Silly OOP question about constructors
I am learning OOP and came across this. A way to reuse constructors:
I was wondering that can I just use default values for parameters like this, to achieve same result:
If yes then is their any use case when we would prefer the 1st way over 2nd?
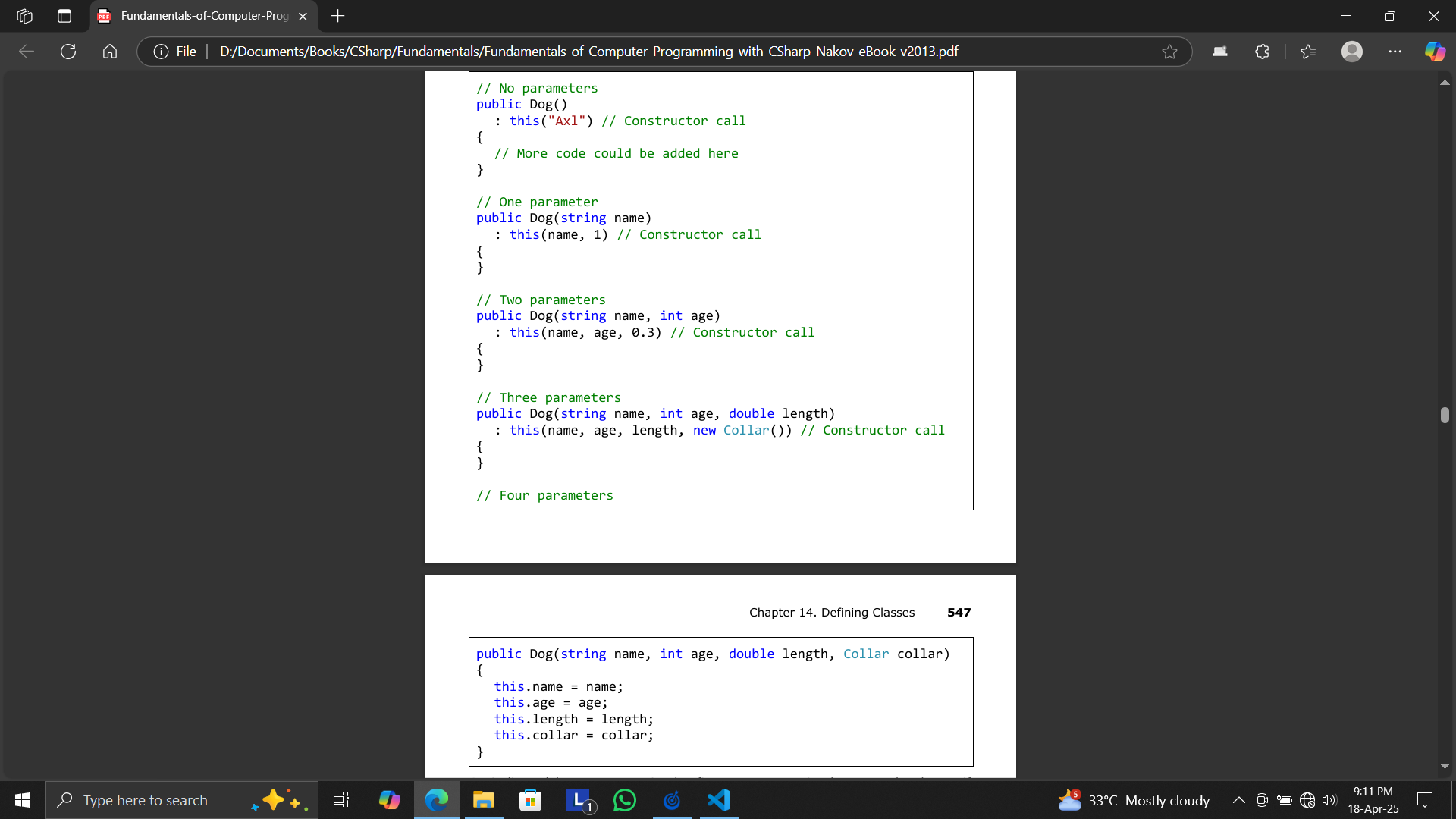
6 Replies
Optional arguments isn't exactly the same as method/constructor overloading, especially when reflected over
Unknown User•3d ago
Message Not Public
Sign In & Join Server To View
But if its a type only used internally or similar and that isn't a problem for you, yeah this works
Hmm... I will see
And yes this book is ancient 😅
Your not going to be able to do the last parameter,
Collar collar = new Collar()
because its not a compile time constant.
buuuut, you can do something like this:
public Dog() : this("NoName", 1, 0.3, new Collar()) { }
This will conflict with this constructor however:
public Dog() : this("NoName") { }
As that already defines a constructor with no parameters.Thanks, have to play around a bit to get used to it.