What is the proper way to use discord.js with proxy server?
I want to run my Discord bot using a different IP address for both HTTP (REST API) and WebSocket (WSS) connections — so that all outbound traffic from the bot is routed through a specific proxy.
So far I have such simple configuration:
But it gives me timeout error (or doesn't output anything):
node version:
v22.11.0
discord.js: ^14.18.0
,
My proxy has http(s) type. It is indeed working. I have successfully requested main discord page through my proxy with https.5 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!Hi! I'm not very experienced yet, but I suspect the bot needs to use WebSocket to function fully. In that case - yes. I need to proxify webscoket connection as well.
As far as I know, if HTTPS is supported, then WSS should be supported as well (depends on proxy provider, but most of the times works that way). I even wrote a small code snippet to verify that WebSocket works correctly with my proxy:
Success was the result.
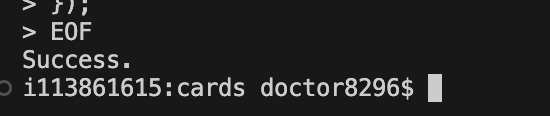
the WSS connection may not be routed correctly through the proxy using undici bec undici handles HTTP traffic not WebSockets i think, the handshake timeout error indicates that the websocket connection to discord is being blocked
but also consider these:
- timeouts
- prooxy config => make sure that ur proxy supports websocket tunneling, most HTTPS proxies do but there could be cases where some configs / restrictions prevent websocket connections
Correct me if I'm wrong
I use proxychains for this. just run the bot with this:
proxychains4 -q -f /path/to/proxychains4/config.conf node index.js
.
all requests coming out of this process including websocket will automatically use the configured proxy server
sample from one of my proxychain configurations: