Fancy Button color change.
Hi all,
I am trying to change the color of a button background. It is initially set via a custom control using the <setter> tag as a template like so:
I am now using a function to change the background color yet it is not working. I am assuming the <setter> is overriding my color change.
This is the example code
This is the xaml reference
Could you give me some guidance as to why the background color isn't changing yet the foreground changes as you can see the red text.
Here is a link to the sample reference code. https://paste.mod.gg/ixhwhtdewdjs/2
Thank you
BlazeBin - ixhwhtdewdjs
A tool for sharing your source code with the world!

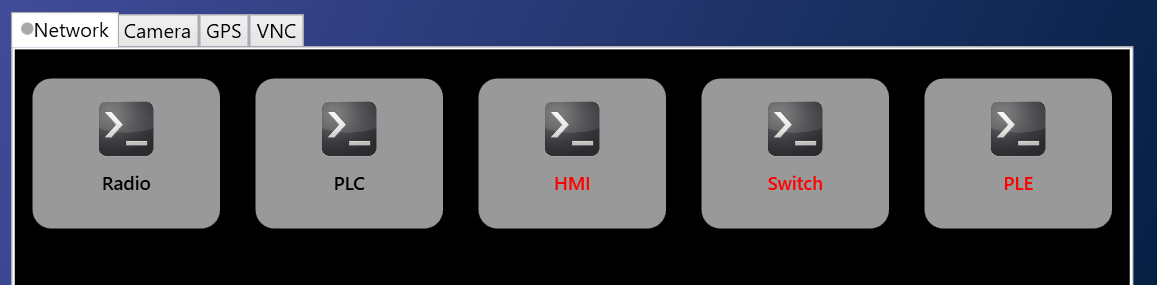
67 Replies
Let me know how this is:
with a viewmodel:
proof of concept that doesn't exactly work with what you have going on, which is having many buttons which shouldn't tie to many properties
I think people will look down on me referencing colors directly in the VM.
I will only elevate you mate, thank you for even taking a look
Here's your situation fleshed out
BlazeBin - uabmzbdugxbb
A tool for sharing your source code with the world!
Thank you I was trying to make your code work
I took out a lot of code related to this stuff:
AddingCalculatorViewModel addingCalculatorViewModel, WeekViewModel weekViewModel, DiffViewModel diffViewModel, ButtonTestViewModel buttonTestViewModel
If you see it, ignore it. I might have deleted more than I should. In which case you can ask.
I'll give you one more file that can help.No worries
Im just reading your code trying to make sense of it and adapt it to my own
and app.xaml:
So basically, the WPF starts up. It doesn't open a window directly
Instead, it runs
App()
because it's the entry point
App
then creates a bunch of services as singletons. Then it creates the ViewModel, then the View.
Then from the app.xaml, it seeds it needs to run Application_Startup
, which will grab the MainWindowView
's required services based on the mainwindowView constructor
which is here: Yup
then it shows the window. Great, we have mainWindow, but not the tab that matters.
So we inject a new
ButtonColorCollectionViewModel
with the help of the services
created in App()
It's set as a property
I put the stuff you care about in a TabControl
, and it's just one of the many tabs
but WPF doesn't know how to show a viewmodel directly. So I added some resources to the MainWindowView's Window's resources
which is more or less
"If I'm trying to show ButtonColorCollectionViewModel
", then show ButtonColorCollectionView
in its place, using that viewModel as the DataContext"
The constructor for the view is simple, and I only removed a bunch of boilerplate from it:
You don't see it, but the DataContext
has been automagically set behind the scenes for you
When ButtonColorCollectionViewModel
was instantiated, it ran the constructor, which creates 12 instances of ButtonItemViewModel
, which has a shitty default color.
ButtonItemViewModel
doesn't have a corresponding View
, because I was lazy. I just used a DataTemplate
. You could easily extract it into its own component.Forgive me for what I am about to say as I am reading your code and comparing the differences to that of my own
I'd recommend setting up a separate project to match my own, then play with it. Then you can slowly integrate it into your solution.
So it looks like I need to have an ObservableObject which then changes the property
I set up a bunch of test/example projects in my solution so I can just unload them later, but still use them as a reference sometimes.
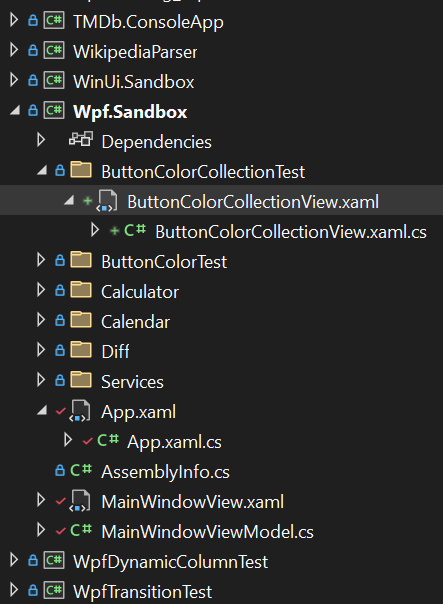
Yeah, that comes from MVVM Community Toolkit. I'm lazy to set up INotifyPropertyChanged myself.
thats ok 😄
having
ObservableObject
with partial
implements that with SetValue
(I think). [ObservableProperty]
creates a property in addition to the field I defined. Fields are bad.
There's a new fancy way of doing it in .NET 9, but I don't know it.I have also noticed that as you stated, I don't actually use the Button Backround but just a Border Background
Oh, is that the crux of the issue? 😄
I didn't really read your question tbh. I'm good at formulating questions, not reading them.
No no
That setter tag seems to change the entire background colour of the button
so if i manually change value to red for example
button goes red
Here's a fun one for you:
I think this might be a bit closer to what you envisioned since you're pinging IP addresses for your security cameras or whatever.
Eventually, you can change it from being a button to being an image and a button underneath called "UpdateFeedThumbnail" or something
or "OpenFeedDiagnostics"
Then you can open up a new window and see when's the last time you had a connection to it or get some other details about it. Maybe where you stored the video.
fuck i'd really love to be able to sit down with you and go over everything!!!!
i shouldn't been asleep already im on night shift and im awake because i don't get the chance to do this while im at work
I only really have one meaningful property created on the
ButtonItemViewModel: ```cs
[ObservableProperty] private string _buttonText = "Click me!";
```
but I imagine you're going to be storing an IP address in there or something, so you're not switching stuff based on
Random.Shared.NextDouble() < 0.5`Yeah
from the code I gave you, I think one bad practice I did was initiating the behavior of the viewModel before the tab is ever activated.
so when I open the application and switch to the tab after 5 seconds elapses, everything is already colorful.
Looking at your way of doing it, i'd have to actually rewrite my entire custom control for my button altogether
I should have added this to the ViewModel with the collections:
and then created a
[RelayCommand]
that'll get hit by that. That way you're not doing network stuff when you don't have to.
I think I could also pause the pinging when the tab isn't open with another event like "closed"that makes fantastic sense
Unloaded
I added this to the
UserContnrol
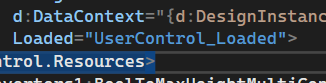
Out of curiousity in my version of the example. (This is for my clarification), does the RadioButton.Foreground only work because it hasn't been set by the style in the template
then F12 on
Loaded
and looked for other words, since F4/Properties
doesn't work for me
oh, for RadioButton?Where as the Radio.Button has been ?
Yeah let me show you what its doing one moment
I don't think I've ever styled one.
Keep an eye out for the 3 that change to red
Those have the .Foreground property being changed
the first two boxes have the .Background
Is that the desired behavior?
The desired behaviour is for the background to change
I was merely testing
Ahh, ok.
to show you that the code is actually doing something
just not actually invoking the changed background colour
yet it does foreground
which is odd ?
I think you should show the full code for one item. I'm not sure how it's set up.
or is everything just in a
Border
?Everything is in a border
did you not see my full code ?
BlazeBin - norykmlilyrt
A tool for sharing your source code with the world!
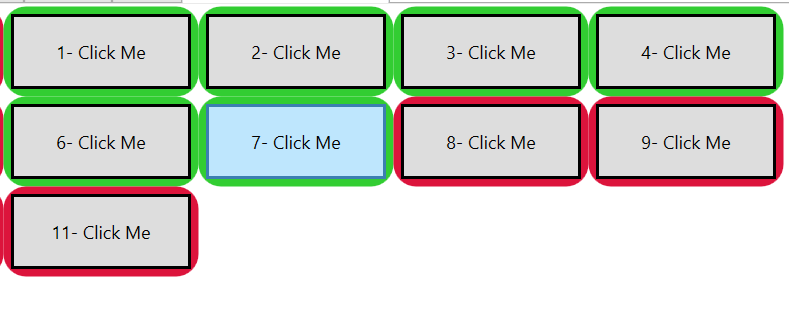
That has the example button which i made a template, the xaml for the use of the button and the code behind it
I saw the opening tags of the xaml, but not the ending
I think maybe you have a bunch of hard-coded xaml for each button you need, right?
Yes sir
I put all of mine in a collection, then present them in a
WrapPanel
, so I can do this: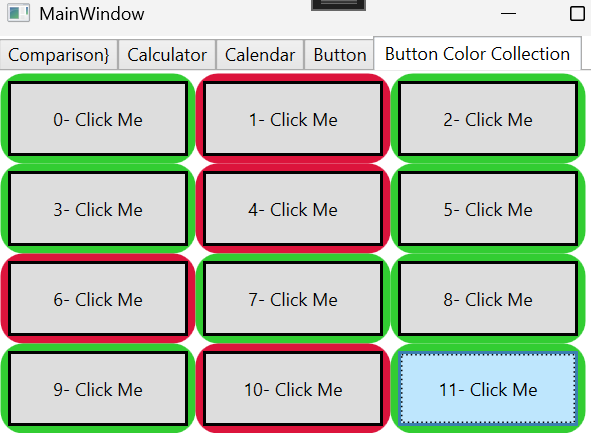
this was the complete button template
ok. I made a small change in my
ItemTemplate
This allows the border to be magenta'd when it's hovered over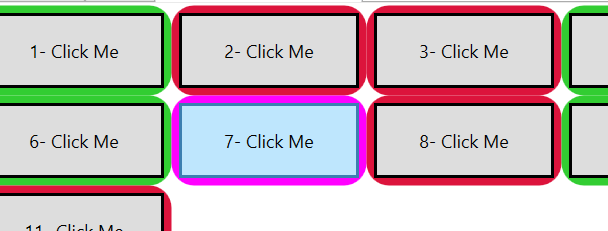
so I had to move where I was originally coloring the Border's background to be in a
Style
like you have it.i see
my trigger for the buttonBackground doesn't work in the xaml shown above
Doing that is too complicated for me.
thats ok
I'd end up doing something gnarly and shitty. It's more XAML than I care to maintain, so I'd push back on it.
You have shown me more than enough
I'm going to sleep now. Questions like this are better suited for #gui , but responses are slower.
The binding code is still the same i believe
I missed your question in #chat because someone posted a link after your question and I focused on that. I usually look at #gui whenever there's a new message and help with easy questions.
So your updated version where the setter value is being bound
ButtonBackground binding
still getting it from here
is that correct ?
yeah awesome