Discord HTTP Interactions Issue
I'm having this weird issue trying to make a bot with CF workers where my worker hangs on the fetch in this block:
The fetch request reaches Discord (it dispatches the defer that I expect it to) but nothing else happens.
Just to add on, I proxied it through my local machine using ngrok, and it worked just fine. It seems like requests coming directly from Discord are causing some sort of issue, but proxying them through my own machine resolves that somehow.
Any ideas on what could be happening?
Some additional code:
https://github.com/SkyKings-Guild/GameNightManager
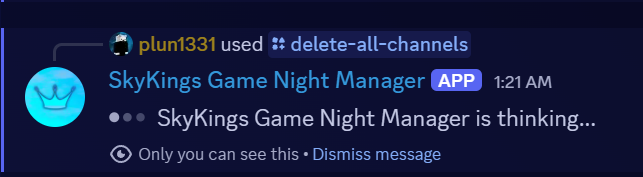
1 Reply
I put a log before/after the
fetch
but the only thing that logs is the one before
I respond over HTTP though, since that behavior is allowed
& again, it works fine if i route discord's requests through my machine to my worker, but not if discord directly requests my worker
so perhaps there's a weird gimmic in there somewhere that i'm not seeing