Actor Updating
question for ya'll, simple update issue i'm seeing that i'm sure someone understands. with my convenient effects module, i'm attempting to handle the spell Aid, which grants +5 healing and +5 max HP for the duration. i'm writing code to specifically do this, because active effects for this kind of stuff doesn't work. here's what i got
This all works well and dandy... unless foundry is reloaded. Then all the changes are lost and the max HP is set back to whatever it was before. I'm passing in the Actor5e from the selected token on the canvas.
Anyone have any ideas?
37 Replies
Suspect you should be calling
actor.update
instead of actor.data.update
will that effect the 'prototype' version if applied to a derived token? also, is the syntax for that the same as what i'm doing just without the .data?
Ohhh I have no idea how to handle unlinked tokens
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
I wanna say "no" to the first question
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
hm let me try this and see. also, yay threads. long overdue feature
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
oh, not on mobile, so haven't had that experience yet lol
alright that is not doing anything
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
ah, it's not data.data. i guess it is still just data
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
huh, looks like it does. the unlinked token is affected, but not the prototype version of it
sweet, i think this is good now. i knew it had to be something simple and i just didn't know it
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
so in summary
that works for all linked and unlinked tokens
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
dang it
wait
oh
lol. vim and copying. my b
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
Yeah you're trying to update
data.attributes
with the value from actor.data.data.attributes
updated it
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
👏 thanks all
wait if i thank you in a thread, does it still give league points?
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
also, can i thank you all at once 🤔
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
lets try. thank you @theripper93 @calego @nekrodarkmoon
@dfreds gave
LeaguePoints™ to @theripper93 (#8 • 182), @calego (#1 • 1008), and @nekrodarkmoon (#27 • 65)
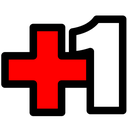
woohoo
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
you get a lot of
in your #progress-reports 😉
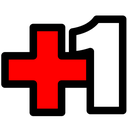
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
speedrun to take over calego ready go
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
lol
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View
😓 I'm like 90% sure we ditched the ability for that to be pinged
Unknown User•4y ago
Message Not Public
Sign In & Join Server To View