge is just an internal node of a tree or directed acyclic graph, and vertices are the leaf nodes. A hypergraph is then just a collection of trees with common, shared nodes (that is, a given internal node or leaf may occur in several different trees). Conversely, every collection of trees can be understood as this generalized hypergraph....
JanusGraph AdjacentVertex Optimization
explain
I get the following output.
```
Original Traversal [GraphStep(vertex,[]), HasStep([plabel.eq(Person)])@[a], VertexStep(OUT,vert...Efficient degree computation for traversals of big graphs
profile()
step in Gremlin is different than the Neptune Profile API. The latter is going to provide a great deal more info, including whether or not the entire query is being optimized by Neptune:
https://docs.aws.amazon.com/neptune/latest/userguide/gremlin-profile-api.html
If you have 10s of millions of nodes, you could use Neptune Analytics to do the degree calculations. Then extract the degree properties from NA, delete the NA graph, and bulk load those values back into NDB. We're working to make this round-trip process more seamless. But it isn't too hard to automate in the current form....Gremlin query to order vertices with some locked to specific positions
Product
vertices...Is it possible to configure SSL with PEM certificate types?
keyStoreType
and trustStoreType
either JKS or PKCS12 format: https://tinkerpop.apache.org/javadocs/current/full/org/apache/tinkerpop/gremlin/server/Settings.SslSettings.html
Is this true? Is there any way for us to configure SSL with PEM format certificates?...Query works when executed in console but not in javascript
Very slow regex query (AWS Neptune)
TextP.regex()
expression. We are observing very bad query performance; even after several other optimizations, it still takes 20-45 seconds, often timing out.
Simplified query:
```
g.V()...To get a performant query for partial text matches the suggestion is to use the Full Text search integration (https://docs.aws.amazon.com/neptune/latest/userguide/full-text-search.html) , which will integrate with OpenSearch to provide robust full text searching capabilities within a Gremlin query...
How can we extract values only
g.V().project('Latitude', 'Longitude').by('lat').by('lon').math(...)
. You would replace the ... in the math() step with the Haversine formula.
2. If you want to keep these as two separate queries, then you should use one of the Gremlin Languages Variants (GLVs) which are essentially drivers that will automatically deserialize the result into the appropriate type so you don't have to deal with the GraphSON (which is what your initial post shows). Read triggan's answer above for more details about that....How to speed up gremlin query
I read that I should create a composite index to improve speed and performance or something of that sort, but I am unfamiliar with how to do that in Python.as a point of clarification around indices, you wouldn't likely do that step with python. you typically use gremlinpython just to write Gremlin. For index management, you need to use JanusGraph's APIs directly. often those are just commands you would execute directly in Gremlin Console against a JanusGraph instance....
logging and alerting inside a gremlin step
Shell flight created for paxKey ${paxKey} with flightId ${flightLegID}
))
...Optimizing connection between Python API (FastAPI) and Neptune
## Breadth-First Traversal Fact Check
repeat()
is executed via the query hint as noted here: https://docs.aws.amazon.com/neptune/latest/userguide/gremlin-query-hints-repeatMode.htmlHow to find the edges of a node that have a weight of x or greater?
g.V().has("person", "name", "A").out(...
returns Vertices
to get edges need to use outE()
something like
a_friends = g.V().has("person", "name", "A").outE("is friends with").has("weight", P.gt(0.75)).inV().to_list()
...op_traversal P98 Spikes
fold().coalesce(unfold(),...)
style "get or create" based vertex mutations....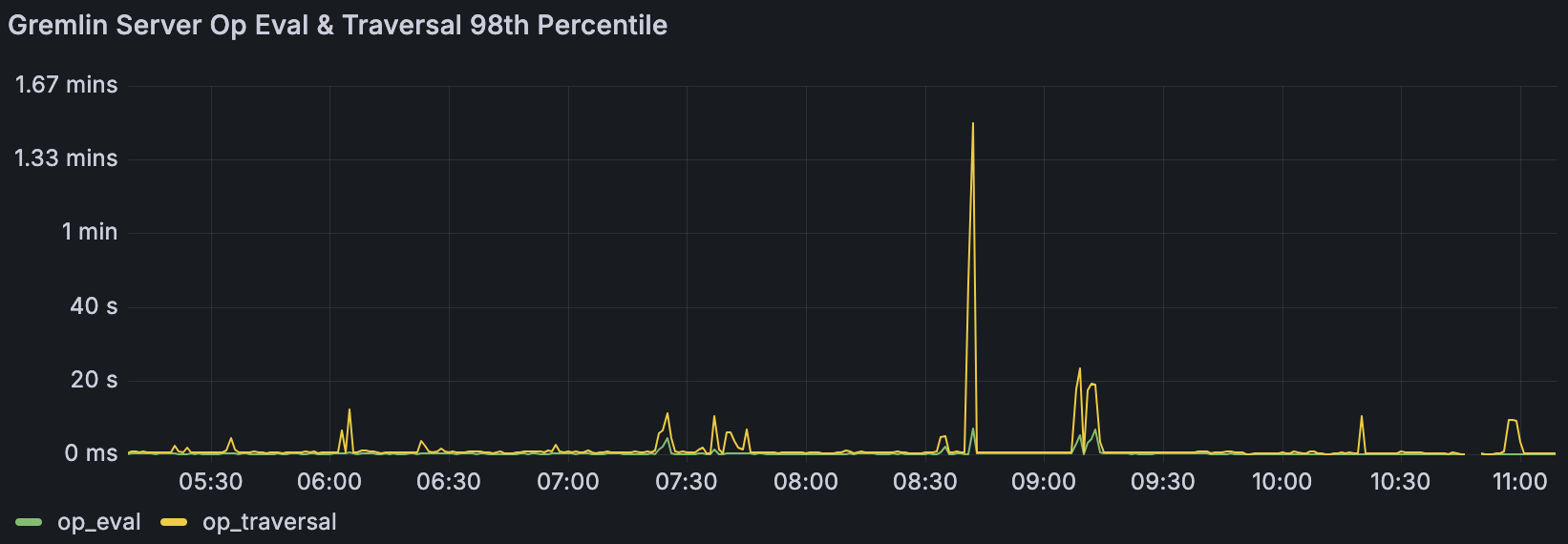