Expo and hono.js
In my hono.js
after logging in, the client sends the endpoint to the backend.
In the backend what should I do next for this?
And after logging in, the user table got updated but the useSession returns null.
61 Replies
Are you trynna use Google oauth ?
Yes, I am
From my expo app
And it made the user in the table but
not sure what to do next
in the backend
And why the session is null in the client even though it's logged in?
The doc isn't completely saying what to do š¦
This is when trying to login
GitHub
GitHub - LovelessCodes/hono-better-auth: š Hono x Better Auth: S...
š Hono x Better Auth: Seamlessly integrate powerful authentication with Hono, Better-Auth and Drizzle ORM. Secure, scalable, and built for modern web applications. š”ļøāØ - LovelessCodes/hono-better-auth
Okay, thanks. I will let you know. I hope it works š¦
in hono.js
and in expo
It still returns null for session in the client..
when using this
It all makes thses tho
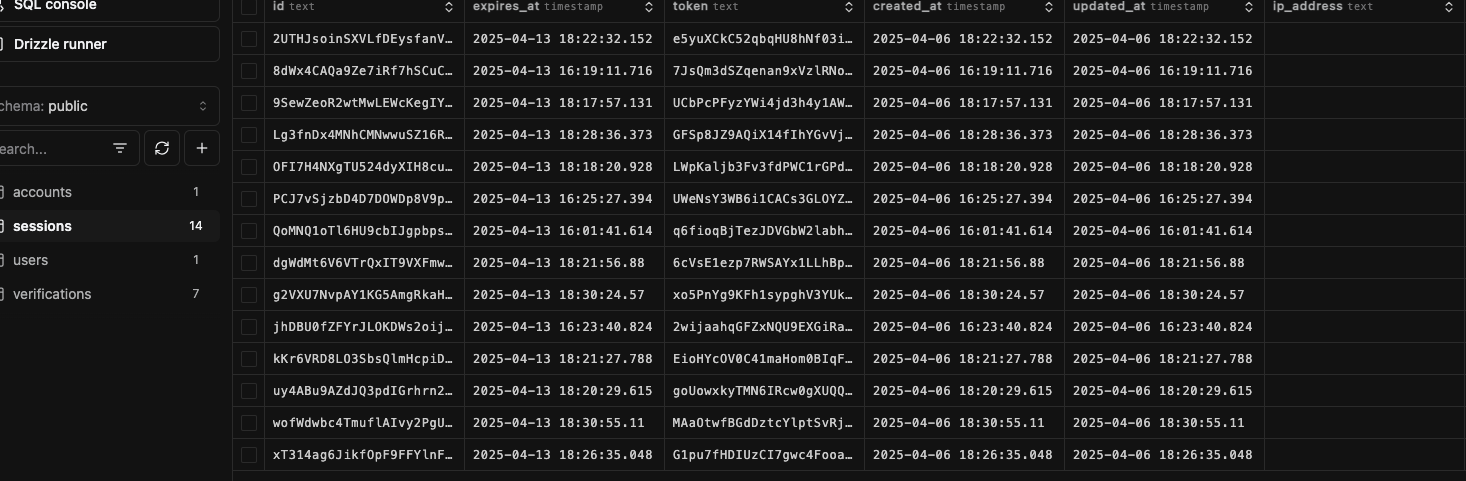
Do I need these two endpoints?
Can you do manually fetching for session endpoint itself
No you don't actually need the endpoints
Could you tell me how to do that?
I'm not sure
You mean like this?
Wait I'm in the middle of sth one minute
ok
I dropped the tables in DB but now it doesn't insert a user
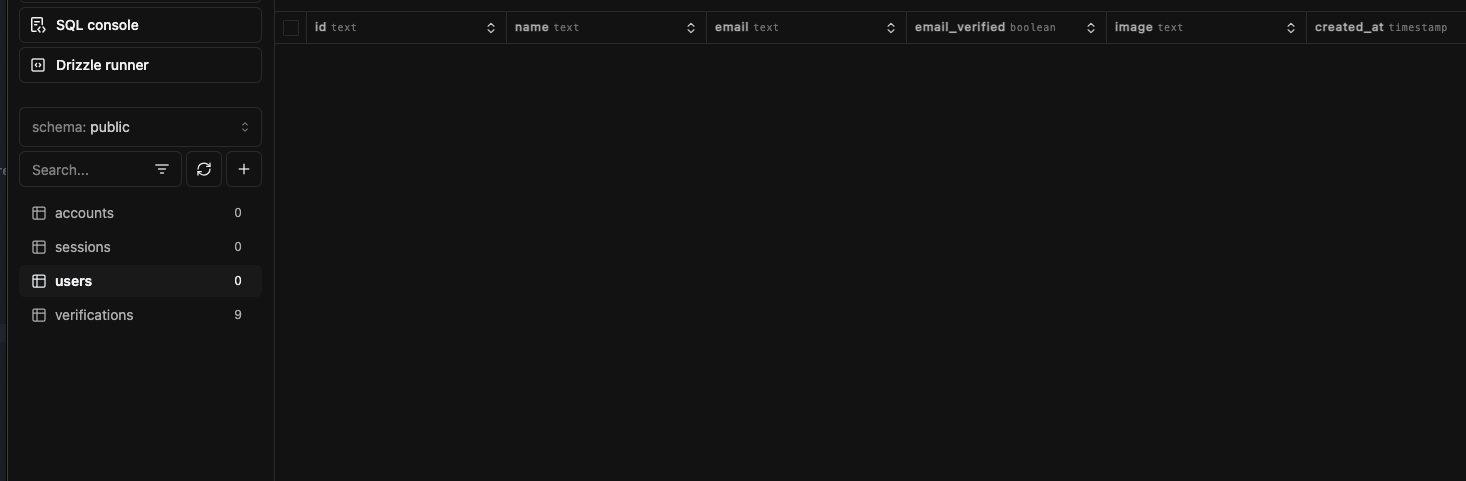
But without sign-in/social endpoint it returns 404

in the client
That's why I added that endpoint
you have not tweaked your hono backend like /api/auth ? does that apply to your callbacks ?
and make sure to add expo plugin on your backend hono as well
and expoClient on your app
They're added
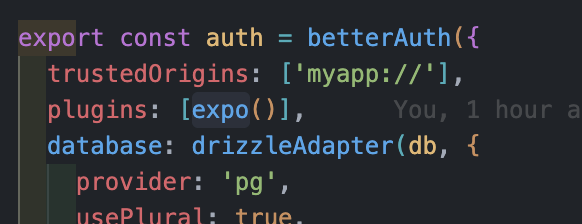
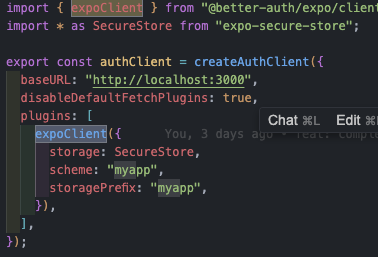
already
What do you mean by "you have not tweaked your hono backend like /api/auth-provider ? "?
i mean you should not have to handle the callback by yourself which you are defining
also and /auth is meant to handle all your callbacks
so make sure to adjust it to your need like api/auth ? or sth
So after remove the /auth-provider endpoint
it doesn't return 404
but after clicking signIn.socical function it doesn't do anything
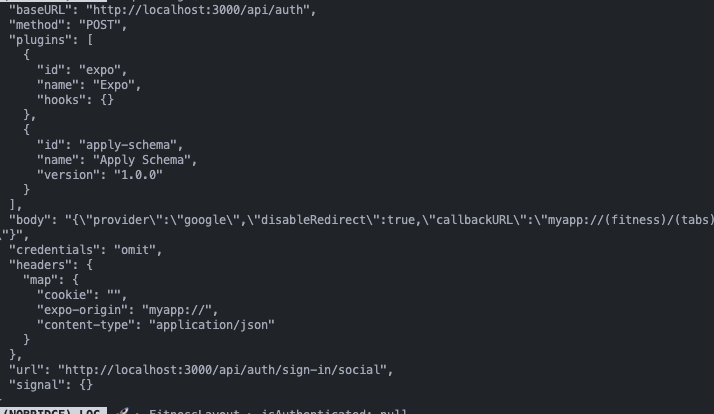
It just does this request
It doesn't go to the Google sign-in page
what about the backend endpoint ? the port it is running and make sure to have that while creating an auth client on expo
Ah it returns this data

So I sholud login with this link?
I got this in the client from server
how are you calling it on the client ?
Wit that URL I was able to create a user
Like this
so what does the data looks like
like this
I can get url and redirect
nice soo can you inspect the db
for user ?
and session
The user

The session

But when using useSessions in the client, it returns null
now we have hooked up with the backend
š struggling
Yeah, but why it doesn't return session?
so how are you trynna get the session ?
the snippet
In the client
The bakcend is the same
It hit the endpoint
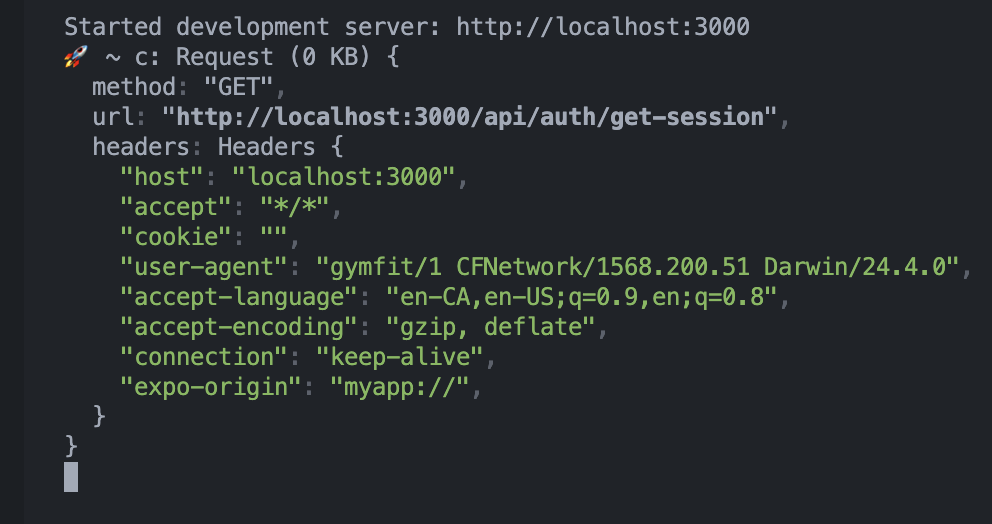
in the backend
can u able to get the cookies -
const cookies = authClient.getCookie();
with thisNo, I can't unfortunately
Cookies: nothing
do you have securestorage with your client config -
expoClient({
scheme: "myapp",
storagePrefix: "myapp",
storage: SecureStore,
})
Do you mean this one?
Yes, it's set
what version of better auth are you using ?
"better-auth": "^1.2.6-beta.4",
"@better-auth/expo": "^1.2.6-beta.4",
1.2.6-beta.4
can u confirm that it is hiting the backend with /get-session endpoint when u use the useSession hook
Confirmed
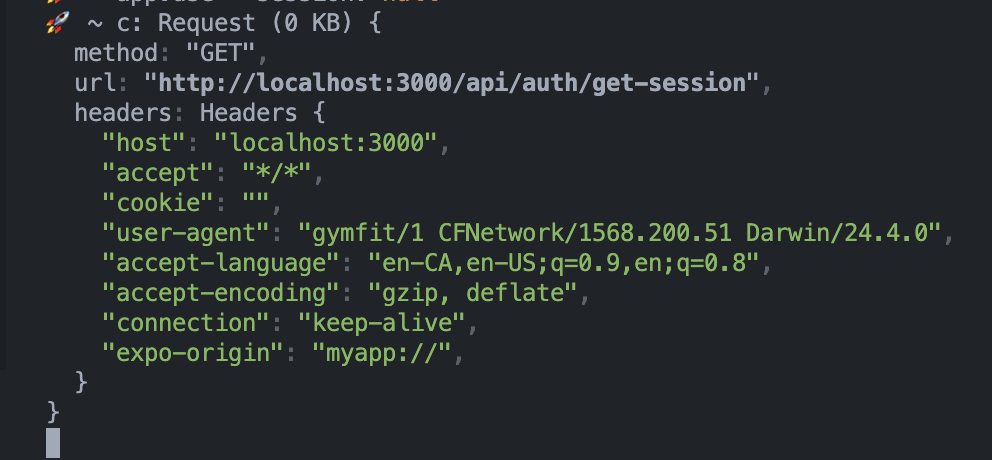
It's hitting the /get-sessions endpoint
check your backend log as well
It's from the backend
this endpoint is not actually a custom one ryt ?
It's not the frontend is hitting the get-session when using useSession
I'm checking the log here
yeah yeah i mean the you have not made custom endpoint for getting session with that api route ?
No I haven't made any endpoint for getting it
this is strange
Would this one
do something
it in the doc
it will make it tied with the context on backend not actually to expo client
ok
method: "GET",
url: "http://localhost:3000/api/auth/callback/google?state=RwWTDprNonWUPi3oGtE3n4G7T-7jo5AM&code=4%2F0Ab_5qlnLv4NRU3wENSEJ3UEdQjlQghjVdn7zYssnwvYL0k01xW0Iydy1PHisK3sB-qzkZQ&scope=email+profile+https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.email+https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.profile+openid&authuser=0&prompt=none",
headers: Headers {
"host": "localhost:3000",
"accept": "text/html,application/xhtml+xml,application/xml;q=0.9,/;q=0.8",
"sec-fetch-mode": "navigate",
"user-agent": "Mozilla/5.0 (iPhone; CPU iPhone OS 18_1 like Mac OS X) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/18.1 Mobile/15E148 Safari/604.1",
"accept-language": "en-CA,en-US;q=0.9,en;q=0.8",
"sec-fetch-dest": "document",
"accept-encoding": "gzip, deflate",
"cookie": "better-auth.session_token=FhCbI3ebCrNdvvd9oXVzmUfdChg7KjMl.1lWf7Jt%2F1FQ0yx8kvsq6dnfgNsF6vYcSW%2BtK8%2FFcmd0%3D",
"connection": "keep-alive",
"sec-fetch-site": "none",
"priority": "u=0, i",
}
}
when logging in
I can see the cookie
so did you run the hook ? useSession ?
Thank you very much. Appreciate that. haha
I'm closing one
no worries glad it worked, feel free to optimize the docs from what we experience
Okay, will do. Thanks!!
š„