✅ Creating a single global object that can be accessed by other classes?
I'm someone who comes from using Pascal and Lua, so bear with me here.
I want to create a single nested list with custom properties, which I can then iterate through. But it's not intuitive how we actually do that. To demonstrate, here is a snippet of the code I'm working on for Bannerlord:
I only need one instance of
tsClassroom
, and I'll handle clearing it out and refilling it. But a single instance would be a public static
, which can't have objects assigned to it right?
public static List<tsClassroom> = new List<tsClassroom>
is not supported in C#, so I'm a bit lost, even if it's obvious to others 🐤23 Replies
i'm not sure why you say
public static List<tsClassroom> = new List<tsClassroom>
is not supported. that's perfectly valid c#.
do you have some error message?Yeah it's a weird one. Like it just breaks C#
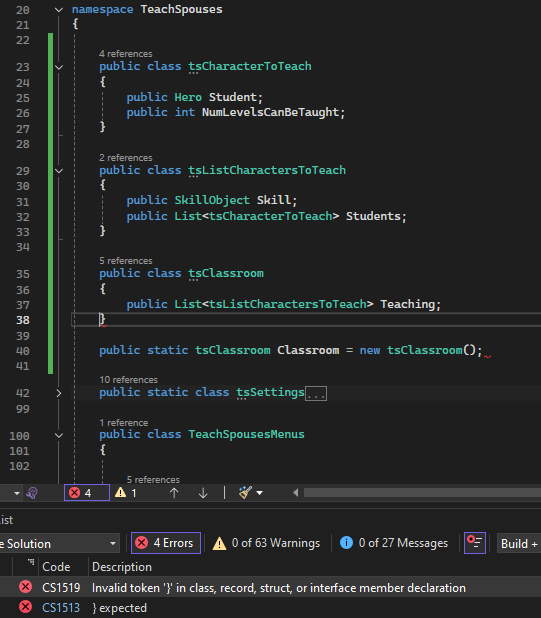
And without the brackets, it says there needs to be brackets.
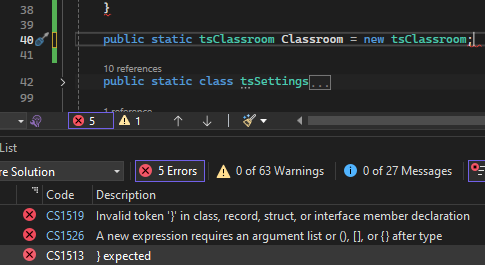
well you need to declare members in types
there's no such thing as a top level, or "global" member
Yeah that lost me already. I know we can assign basic variables like ints and floats, and access them easily by just doing e.g:
int iBaseCost = tsSettings.tsBase
Hang on maybe I'm on the verge of figuring this outAha! So it's like this? Got to put it in a static class
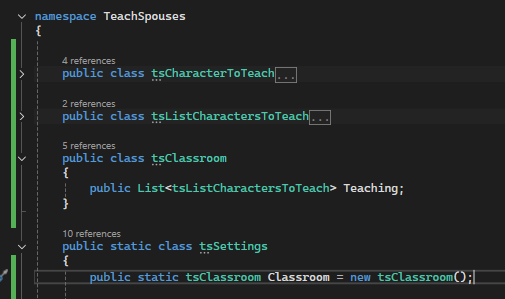
for example, yes
static members can be in non-static classes as well
for example
(we don't use hungarian notation in c#)
Banging. And I take it we need to create the objects inside it too? So later do
tsSettings.Classroom.Teaching = new List<tsListCharactersToTeach>
you can use an object initializer
Yeah I noticed the different syntax. For me, I get lost and distracted very easily. So having the variable type at the start of the name helps keep me steady
c# is statically typed, every variable already has a specific type
it's not like in lua where you genuinely need the name to keep track of the type
Aye I meant more for me typing out code. Pascal is the same
Easily over half of my issues are me mixing up types because I got careless or distracted
you can't mix up types in c# is what i'm saying
and even while typing you can view the type of any variable you use

just saying in case you plan on creating bigger projects with contributors
they'll likely be very confused about the hungarian notation choice
not that it matters while you're just learning
or
to be more explicit
you can also just make
tsClassroom
static
itselfYou are a wizard god damn. This is magic
but it's sort of bad practice to make everything static
Oh aye I've not made everything static. Just the stuff I only need once. The game I'm modding is filled with non-static objects, and I'm doing stuff to them
you wouldn't really need a static
Classroom
, imo
if you need one, you'd instantiate it in Program.Main
or something
and pass it around as neededYeah there needs to be one, but just one. Passing things around is a mess, because of how events work and other weird stuff. There will be proper ways to go about it
but I'm a baby bird with C# atm
it's not "wrong" to use a singleton pattern for this, but it's more "oop-like" not to use it and instead pass around instances
whichever works for you for now, just be aware
$close
If you have no further questions, please use /close to mark the forum thread as answered
I owe you my life