Extremely strange behavior
I'm not sure what to call this.
All addresses are stored in the address table. To distinguish mailing or physical addresses, their IDs are placed into the respective tables which are then reference by student.
When a student has equivalent mailing and physical addresses, the function above in my NestJS application returns
undefined
for the address_physical
within the Student object and I have no idea why. I suspect that because there are name similarities or the IDs to the mailing and physical addresses are the same causes Prisma to go haywire. The even weirder thing is that when I console.log the address_physical.address
, it is very clearly defined (although the same as mailing_address
, which is intended). I have checked my NestJS entity models and there are no issues there either.4 Replies
You're in no rush, so we'll let a dev step in. Enjoy your coffee, or drop into
#ask-ai
if you get antsy for a second opinion!typescript
* Get the student component of this user, if it exists
* @param userId The id of the user
* @returns The student component, or an empty object if no student component exists
*/
async getStudent(userId: number) {
const res = await this.prisma.student.findUniqueOrThrow({
where: {
user_id: userId,
},
include: {
address_mail: {
include: {
address: true,
}
},
address_physical: {
include: {
address: true,
},
},
coordinator: {
include: {
center: true,
}
},
district: true,
course: true,
}
})
const { address_mail, address_physical, coordinator, district, course, ...rest } = res
const stu = new Student({
address_mail: new Address(address_mail.address),
address_physical: new Address(address_physical.address),
coordinator: new Coordinator(coordinator),
district: district ? new District(district) : null,
course: new Course(course),
...rest,
})
console.log(stu)
console.log(address_mail)
console.log(address_physical)
console.log(address_physical == address_mail)
console.log(address_physical === address_mail)
return stu
}
typescript
* Get the student component of this user, if it exists
* @param userId The id of the user
* @returns The student component, or an empty object if no student component exists
*/
async getStudent(userId: number) {
const res = await this.prisma.student.findUniqueOrThrow({
where: {
user_id: userId,
},
include: {
address_mail: {
include: {
address: true,
}
},
address_physical: {
include: {
address: true,
},
},
coordinator: {
include: {
center: true,
}
},
district: true,
course: true,
}
})
const { address_mail, address_physical, coordinator, district, course, ...rest } = res
const stu = new Student({
address_mail: new Address(address_mail.address),
address_physical: new Address(address_physical.address),
coordinator: new Coordinator(coordinator),
district: district ? new District(district) : null,
course: new Course(course),
...rest,
})
console.log(stu)
console.log(address_mail)
console.log(address_physical)
console.log(address_physical == address_mail)
console.log(address_physical === address_mail)
return stu
}
model address {
id Int @id @default(autoincrement())
state String @db.Char(2)
zip_code String @db.VarChar(20)
city String @db.VarChar(100)
street String @db.VarChar(256)
street_alt String? @db.VarChar(256)
attn String? @db.VarChar(256)
address_mail address_mail?
address_physical address_physical?
}
model address_mail {
address_id Int @id
address address @relation(fields: [address_id], references: [id], onDelete: NoAction, onUpdate: NoAction, map: "address_mail_ibfk_1")
center. center[]
district district[]
student student[]
}
model address_physical {
address_id Int @id
address address @relation(fields: [address_id], references: [id], onDelete: NoAction, onUpdate: NoAction, map: "address_physical_ibfk_1")
center center[]
district district[]
student student[]
}
model address {
id Int @id @default(autoincrement())
state String @db.Char(2)
zip_code String @db.VarChar(20)
city String @db.VarChar(100)
street String @db.VarChar(256)
street_alt String? @db.VarChar(256)
attn String? @db.VarChar(256)
address_mail address_mail?
address_physical address_physical?
}
model address_mail {
address_id Int @id
address address @relation(fields: [address_id], references: [id], onDelete: NoAction, onUpdate: NoAction, map: "address_mail_ibfk_1")
center. center[]
district district[]
student student[]
}
model address_physical {
address_id Int @id
address address @relation(fields: [address_id], references: [id], onDelete: NoAction, onUpdate: NoAction, map: "address_physical_ibfk_1")
center center[]
district district[]
student student[]
}
model student {
id Int @id @default(autoincrement())
user_id Int @unique(map: "user_id")
coordinator_id Int
district_name String? @db.VarChar(100)
course_id Int
address_mail_id Int
address_physical_id Int
first_name String @db.VarChar(100)
last_name String @db.VarChar(100)
middle_name String @db.VarChar(100)
birthday DateTime @db.Date
grade_level String @db.VarChar(20)
gender String @db.VarChar(50)
user user @relation(fields: [user_id], references: [id], onDelete: NoAction, onUpdate: NoAction, map: "student_ibfk_1")
coordinator coordinator @relation(fields: [coordinator_id], references: [id], onDelete: NoAction, onUpdate: NoAction, map: "student_ibfk_2")
district district? @relation(fields: [district_name], references: [name], onDelete: NoAction, onUpdate: NoAction, map: "student_ibfk_3")
course course @relation(fields: [course_id], references: [id], onDelete: NoAction, onUpdate: NoAction, map: "student_ibfk_4")
address_mail address_mail @relation(fields: [address_mail_id], references: [address_id], onDelete: NoAction, onUpdate: NoAction, map: "student_ibfk_5")
address_physical
}
model student {
id Int @id @default(autoincrement())
user_id Int @unique(map: "user_id")
coordinator_id Int
district_name String? @db.VarChar(100)
course_id Int
address_mail_id Int
address_physical_id Int
first_name String @db.VarChar(100)
last_name String @db.VarChar(100)
middle_name String @db.VarChar(100)
birthday DateTime @db.Date
grade_level String @db.VarChar(20)
gender String @db.VarChar(50)
user user @relation(fields: [user_id], references: [id], onDelete: NoAction, onUpdate: NoAction, map: "student_ibfk_1")
coordinator coordinator @relation(fields: [coordinator_id], references: [id], onDelete: NoAction, onUpdate: NoAction, map: "student_ibfk_2")
district district? @relation(fields: [district_name], references: [name], onDelete: NoAction, onUpdate: NoAction, map: "student_ibfk_3")
course course @relation(fields: [course_id], references: [id], onDelete: NoAction, onUpdate: NoAction, map: "student_ibfk_4")
address_mail address_mail @relation(fields: [address_mail_id], references: [address_id], onDelete: NoAction, onUpdate: NoAction, map: "student_ibfk_5")
address_physical
}
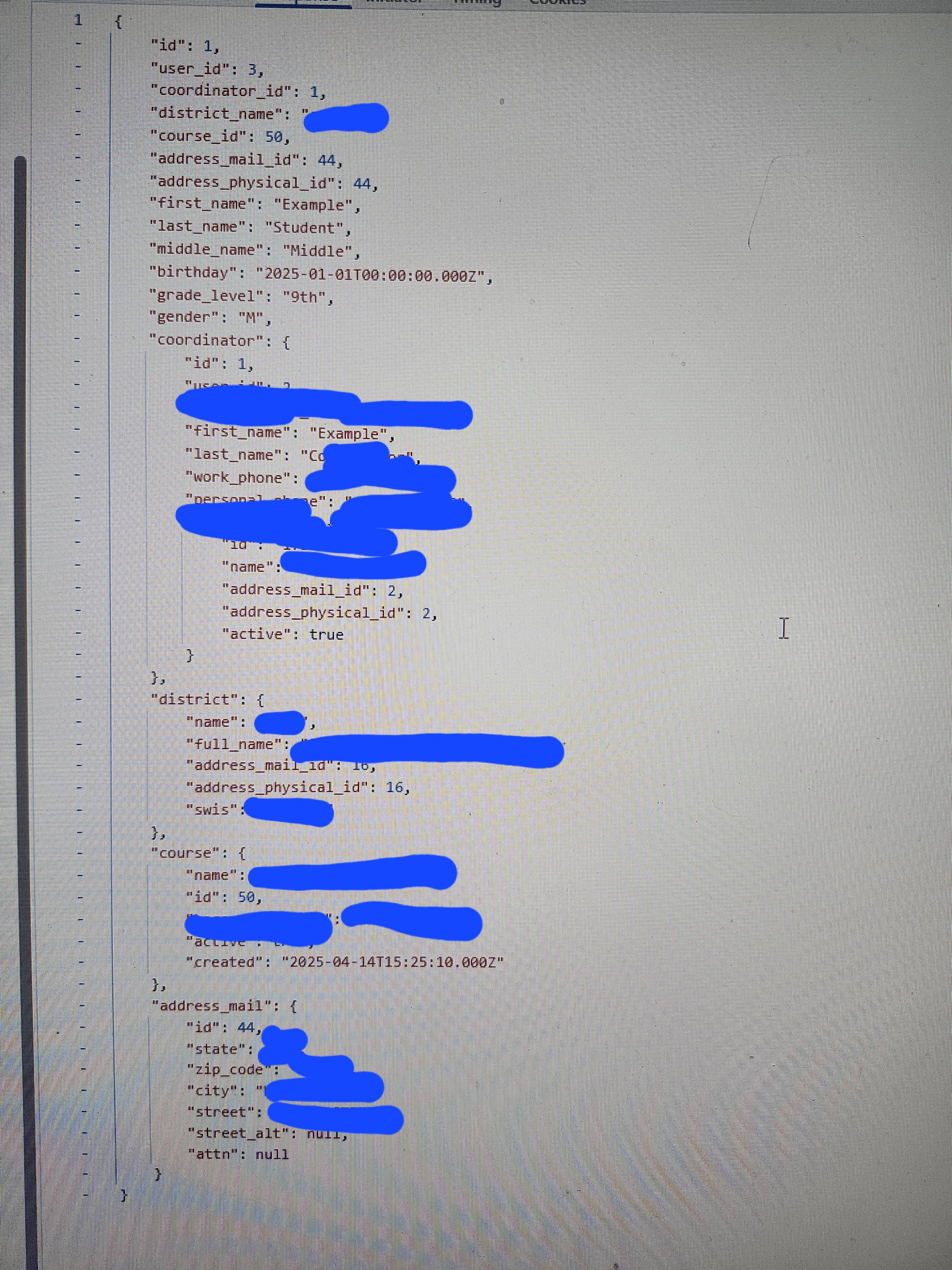
Student entity:
Even when the address_mail_id and address_physical_id are different, address_physical refuses to be returned in the API within the Student object.
Nevermind, solved it. If there's one thing I hate the most it's cache invalidation.
import { Coordinator } from './coordinator.entity';
import { User } from './user.entity';
import { District } from './district.entity';
import { Course } from './course.entity';
import { Address } from './address.entity';
export class Student {
/** Student ID */
id: number;
/** This student's user component */
user_id: number;
/** The coordinator who monitors this student */
coordinator_id: number;
/** The district this student is associated with */
district_name: string | null;
/** The course this student is taking */
course_id: number;
/** Mailing address id */
address_mail_id: number;
/** Physical address id */
address_physical_id: number;
/** First name */
first_name: string;
/** Last name */
last_name: string;
/** Middle name */
middle_name: string;
/** Birthday */
birthday: Date;
/** The grade level of this student. This is usually 'adult', '9th', '10th', '11th', '12th' */
grade_level: string;
/** Gender of the student */
gender: string;
/** User component */
user: User
/** Coordinator component */
coordinator: Coordinator
/** District of this student. A student can possibly be not part of a district especially if they are an 'adult'. */
district: District | null
/** Course of this student */
course: Course
/** Mailing address of this student */
address_mail: Address
/** Physical address of this student */
address_physical: Address
constructor({user, coordinator, district, course, address_mail, address_physical, ...data}: Partial<Student>) {
Object.assign(this, data)
if (user) {
this.user = user
}
if (coordinator) {
this.coordinator = coordinator
}
if (district) {
this.district = district
}
if (course) {
this.course = course
}
if (address_mail) {
this.address_mail = address_mail
}
if (address_physical) {
this.address_physical = address_physical
}
}
}
import { Coordinator } from './coordinator.entity';
import { User } from './user.entity';
import { District } from './district.entity';
import { Course } from './course.entity';
import { Address } from './address.entity';
export class Student {
/** Student ID */
id: number;
/** This student's user component */
user_id: number;
/** The coordinator who monitors this student */
coordinator_id: number;
/** The district this student is associated with */
district_name: string | null;
/** The course this student is taking */
course_id: number;
/** Mailing address id */
address_mail_id: number;
/** Physical address id */
address_physical_id: number;
/** First name */
first_name: string;
/** Last name */
last_name: string;
/** Middle name */
middle_name: string;
/** Birthday */
birthday: Date;
/** The grade level of this student. This is usually 'adult', '9th', '10th', '11th', '12th' */
grade_level: string;
/** Gender of the student */
gender: string;
/** User component */
user: User
/** Coordinator component */
coordinator: Coordinator
/** District of this student. A student can possibly be not part of a district especially if they are an 'adult'. */
district: District | null
/** Course of this student */
course: Course
/** Mailing address of this student */
address_mail: Address
/** Physical address of this student */
address_physical: Address
constructor({user, coordinator, district, course, address_mail, address_physical, ...data}: Partial<Student>) {
Object.assign(this, data)
if (user) {
this.user = user
}
if (coordinator) {
this.coordinator = coordinator
}
if (district) {
this.district = district
}
if (course) {
this.course = course
}
if (address_mail) {
this.address_mail = address_mail
}
if (address_physical) {
this.address_physical = address_physical
}
}
}