β StreamReader returns empty string on valid file stream
I have this code:
After this,
str
is empty. The stream, however, is valid. The Length
property matches the length of the file, it was opened with the Read
permission and the CanRead
property is true
. The position was at 0
, and has not been advanced and is still at 0
after the read. The attached screenshot shows the stream
(and str
) variables after the ReadToEnd
call.
To make sure the stream is valid, I added this after the ReadToEnd
:
And this correctly writes the full file, in all its glory, to the provided location, which should confirm that the issue is not with the stream itself, and all file permissions of the stream have been set correctly.
I'm using .NET Framework 4.8.
What could be the issue here?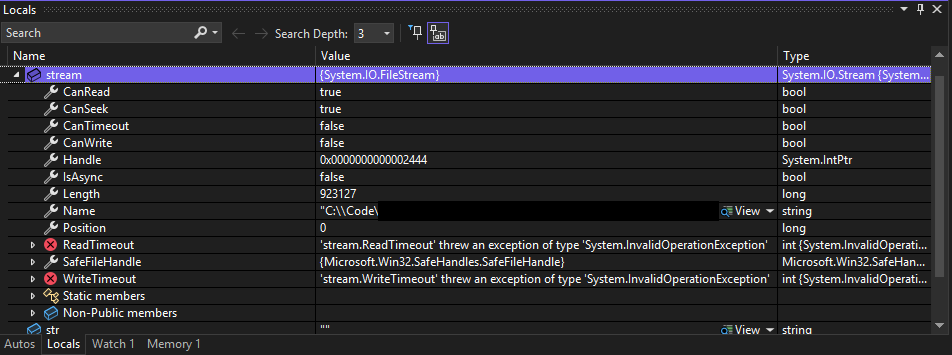
28 Replies
Oh, shit, almost forgot to mention the funnest part
It works sometimes on the exact same file x.x
But the screenshot is still from a failed attempt (I added a breakpoint with the
str == ""
condition)
So even in the cases where it fails, it has a valid stream, that can successfully write to a file, but the StreamReader
still fails to use it correctly...... Huh. What does
File.ReadAllText
do?
(That should do exactly the same internally, which means it's a good way to figure out whether the problem is with the file itself (or maybe the SR), or whether it's something to do with your FileStream
)the entire code snippet might be good to look over just for sanity i guess
Hm, will need to see how to best check that π
At the point I'm at, I only have the stream available, but give me a few
I'll see if I can trim it down properly, this code is a bit involved and the stream is created in a base class, then used several classes down in the inheritance hierarchy
owch
Ugh, I think I have it, though it's hard to reliably reproduce it... @canton7 I think that
File.ReadAllText
throws an exception, where reader.ReadToEnd
just returns an empty string... and CopyTo
also does not seem to complain, despite not writing anything. I stated in the opening post, that the file gets successfully written, but that was a derp on my part <_< The file reading process was just started multiple times, and the second or third or w/e time it worked, and overwrite the 0B file the failed attempt created with the proper file...
I'm still not sure why neither File.Open
nor reader.ReadToEnd
nor stream.CopyTo
throw an exception though :\
File.ReadAllText
did throw...Read the source for ReadAllText, it literally just does what you're doing
Unfortunately I can't guarantee that the case where
File.ReadAllText
throws is the same as where reader.ReadToEnd
fails...What's the exception?
IOException, I can get you the error message in a sec
One difference is that it uses a different
Stream
constructorYeah, which might help narrow down the cause
I'll see if modifying the
Stream
constructor would help get that exception later onHmm, evidence is starting to point in the same direction here
Ok, I figured it out, and in retrospect, it's kinda obvious... though I still dislike that behavior by the reader
I opened the stream with
ReadWrite
sharing permissions
And another program was writing
Which the stream accepted
And the reader then just produced an empty output
Still not sure how I feel about that behavior by the reader π
But explains it, and easy to change
Read
is the correct share permission here anyway, no idea why it was ReadWrite
Thanks for the help, as usual π«‘Glad you got to the bottom of it! I wouldn't have expected that behaviour either, but then I haven't played with ReadWrite much
Same, but good to know
you need to close
ur stream
if
exists in the same place as
then u need to close that stream properly
Err that's obviously not the issue
using var just closes the stream IIRC at the END of the whole method
The process cannot access the file 'C:\Code...' because it is being used by another process.
Read the backlog
The stream needs to remain open in my case, it's being closed by our file reading harness outside of that method
But my issue was why it didn't throw an exception, along with some observational failures on my part
But it's all good now π
why?
why must it remain open?
just curious
Because it's being closed later
Why can't u read, offload the bytes and close
This is a complicated file reading harness that handles a bunch of issues
The inner method (which is where the above code happened) does not own the stream
It only accesses it partially
Read only stream π
It's solved now
My guess is that the stream is at the end of data already. Are you sure that whatever the source of the stream is has the Position at the front?
If not, ReadToEnd is doing the right thingβ¦ you are already at βthe endβ. There are zero characters left to read.
And that the reason that itβs unreliable is because you have two things messing with the same stream, which is a big no-no.
It isn't. See the screenshots